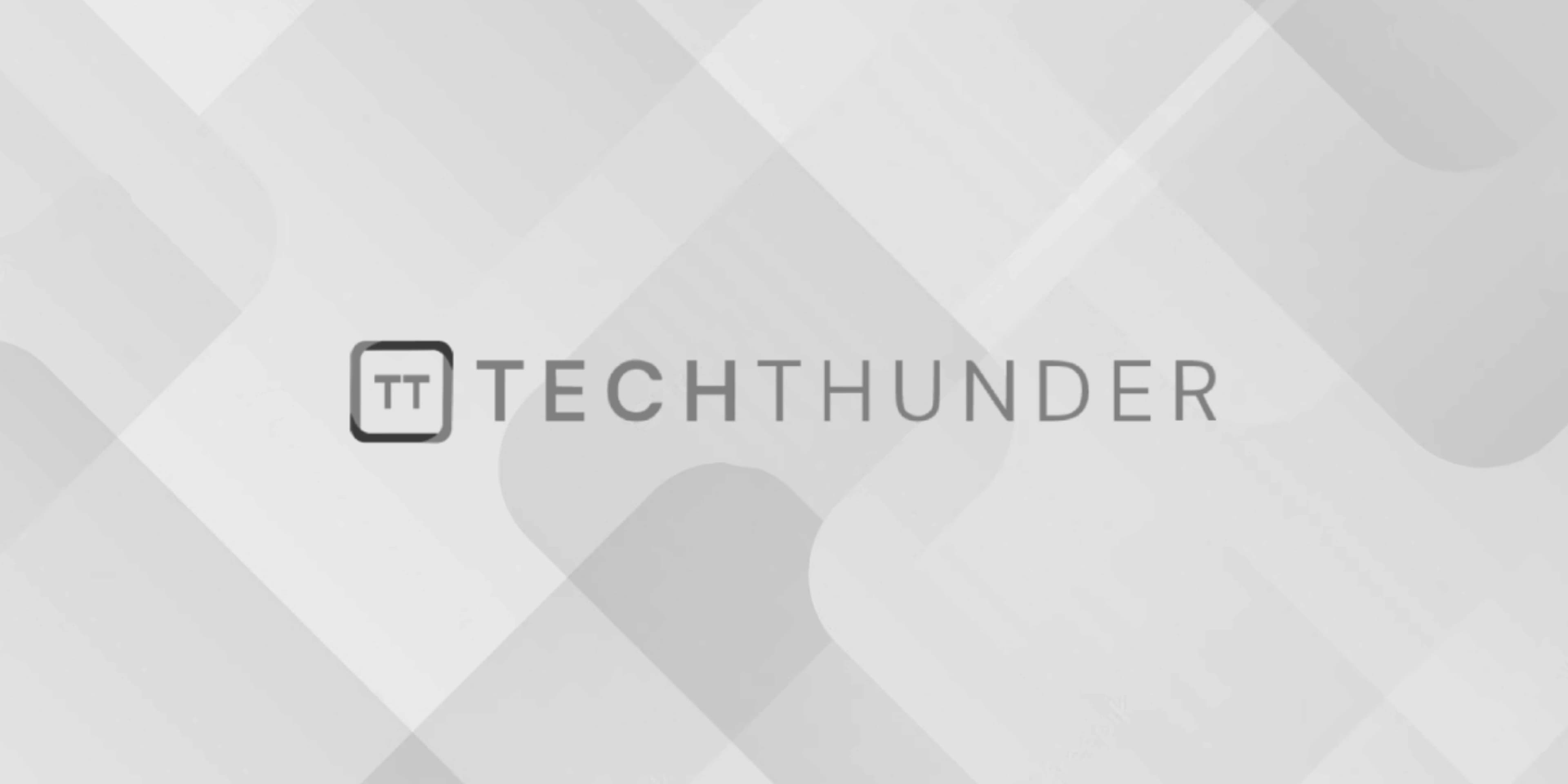
CSS overlay
In CSS, an overlay is a technique used to create a translucent layer on top of an element or an entire webpage. The overlay is often used to create modal dialogs, lightboxes, or hover effects, where the content behind the overlay is partially obscured to draw attention to the overlayed content.
Creating an overlay typically involves using a combination of CSS properties, such as position
, z-index
, background
, and opacity
. Below is an example of how to create a simple overlay for a modal dialog:
HTML:
<button id="open-modal-btn">Open Modal</button>
<div class="overlay" id="modal-overlay">
<div class="modal">
<h2>Modal Content</h2>
<p>This is the content of the modal dialog.</p>
<button id="close-modal-btn">Close Modal</button>
</div>
</div>
CSS:
/* Hide the overlay by default */
.overlay {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5); /* Translucent black color */
z-index: 9999; /* Make the overlay appear above other content */
}
/* Center the modal dialog */
.modal {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #fff;
padding: 20px;
}
/* Show the overlay when the modal is active */
.overlay.active {
display: block;
}
JavaScript (optional, to show/hide the overlay):
const openModalBtn = document.getElementById('open-modal-btn');
const closeModalBtn = document.getElementById('close-modal-btn');
const modalOverlay = document.getElementById('modal-overlay');
openModalBtn.addEventListener('click', () => {
modalOverlay.classList.add('active');
});
closeModalBtn.addEventListener('click', () => {
modalOverlay.classList.remove('active');
});
In this example, we have a simple HTML structure with a button that triggers the modal and an overlay with a modal dialog inside it. The CSS styles the overlay to cover the entire viewport with a translucent black background color (rgba(0, 0, 0, 0.5)
). The modal dialog is centered both horizontally and vertically on the screen.
The JavaScript code is optional but is used to show and hide the overlay by adding or removing the active
class from the .overlay
element. You can use your preferred method (e.g., CSS :target
pseudo-class or JavaScript) to control the visibility of the overlay.
This is a basic example of creating a CSS overlay. You can further customize and enhance it based on your specific use case and design requirements. For more complex overlays or animations, you may consider using CSS transitions or animations.