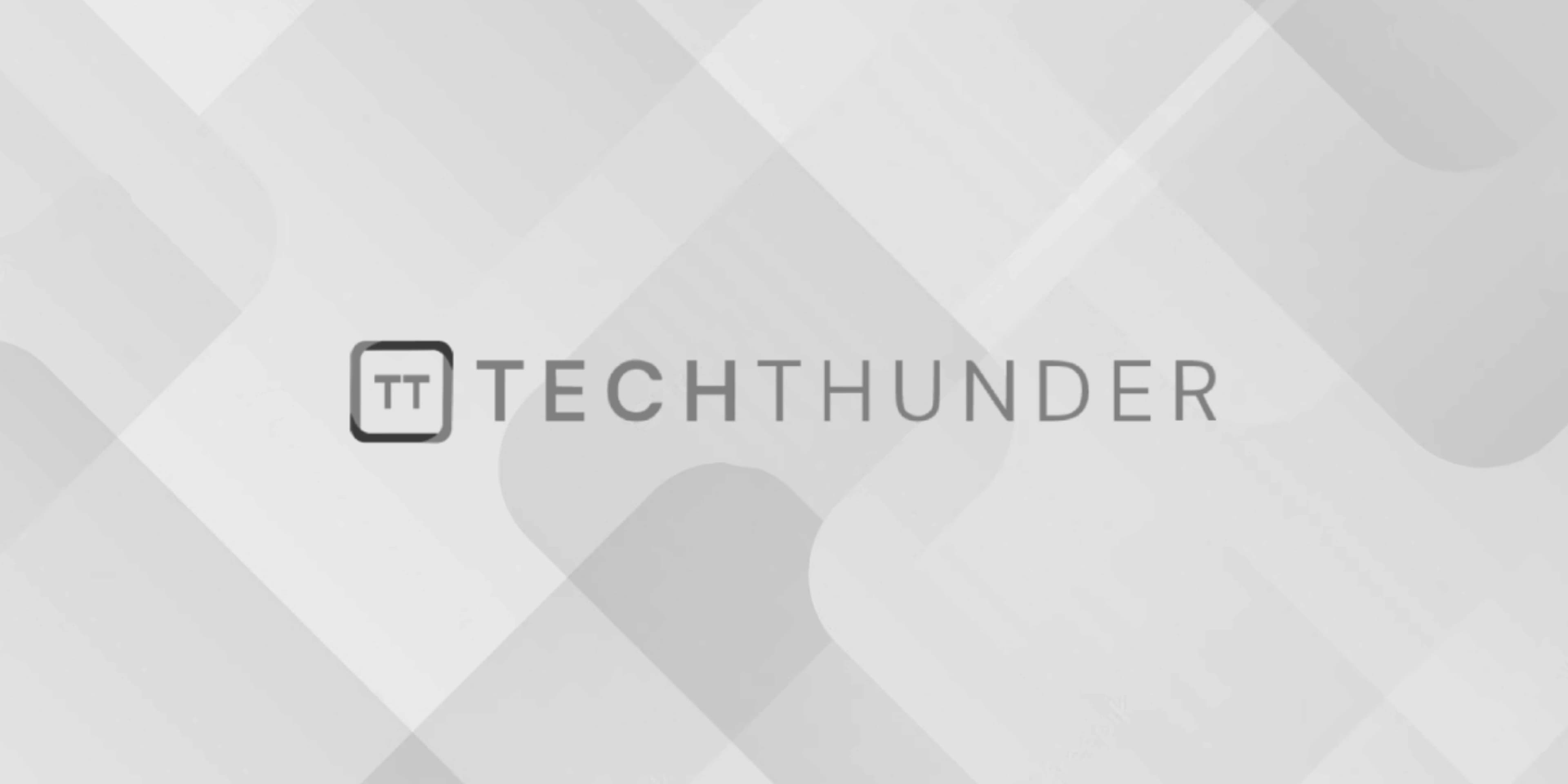
XQuery Tutorial
Sure! Let’s go through a basic XQuery tutorial to help you get started with the language. In this tutorial, we’ll cover some essential concepts of XQuery and walk you through a few examples.
1. XQuery Basics:
- XQuery is a functional and declarative language used for querying and processing XML data.
- It is similar to SQL for querying relational databases but is specifically designed for working with XML.
- XQuery uses FLWOR (For-Let-Where-Order by-Return) expressions for iterations, filtering, and returning results.
2. XQuery Environment Setup:
- To run XQuery, you need an XQuery processor or editor installed on your computer.
- Some popular XQuery processors are Saxon, BaseX, and Zorba.
- Alternatively, you can use XQuery plugins or extensions in XML editors like Oxygen XML Editor or IntelliJ IDEA.
3. Basic XQuery Example:
- Let’s start with a simple example to extract data from an XML document.
XML Document (data.xml):
<books>
<book>
<title>XQuery 101</title>
<author>John Doe</author>
<price>25.00</price>
</book>
<book>
<title>XML Fundamentals</title>
<author>Jane Smith</author>
<price>30.00</price>
</book>
</books>
XQuery to Extract Book Titles:
for $book in doc("data.xml")//book
return $book/title
Output:
<title>XQuery 101</title>
<title>XML Fundamentals</title>
Explanation:
- The XQuery iterates over each
<book>
element using the “for” clause. - The “return” clause specifies what to return for each iteration, which is the
<title>
element of each<book>
.
4. More XQuery Examples:
- Let’s explore more XQuery expressions to see what else you can do with it.
a) Filtering and Conditional Statements:
for $book in doc("data.xml")//book
where $book/price > 25
return $book/title
Output:
<title>XML Fundamentals</title>
Explanation:
- This XQuery filters books with a price greater than 25 and returns their titles.
b) Using Let Clauses:
for $book in doc("data.xml")//book
let $formattedPrice := concat("$", $book/price)
return <book>{ $book/title } - Price: { $formattedPrice }</book>
Output:
<book>XQuery 101 - Price: $25.00</book>
<book>XML Fundamentals - Price: $30.00</book>
Explanation:
- This XQuery uses a “let” clause to define a new variable
$formattedPrice
to store the formatted price with a dollar sign. - The “return” clause creates a new
<book>
element with the title and formatted price.
5. XML Namespaces:
- If your XML document contains namespaces, you can use prefixes to access elements within namespaces.
XML Document with Namespace (data.xml):
<books xmlns="http://www.example.com/books">
<book>
<title>XQuery 101</title>
<author>John Doe</author>
<price>25.00</price>
</book>
</books>
XQuery to Access Elements with Namespace:
declare namespace b="http://www.example.com/books";
for $book in doc("data.xml")//b:book
return $book/b:title
Output:
<title>XQuery 101</title>
Explanation:
- The “declare namespace” statement declares a namespace prefix
b
for the namespace URI “http://www.example.com/books”. - The XQuery uses this namespace prefix to access elements within the “http://www.example.com/books” namespace.
These examples provide a basic understanding of XQuery’s capabilities. As you continue to explore the language, you’ll find XQuery to be a powerful tool for querying and processing XML data efficiently. Feel free to experiment with more complex queries and explore XQuery’s various functions and features to fully utilize its potential.