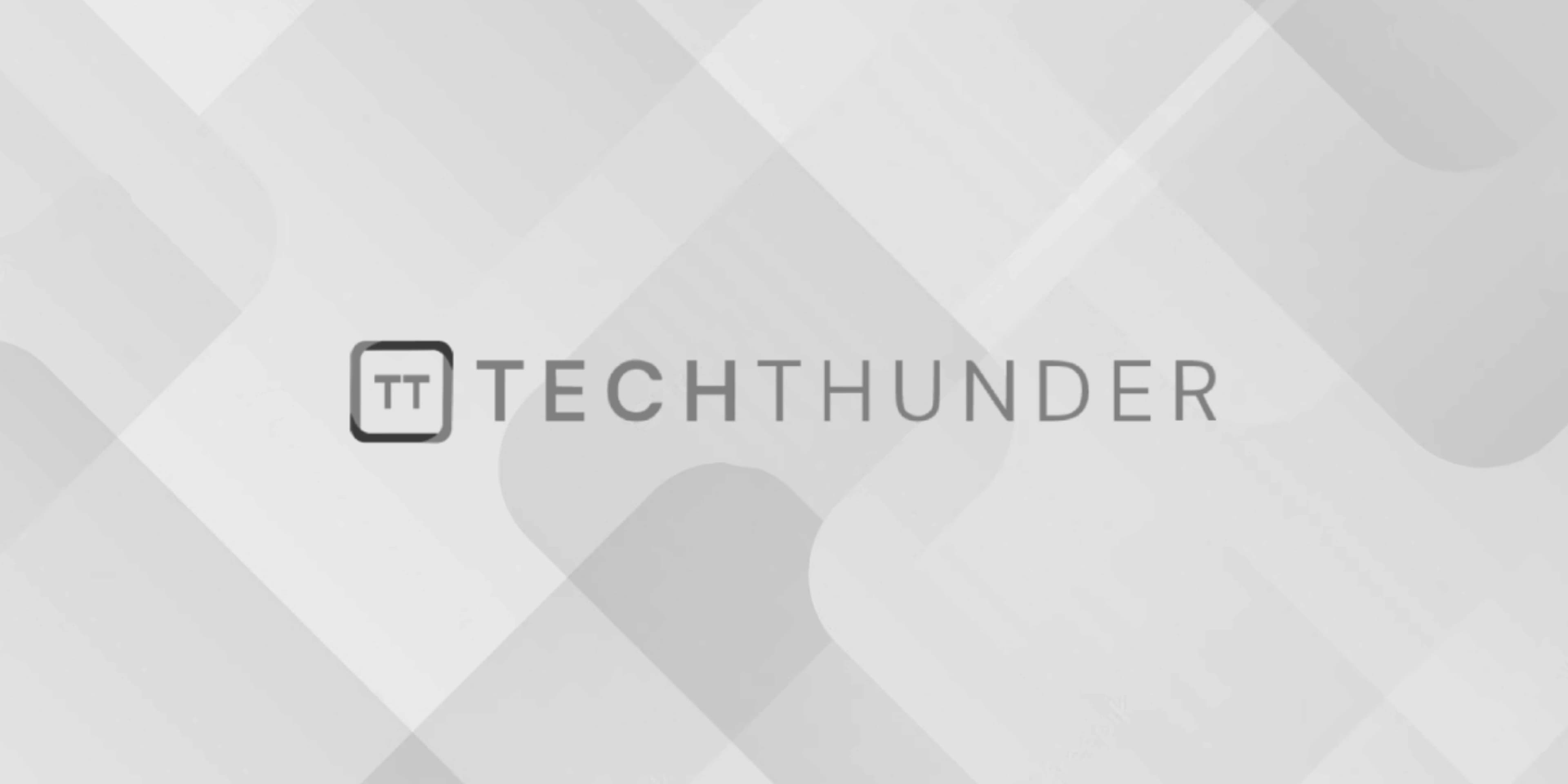
MediaPlayer: Audio in Android
The MediaPlayer
class in Android allows you to play audio and video files in your Android app. In this guide, I’ll show you how to use MediaPlayer
to play audio files.
1. Prepare Your Audio File:
Make sure you have an audio file (e.g., MP3, WAV, OGG) that you want to play in your Android app. Place the audio file in the appropriate location in your project’s resources (usually in the res/raw
folder).
2. Initialize MediaPlayer
:
In your activity or fragment, create a MediaPlayer
object and set up the audio source. You should also prepare the MediaPlayer
for playback.
import android.media.MediaPlayer;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
public class AudioPlayerActivity extends AppCompatActivity {
private MediaPlayer mediaPlayer;
private Button playButton;
private boolean isPlaying = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_audio_player);
mediaPlayer = MediaPlayer.create(this, R.raw.your_audio_file); // Replace with your audio file name
playButton = findViewById(R.id.playButton);
playButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!isPlaying) {
mediaPlayer.start();
playButton.setText("Pause");
} else {
mediaPlayer.pause();
playButton.setText("Play");
}
isPlaying = !isPlaying;
}
});
}
@Override
protected void onDestroy() {
super.onDestroy();
if (mediaPlayer != null) {
mediaPlayer.release();
mediaPlayer = null;
}
}
}
In this example:
- We create a
MediaPlayer
object and load the audio file from theres/raw
folder usingMediaPlayer.create()
. Replace"your_audio_file"
with the actual name of your audio file without the file extension. - We set up a
Button
that starts or pauses playback when clicked. - In the
onClick
listener, we check whether the audio is currently playing or paused, and we toggle playback accordingly. - In the
onDestroy
method, we release theMediaPlayer
resources to free up system resources when the activity is destroyed.
3. Create Layout XML:
Create a layout XML file for your activity. In this example, we have a simple layout with a play/pause button:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:context=".AudioPlayerActivity">
<Button
android:id="@+id/playButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Play"
android:textSize="18sp" />
</RelativeLayout>
4. Permissions:
If you’re targeting Android 6.0 (API level 23) or higher, you need to request the INTERNET
and WAKE_LOCK
permissions in your AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
5. Testing:
Test your audio playback functionality on different devices to ensure it works as expected.
This example demonstrates basic audio playback using MediaPlayer
. Depending on your app’s requirements, you can customize the audio playback further, add features like seeking, volume control, and handle playback completion or errors.