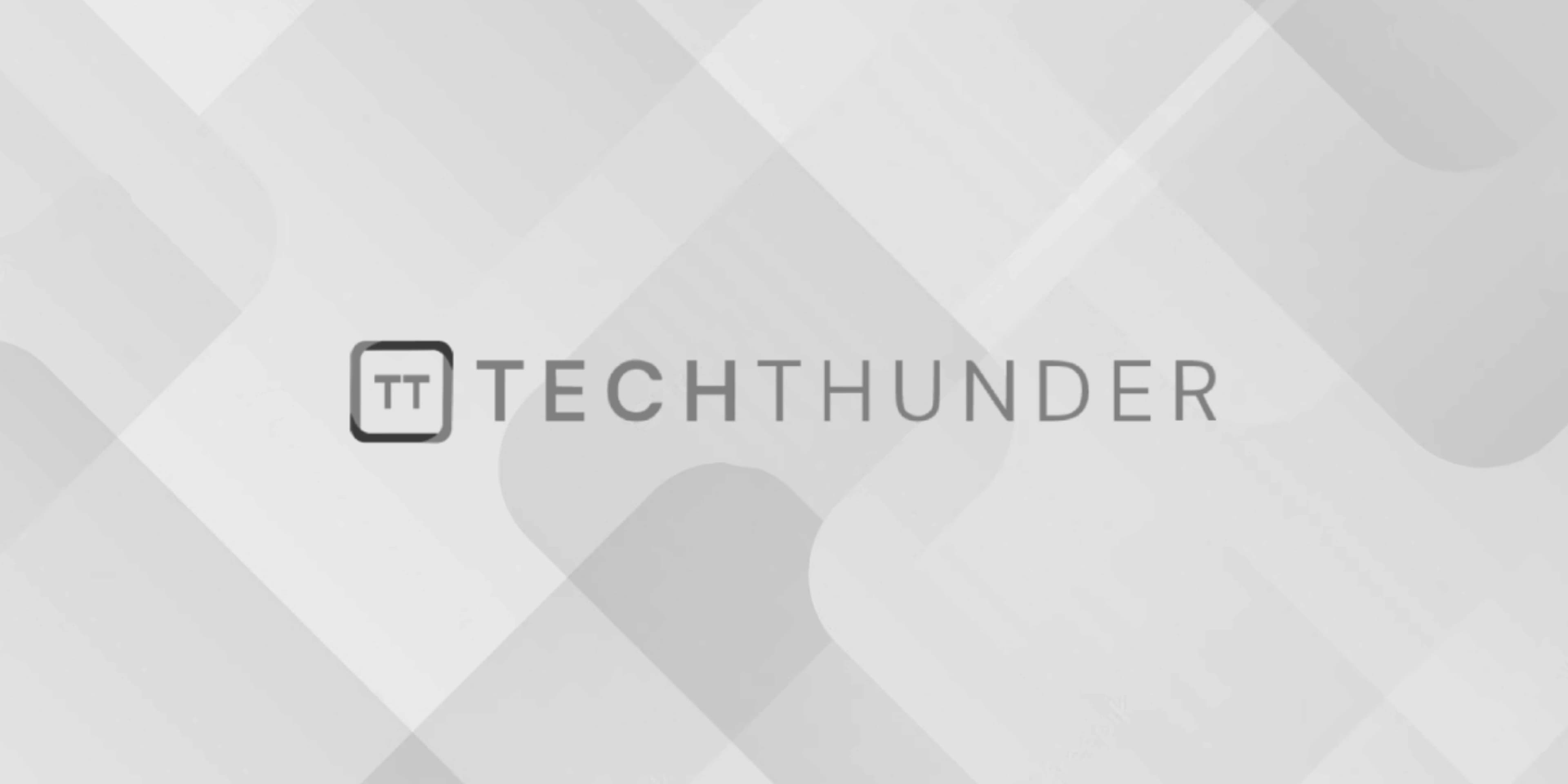
Popup Menu in Android
The Android PopupMenu is a user interface component that displays a list of items or options in a pop-up dialog anchored to a specific view. PopupMenus are typically used to provide a list of actions or choices when a user interacts with a UI element, such as a button or an ImageView. They are a convenient way to offer additional functionality or options without cluttering the main screen.
Here’s how you can create and use a PopupMenu in Android:
Create a PopupMenu:
// Initialize a new PopupMenu with the context and the anchor view
PopupMenu popupMenu = new PopupMenu(this, anchorView);
this
refers to the context of your activity or fragment.anchorView
is the view to which the PopupMenu will be anchored.
Inflate a Menu Resource:
// Inflate a menu resource into the PopupMenu
popupMenu.getMenuInflater().inflate(R.menu.popup_menu, popupMenu.getMenu());
R.menu.popup_menu
is the XML resource file defining the menu items for the PopupMenu. You should create this resource file in theres/menu
directory.
Set Click Listeners: You can set click listeners for the items in the PopupMenu to handle user interactions:
popupMenu.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
switch (item.getItemId()) {
case R.id.menu_item_1:
// Handle menu item 1 click
return true;
case R.id.menu_item_2:
// Handle menu item 2 click
return true;
default:
return false;
}
}
});
Show the PopupMenu:
// Show the PopupMenu
popupMenu.show();
- This will display the PopupMenu anchored to the specified view (e.g., a button or an ImageView), and the user can select an item from the menu.
- (Optional) Handle Dismiss Event: You can also set an OnDismissListener to handle actions when the PopupMenu is dismissed:
popupMenu.setOnDismissListener(new PopupMenu.OnDismissListener() {
@Override
public void onDismiss(PopupMenu menu) {
// Handle PopupMenu dismissal
}
});
Here’s an example XML menu resource (res/menu/popup_menu.xml
) that defines two menu items:
<!-- res/menu/popup_menu.xml -->
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/menu_item_1"
android:title="Option 1" />
<item
android:id="@+id/menu_item_2"
android:title="Option 2" />
</menu>
This example creates a PopupMenu with two menu items (“Option 1” and “Option 2”). When an item is clicked, the corresponding onMenuItemClick()
method is called, allowing you to handle the user’s selection.
PopupMenus are a useful way to provide context-specific options or actions in your Android app’s user interface.