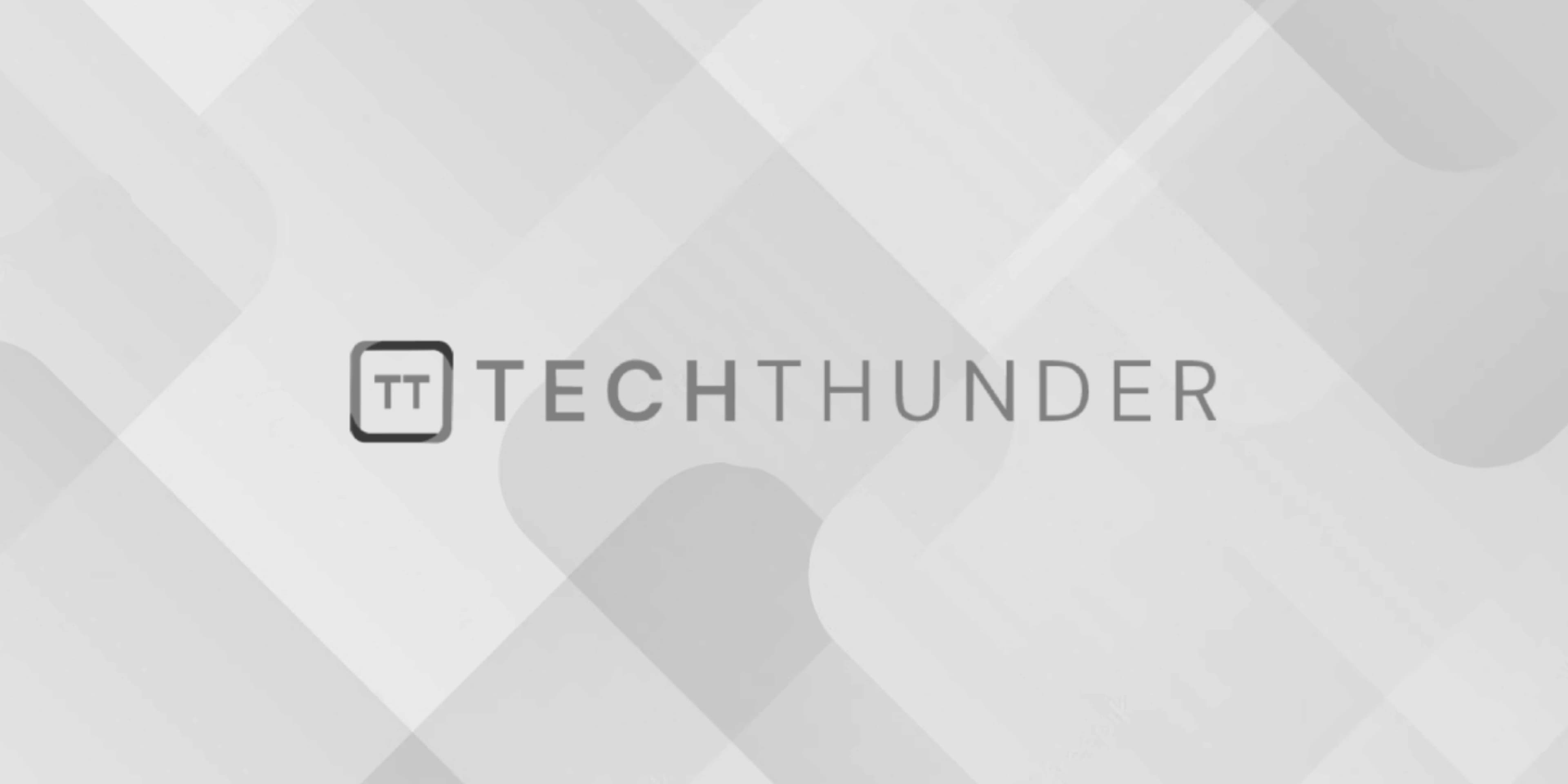
VideoView: Video in Android
The VideoView
widget in Android is a simple way to play video content within your app. It provides a built-in UI for video playback, making it easy to integrate video into your Android application. Here’s how you can use VideoView
to play videos:
1. Add VideoView
to Your Layout:
In your XML layout file, add a VideoView
element where you want to display the video. For example:
<VideoView
android:id="@+id/videoView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
2. Set Up VideoView
in Your Activity or Fragment:
In your activity or fragment, you’ll need to reference the VideoView
and set it up to play a video. Here’s a basic example:
import android.net.Uri;
import android.os.Bundle;
import android.widget.MediaController;
import android.widget.VideoView;
import androidx.appcompat.app.AppCompatActivity;
public class VideoPlayerActivity extends AppCompatActivity {
private VideoView videoView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_video_player);
// Find the VideoView by its ID
videoView = findViewById(R.id.videoView);
// Specify the video file's URI (either a local file or a remote URL)
Uri videoUri = Uri.parse("android.resource://" + getPackageName() + "/" + R.raw.sample_video);
// Set the video URI to the VideoView
videoView.setVideoURI(videoUri);
// Create media controller for playback controls (optional)
MediaController mediaController = new MediaController(this);
mediaController.setAnchorView(videoView);
videoView.setMediaController(mediaController);
// Start playing the video
videoView.start();
}
}
In this example:
- We find the
VideoView
by its ID. - We specify the video’s URI using
Uri.parse()
. In this case, we load a video from the app’s resources usingandroid.resource://
. - Optionally, we create a
MediaController
for playback controls and attach it to theVideoView
. - Finally, we start playing the video using
videoView.start()
.
3. Specify Video Sources:
You can specify different sources for the video, such as a local file, a remote URL, or a resource in your app’s res/raw
folder. Just ensure that you provide the correct URI to the setVideoURI
method.
4. Handle Video Events:
VideoView
provides event listeners to handle events such as completion or errors. You can set an OnCompletionListener
or an OnErrorListener
to handle these events.
videoView.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mp) {
// Handle video completion event
}
});
videoView.setOnErrorListener(new MediaPlayer.OnErrorListener() {
@Override
public boolean onError(MediaPlayer mp, int what, int extra) {
// Handle video error event
return false;
}
});
5. Permissions:
If you’re playing videos from the internet, don’t forget to add the appropriate internet permissions to your AndroidManifest.xml
file:
<uses-permission android:name="android.permission.INTERNET" />
6. Testing:
Test your video playback on various devices and Android versions to ensure compatibility and a smooth user experience.
VideoView
is a straightforward way to add video playback to your Android app. If you require more advanced features, such as custom video controls or additional interactivity, you may consider using the ExoPlayer
library or building a custom video player interface.