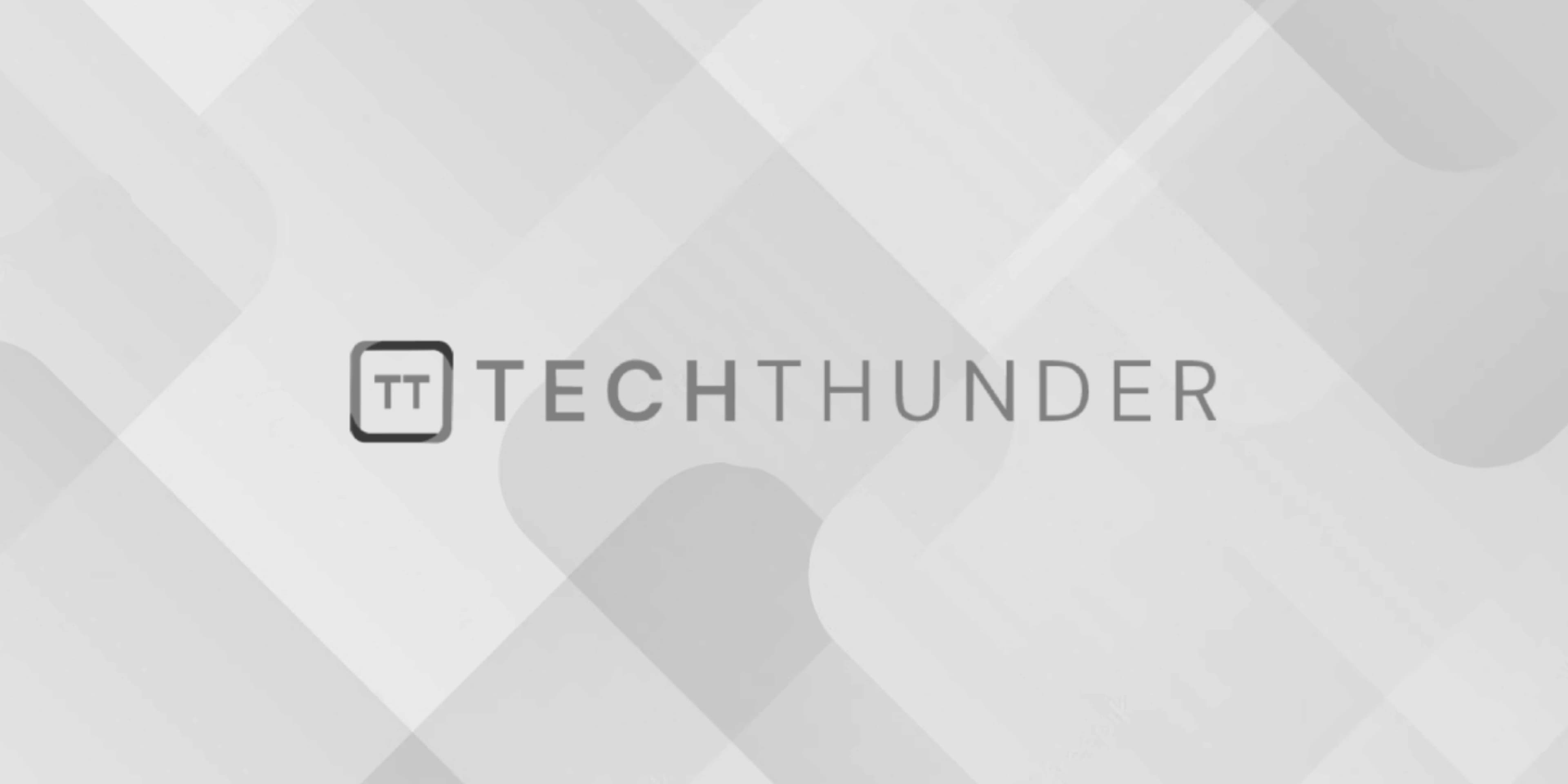
XQuery FLWOR
In XQuery, FLWOR (For-Let-Where-Order by-Return) expressions are used to perform iterations, filtering, sorting, and returning results in a structured and readable way. FLWOR expressions are similar to SQL queries and provide a convenient and powerful way to process and transform XML data.
The components of a FLWOR expression are as follows:
- For: The “for” clause defines the variable(s) and their associated sequences over which the iteration will occur.
- Let: The “let” clause allows you to define new variables for use within the FLWOR expression.
- Where: The “where” clause specifies the condition that each item in the sequence must satisfy in order to be included in the result.
- Order by: The “order by” clause sorts the items in the sequence based on a specified order.
- Return: The “return” clause defines the result to be returned for each item in the sequence after the iterations, filtering, and sorting are applied.
Here’s a general structure of a FLWOR expression:
for $variable in $sequence
let $newVariable := some-expression
where some-condition
order by $sortExpression
return some-result
Let’s see an example of a FLWOR expression in XQuery:
let $numbers := (5, 2, 7, 1, 8)
for $num in $numbers
where $num > 3
order by $num descending
return $num * 2
Output:
16
14
10
In this example, we have a sequence of numbers stored in the variable $numbers
. The FLWOR expression iterates over each item in the sequence using the “for” clause (for $num in $numbers
). The “where” clause filters out the numbers greater than 3 (where $num > 3
), and the “order by” clause sorts the remaining numbers in descending order based on their values (order by $num descending
). Finally, the “return” clause computes the result by doubling each remaining number (return $num * 2
).
FLWOR expressions are a powerful feature of XQuery, allowing you to perform complex data processing and transformations in a declarative and readable manner. They are widely used for querying and manipulating XML data efficiently.