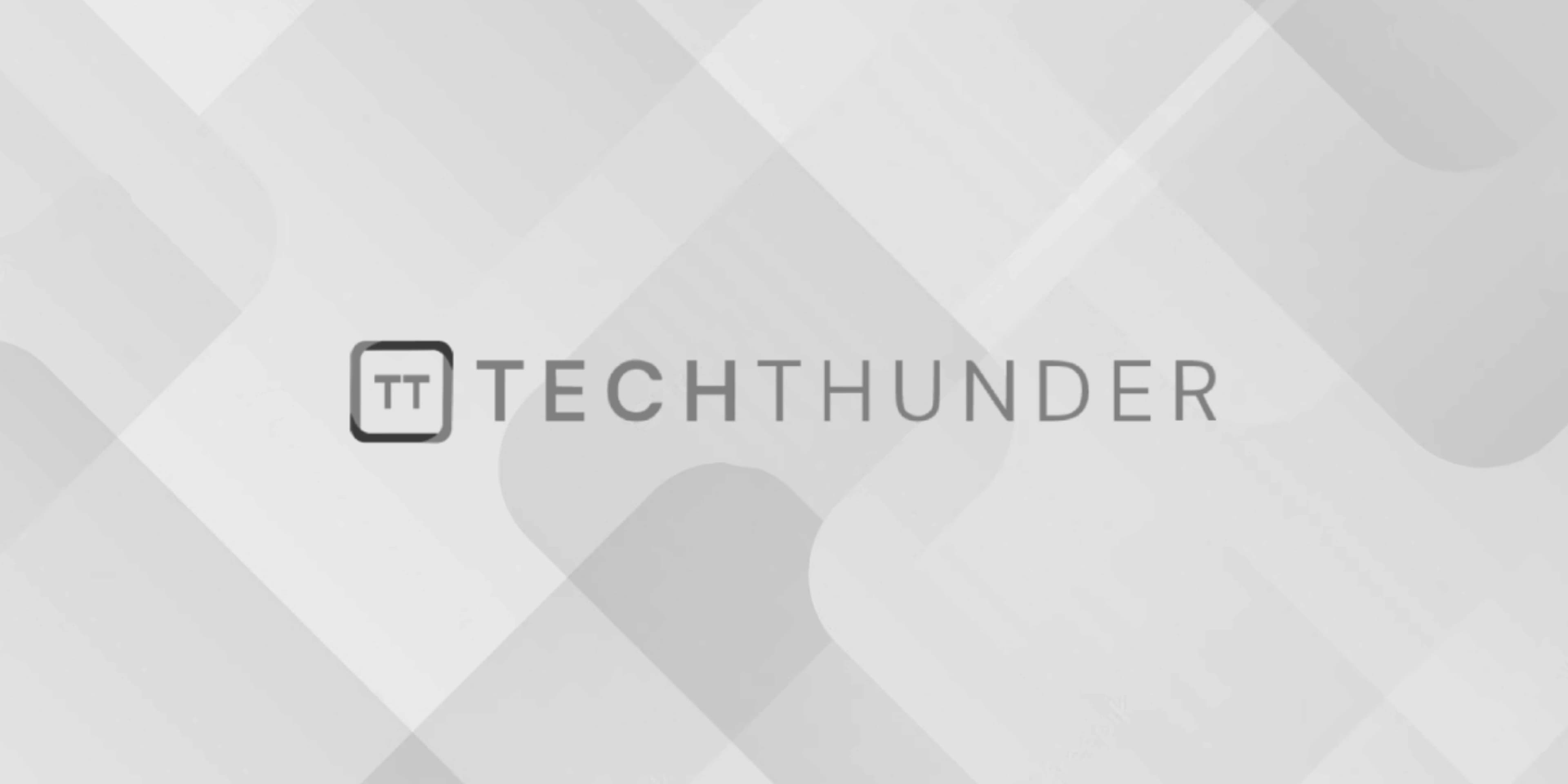
Timetable template using CSS Grid
Creating a timetable template using CSS Grid is a great way to organize and display schedules in a structured and visually appealing manner. CSS Grid provides a two-dimensional layout system that is well-suited for creating tables and grids. Here’s a basic example of a timetable template using CSS Grid:
HTML:
<div class="timetable">
<div class="header">Time</div>
<div class="header">Monday</div>
<div class="header">Tuesday</div>
<div class="header">Wednesday</div>
<div class="header">Thursday</div>
<div class="header">Friday</div>
<div class="time">08:00 - 09:00</div>
<div class="cell">Math</div>
<div class="cell">Science</div>
<div class="cell">English</div>
<div class="cell">History</div>
<div class="cell">Art</div>
<div class="time">09:00 - 10:00</div>
<div class="cell">Science</div>
<div class="cell">Math</div>
<div class="cell">History</div>
<div class="cell">English</div>
<div class="cell">Music</div>
<!-- Add more time slots and cells for the rest of the timetable -->
</div>
CSS:
.timetable {
display: grid;
grid-template-columns: 100px repeat(5, 1fr); /* Adjust column widths as needed */
grid-template-rows: repeat(6, 50px); /* Adjust row heights as needed */
gap: 1px;
border: 1px solid #000;
}
.header, .time {
background-color: #f0f0f0;
text-align: center;
line-height: 50px;
font-weight: bold;
}
.cell {
background-color: #fff;
text-align: center;
line-height: 50px;
}
/* Optional: Add some styles to make it look better */
.timetable {
font-family: Arial, sans-serif;
}
.header {
font-size: 14px;
}
.time {
font-size: 16px;
}
.cell {
font-size: 14px;
border: 1px solid #ccc;
}
In this example, we create a timetable using a parent <div>
with the class “timetable.” Inside this container, we have grid items representing the header (days of the week), time slots, and cells for each subject.
Using CSS Grid, we define the grid layout using grid-template-columns
and grid-template-rows
properties. The repeat()
function allows us to create a repeating pattern for columns and rows. You can adjust the column widths, row heights, and other styles to fit your needs.
The header and time slots have a different background color to distinguish them from the cells. The cells can represent the subjects for each time slot, and you can add more time slots and cells for the rest of the timetable.