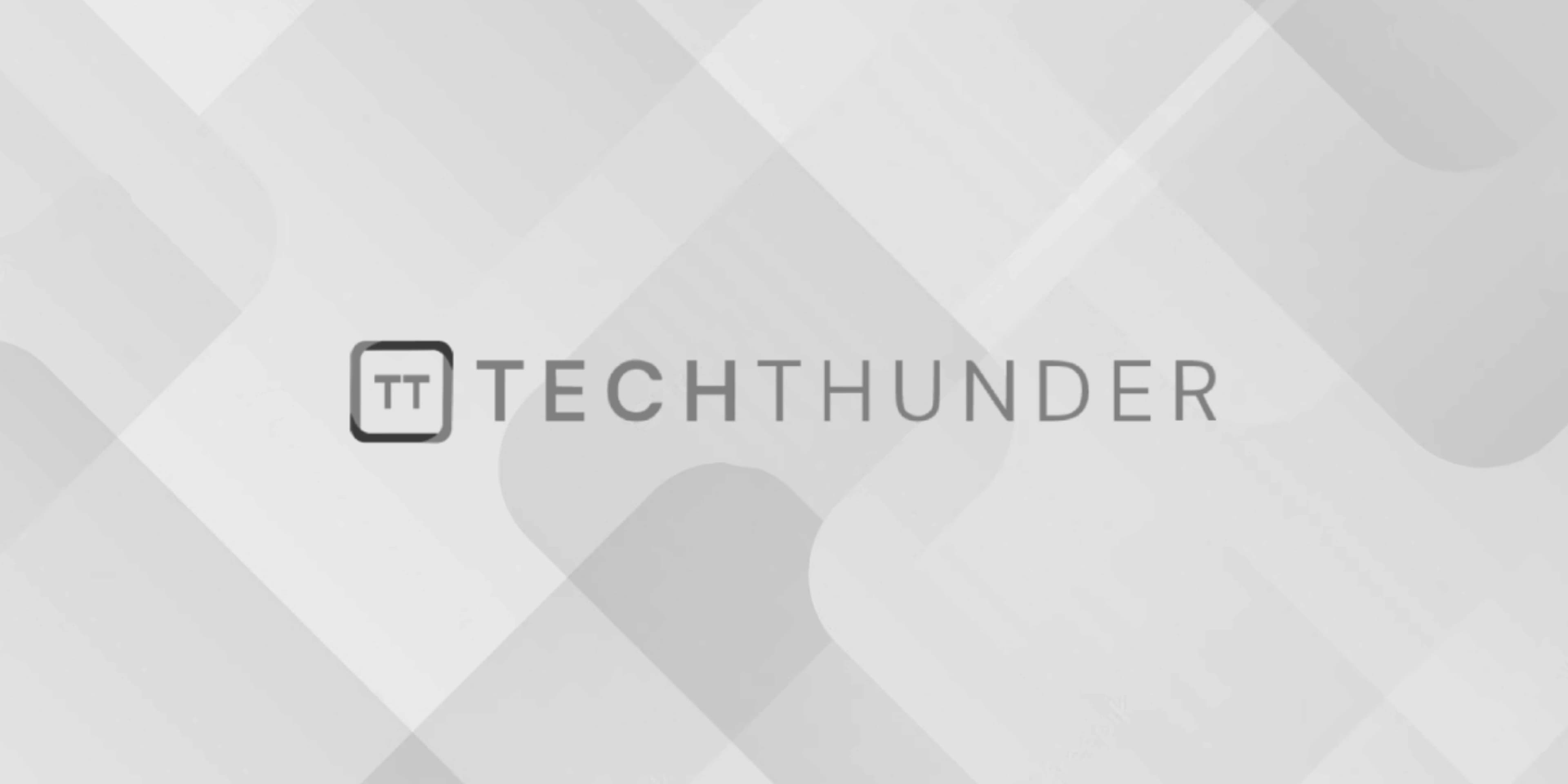
Recording Media in Android
Recording media in Android involves capturing audio and video data using the device’s microphone and camera. Android provides built-in classes and libraries to make media recording and playback relatively straightforward. Here’s a guide on how to record media in Android:
1. Permissions:
Before you can record media, you need to request the appropriate permissions in your AndroidManifest.xml
file and at runtime for Android 6.0 (API level 23) and higher. For audio and camera recording, you typically need the following permissions:
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.CAMERA" />
For writing to storage if you plan to save the recorded media:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
2. Initialize MediaRecorder or Camera API:
Depending on whether you’re recording audio or video, you’ll need to set up either the MediaRecorder
class for audio or the Camera API for video (and possibly audio too). Here are the basics for both:
Audio Recording:
private MediaRecorder mediaRecorder;
// Initialize and configure MediaRecorder
mediaRecorder = new MediaRecorder();
mediaRecorder.setAudioSource(MediaRecorder.AudioSource.MIC);
mediaRecorder.setOutputFormat(MediaRecorder.OutputFormat.THREE_GPP);
mediaRecorder.setAudioEncoder(MediaRecorder.AudioEncoder.AMR_NB);
mediaRecorder.setOutputFile("your_output_file.3gp");
// Prepare MediaRecorder
try {
mediaRecorder.prepare();
} catch (IOException e) {
e.printStackTrace();
}
// Start recording
mediaRecorder.start();
Video Recording (with Audio):
private Camera camera;
private MediaRecorder mediaRecorder;
// Initialize the camera
camera = Camera.open();
// Initialize and configure MediaRecorder
mediaRecorder = new MediaRecorder();
camera.unlock();
mediaRecorder.setCamera(camera);
mediaRecorder.setAudioSource(MediaRecorder.AudioSource.CAMCORDER);
mediaRecorder.setVideoSource(MediaRecorder.VideoSource.CAMERA);
mediaRecorder.setOutputFormat(MediaRecorder.OutputFormat.MPEG_4);
mediaRecorder.setAudioEncoder(MediaRecorder.AudioEncoder.DEFAULT);
mediaRecorder.setVideoEncoder(MediaRecorder.VideoEncoder.DEFAULT);
mediaRecorder.setOutputFile("your_output_file.mp4");
// Prepare MediaRecorder
try {
mediaRecorder.prepare();
} catch (IOException e) {
e.printStackTrace();
}
// Start recording
mediaRecorder.start();
3. Stop Recording:
To stop recording, you’ll need to call the stop
and release
methods for both MediaRecorder
and the camera:
// Stop recording and release resources
mediaRecorder.stop();
mediaRecorder.release();
mediaRecorder = null;
camera.lock();
camera.release();
camera = null;
4. Handle Exceptions:
Be sure to handle exceptions that might occur during initialization, recording, or releasing resources.
5. Save Recorded Media:
If you want to save the recorded media to the device’s storage, you can specify the output file path in the setOutputFile
method when initializing the MediaRecorder
. After recording, the media will be saved at that location.
6. Playback and Preview:
To play back or preview the recorded media, you can use the Android MediaPlayer class for audio or display the video using a VideoView or a SurfaceView for video.
7. Testing:
Thoroughly test your media recording functionality on different devices and Android versions to ensure it works as expected.
Recording media in Android requires careful management of resources and proper handling of exceptions. It’s also essential to consider the user experience, permissions, and storage management when implementing media recording in your app.