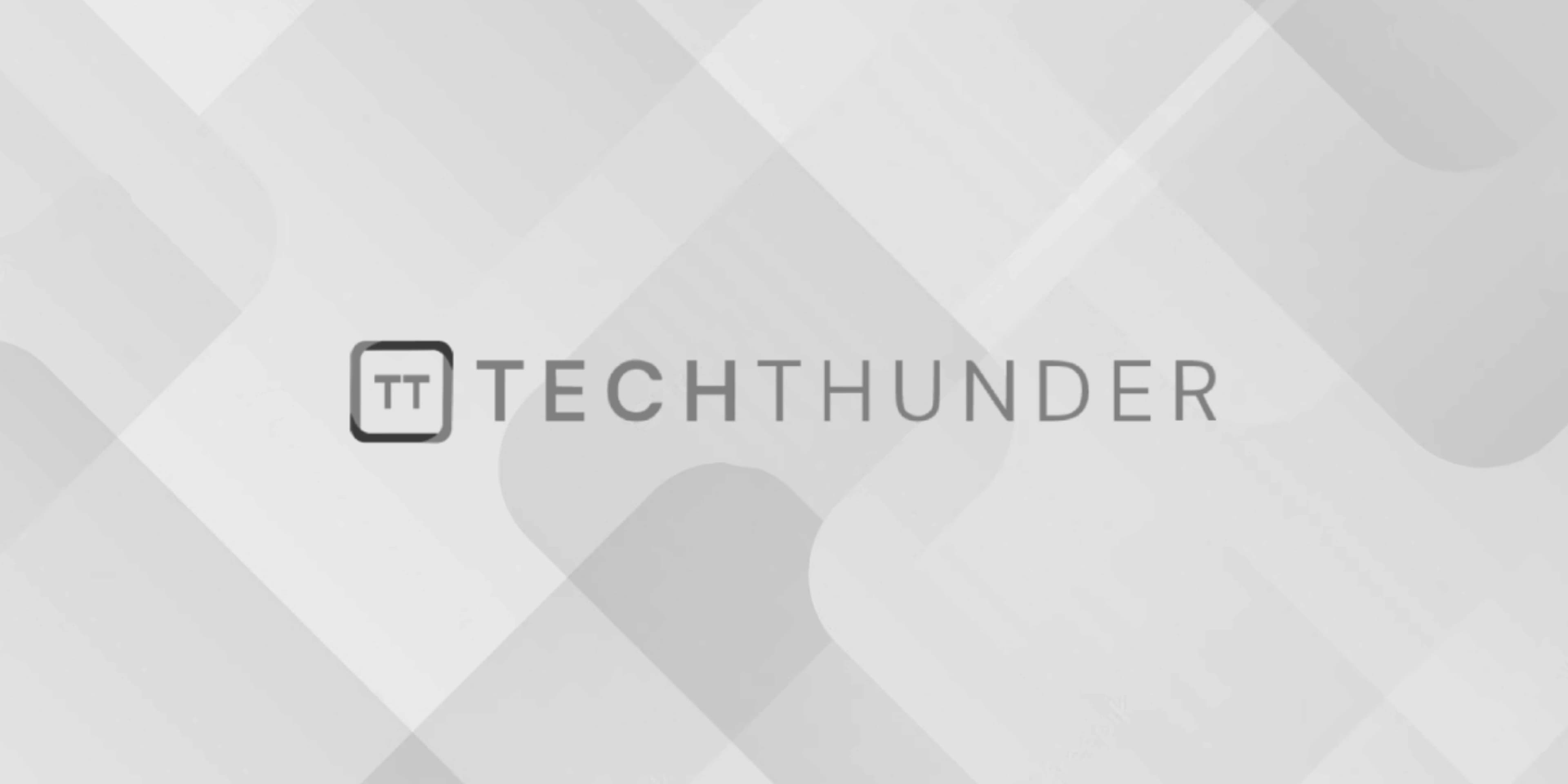
XSLT xsl:choose
In XSLT (Extensible Stylesheet Language Transformations), the xsl:choose
element is used to perform conditional processing. It is part of the XSLT control structures and allows you to choose one of several alternative blocks of code to execute based on specific conditions.
The xsl:choose
element is often used in conjunction with xsl:when
and xsl:otherwise
elements to define the different conditional branches. Here’s the basic syntax of the xsl:choose
element:
<xsl:choose>
<xsl:when test="condition-1">
<!-- Code block to execute when condition-1 is true -->
</xsl:when>
<xsl:when test="condition-2">
<!-- Code block to execute when condition-2 is true -->
</xsl:when>
<!-- More <xsl:when> blocks can be added as needed -->
<xsl:otherwise>
<!-- Code block to execute if none of the conditions are true -->
</xsl:otherwise>
</xsl:choose>
In this structure:
- The
xsl:when
elements are used to define individual conditions. Eachxsl:when
element has atest
attribute that specifies the XPath expression for evaluating the condition. - The
xsl:otherwise
element is optional and provides a default code block to execute if none of the conditions in thexsl:when
elements are true.
The xsl:choose
element evaluates the conditions in order and executes the code block corresponding to the first true condition. If none of the conditions are true and there is an xsl:otherwise
element, its code block will be executed.
Here’s an example of using xsl:choose
to handle different salary ranges:
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<xsl:choose>
<xsl:when test="salary < 30000">
<xsl:text>Low salary.</xsl:text>
</xsl:when>
<xsl:when test="salary < 50000">
<xsl:text>Medium salary.</xsl:text>
</xsl:when>
<xsl:otherwise>
<xsl:text>High salary.</xsl:text>
</xsl:otherwise>
</xsl:choose>
</xsl:template>
</xsl:stylesheet>
In this example, if the value of the “salary” element in the XML input is less than 30,000, it will output “Low salary.” If it is between 30,000 and 50,000, it will output “Medium salary.” Otherwise, it will output “High salary.”
xsl:choose
is a powerful construct in XSLT that allows you to handle complex conditional logic and tailor the transformation output based on specific conditions in the input data.