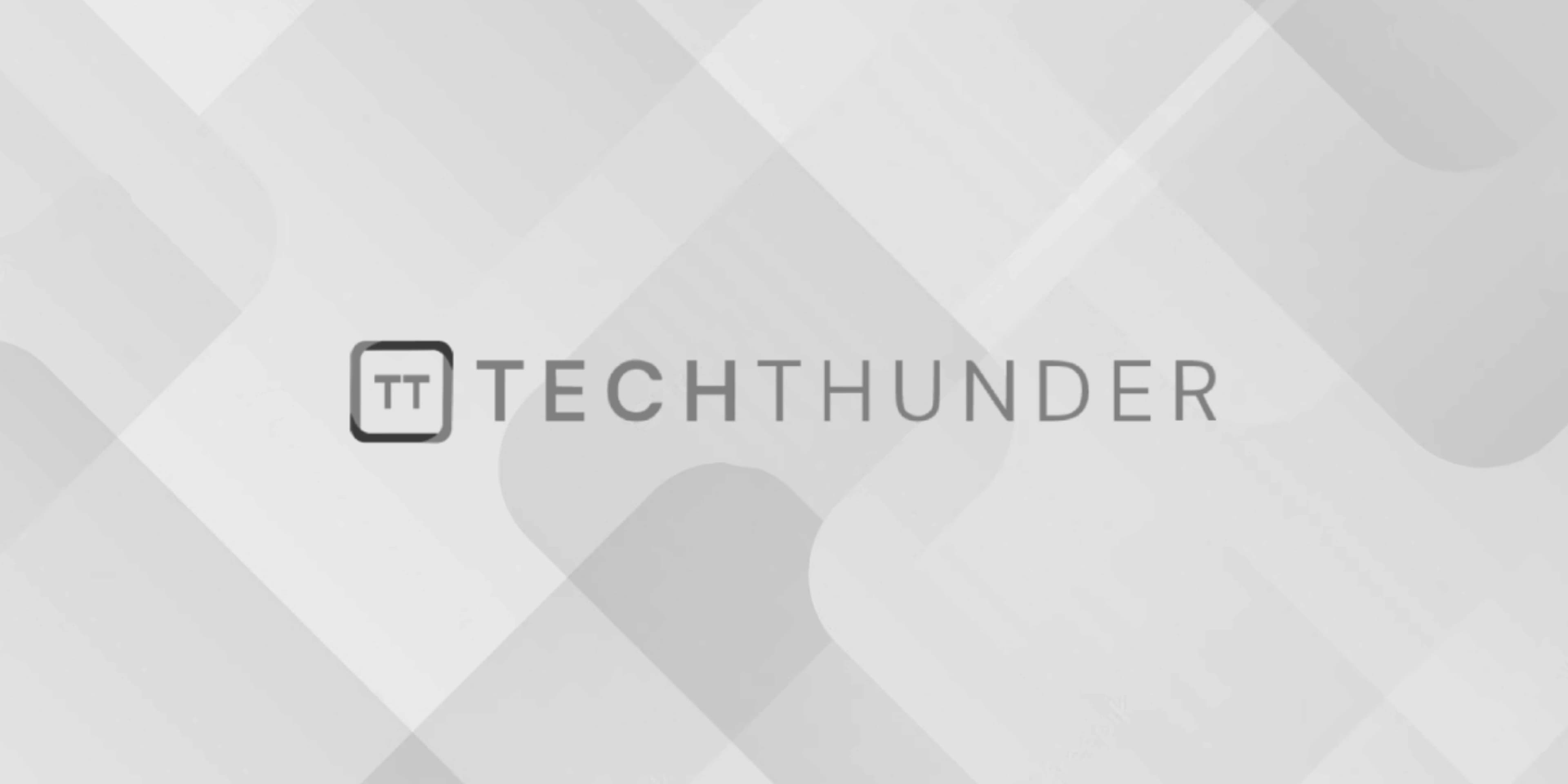
Record Animation using CSS
To create a simple animation using CSS, you can use the @keyframes
rule along with CSS properties to define the animation steps. Below is an example of how you can create a basic animation that makes an element move across the screen:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Record Animation using CSS</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="animation-box"></div>
</body>
</html>
CSS (styles.css):
body {
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
}
.animation-box {
width: 50px;
height: 50px;
background-color: #008cba;
position: relative;
animation: moveRight 3s linear infinite;
}
@keyframes moveRight {
0% {
left: 0;
}
100% {
left: calc(100% - 50px); /* Move to the right edge of the screen */
}
}
In this example, we’ve created a simple animation that moves a blue box from the left side to the right side of the screen. The @keyframes
rule defines the animation steps, and the animation
property applies the animation to the .animation-box
element. The animation will run infinitely, moving the box from left to right repeatedly.
To view the animation, create an HTML file with the provided HTML and CSS code, and open it in a web browser. You should see the blue box moving horizontally across the screen continuously. You can modify the animation properties and styles to create more complex animations according to your needs.