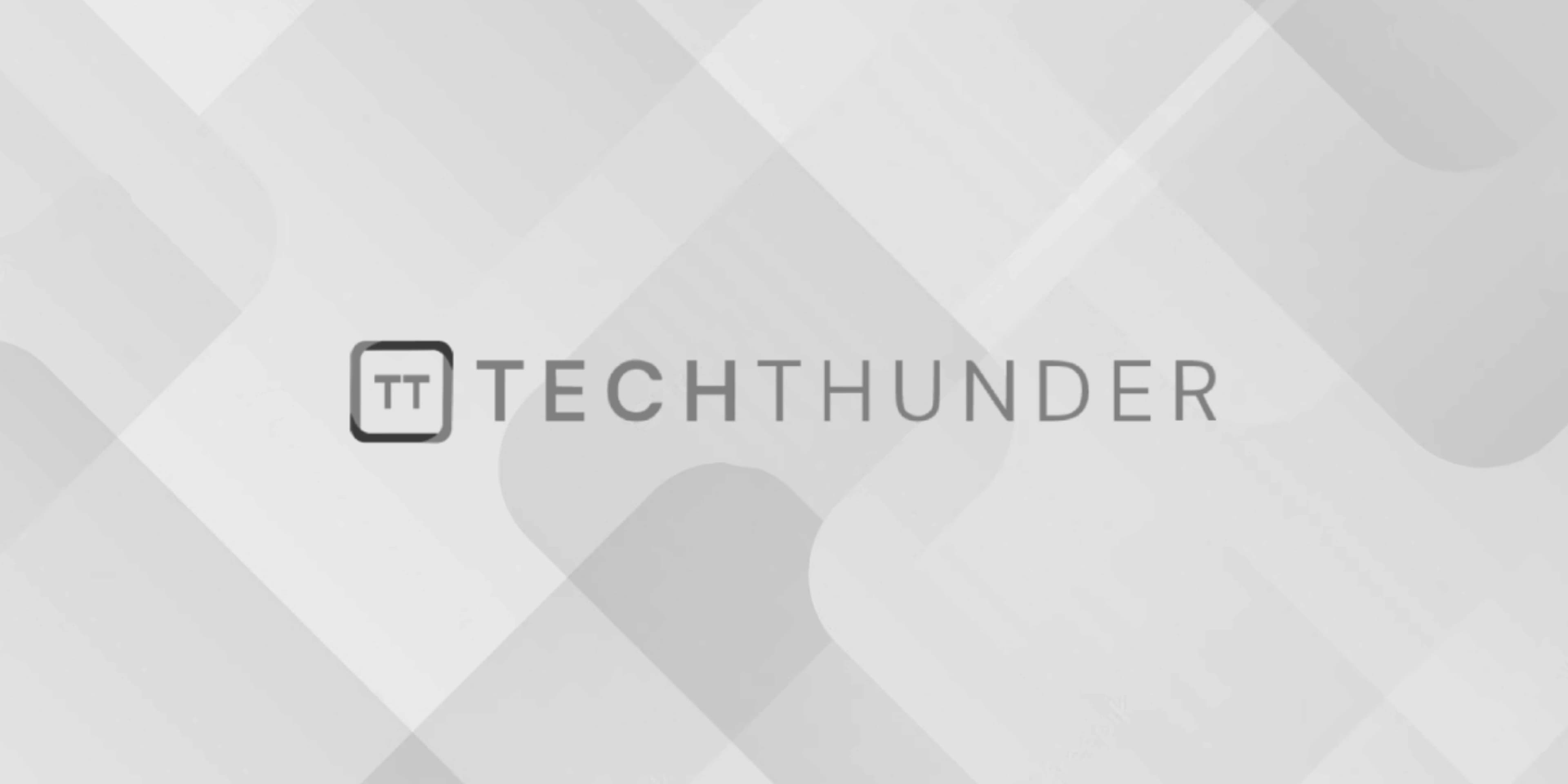
How to use the CSS grid
To use CSS Grid, you need to follow these steps:
- Create a Grid Container:
- To create a grid layout, you need to set a container element as a grid container by applying the
display: grid;
property to it. This property will activate the grid layout on the container and allow you to define its grid properties.
- Define Grid Rows and Columns:
- Once the container is set as a grid container, you can define the rows and columns of the grid using the
grid-template-rows
andgrid-template-columns
properties. These properties allow you to specify the size of each row and column in the grid.
- Place Grid Items:
- Now you can place elements inside the grid container, which will become grid items. Use the
grid-column
andgrid-row
properties to specify the starting and ending points of the grid items within the grid. Alternatively, you can use the shorthandgrid-area
property to place grid items in named grid areas.
Here’s an example to demonstrate using CSS Grid:
HTML:
<div class="grid-container">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
<div class="item">Item 4</div>
</div>
CSS:
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr); /* Three columns of equal width */
grid-gap: 10px; /* Gap between grid items */
}
.item {
background-color: #007bff;
color: white;
padding: 20px;
}
In this example, we create a grid container with the class “grid-container” and apply the display: grid;
property to it. We define three equal-width columns using grid-template-columns: repeat(3, 1fr);
. The grid-gap
property adds a 10px gap between grid items.
Each <div>
with the class “item” becomes a grid item within the grid container. The items will be automatically placed in the grid due to the default placement algorithm of CSS Grid.
CSS Grid is a powerful layout system that allows you to create flexible and responsive designs. You can adjust the number of columns, row heights, and grid item placement to achieve different layouts. Additionally, CSS Grid offers additional properties and features for more advanced grid layouts, such as grid templates, alignment, and named grid areas.