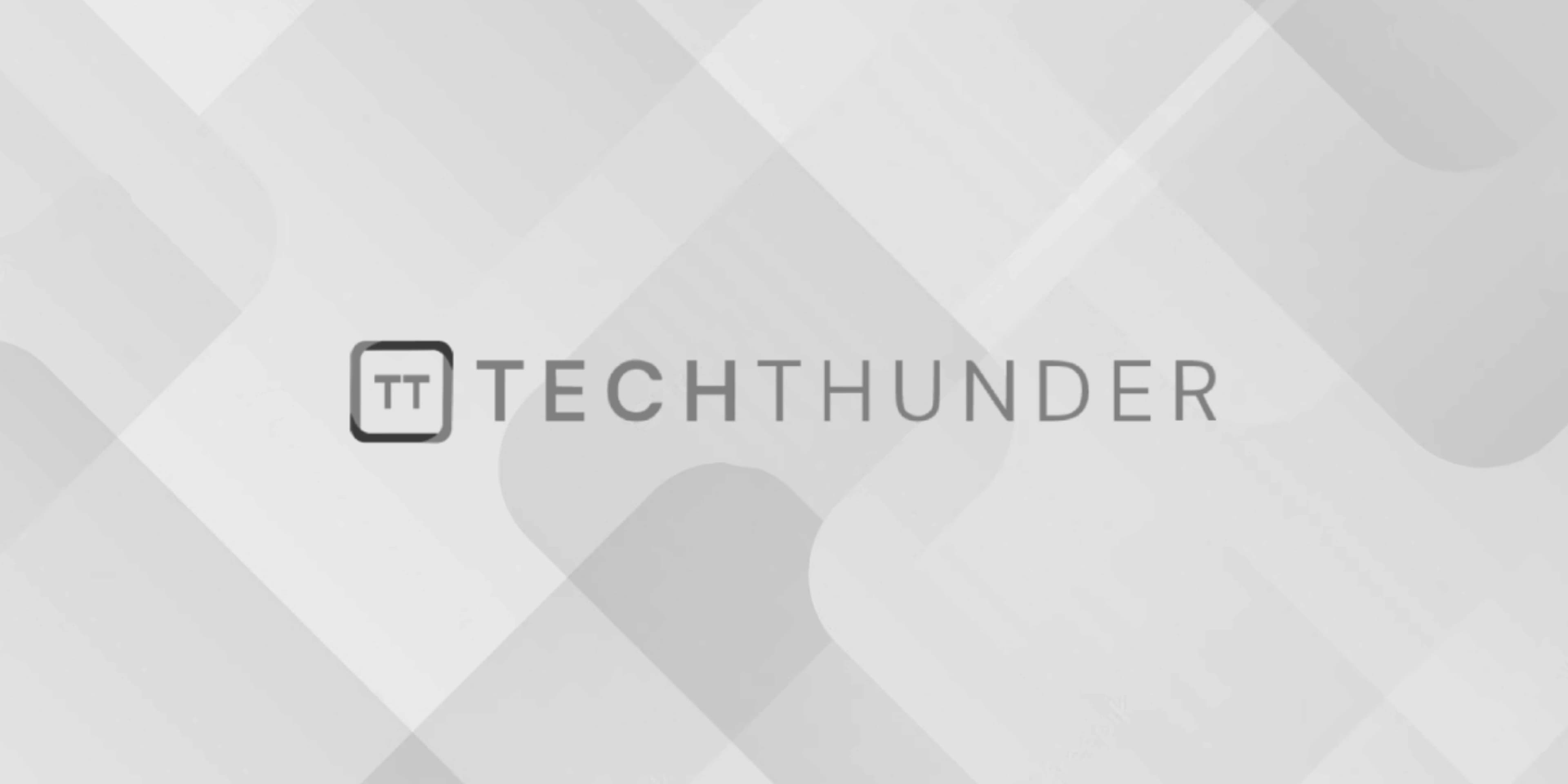
127 views
XQuery Syntax
XQuery is a powerful language designed for querying and manipulating XML data. Its syntax is similar to that of other functional programming languages, and it uses XML-like syntax for constructing and processing XML data. Here’s an overview of the basic syntax elements in XQuery:
- Elements and Attributes:
- XML elements and attributes are represented using angle brackets
< >
. - Example:
<book>...</book>
represents an XML element named “book.”
- Variables:
- Variables in XQuery start with a dollar sign
$
. - Example:
$name
,$age
, etc.
- Literals:
- String literals are enclosed in double quotes (
"
) or single quotes ('
). - Numeric literals are just numbers, e.g.,
42
,3.14
. - Boolean literals are
true()
andfalse()
.
- Sequences:
- Sequences are ordered collections of items and are represented using parentheses
()
. - Example:
(1, 2, 3)
,("apple", "banana")
, etc.
- Comments:
- XQuery supports single-line comments starting with
(:
and ending with:)
. - Example:
(: This is a comment :)
- FLWOR Expressions:
- FLWOR expressions (For-Let-Where-Order by-Return) are used for iterations, filtering, sorting, and returning results.
- Example:
xquery for $book in $books where $book/price > 20 order by $book/title return $book/title
- Functions:
- XQuery has built-in functions for various operations on XML data.
- Functions are called using the function name followed by parentheses and arguments if any.
- Example:
fn:string-length($text)
,fn:sum($numbers)
, etc.
- Predicates:
- Predicates are used to filter nodes or items in sequences based on conditions.
- Predicates are enclosed in square brackets
[]
. - Example:
//book[@category='fiction']
selects all<book>
elements with the attributecategory
equal to ‘fiction’.
- Whitespace Handling:
- By default, XQuery ignores whitespace, but you can control it using the
preserve
andstrip
options.
- XML Output Method:
- The output method specifies how XML or HTML results are serialized.
- Example:
declare option output:method 'xml';
,declare option output:method 'html';
.
XQuery is a flexible and expressive language, allowing you to work with XML data efficiently. It’s worth noting that XQuery also allows you to perform complex transformations, grouping, and aggregations on XML data, making it a powerful tool for handling XML-based tasks.