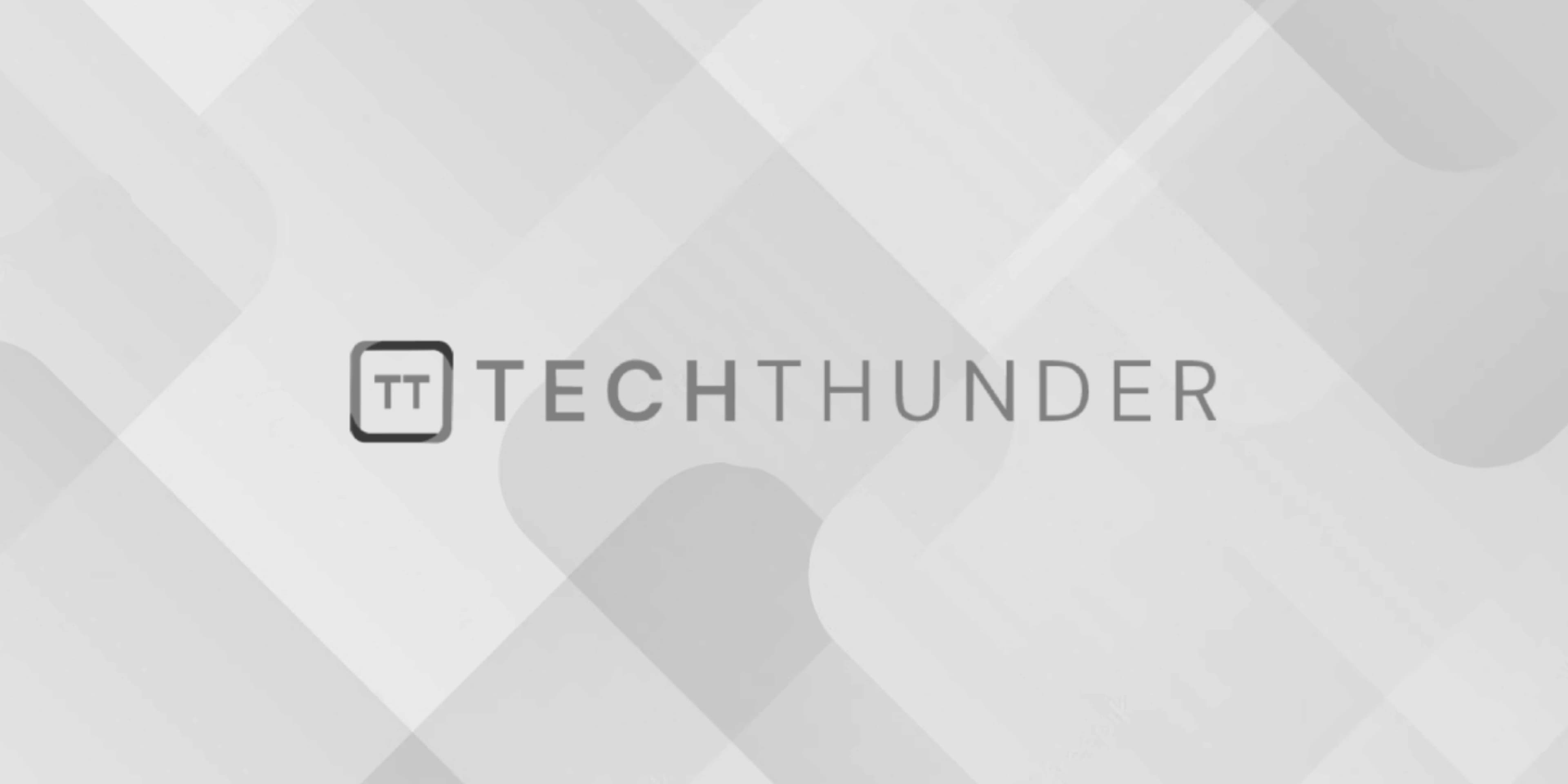
219 views
Java Custom Exceptions
To create your own custom exceptions by extending the existing exception classes provided by the Java Standard Library. This allows you to define specific exception types that are meaningful for your application and provide more informative error messages to aid in debugging. Here’s how you can create and use custom exceptions:
- Create a Custom Exception Class:
You should create a new class that extends an existing exception class (usuallyException
or one of its subclasses). This new class will represent your custom exception. You can add constructors to this class to provide additional information when creating instances of your custom exception.
public class CustomException extends Exception {
public CustomException() {
super();
}
public CustomException(String message) {
super(message);
}
public CustomException(String message, Throwable cause) {
super(message, cause);
}
}
- Throw Your Custom Exception:
In your code, when a certain condition indicates an exceptional situation, you can throw your custom exception using thethrow
keyword.
public class Example {
public void doSomething(int value) throws CustomException {
if (value < 0) {
throw new CustomException("Value cannot be negative");
}
// Rest of the method's logic
}
}
- Handle Your Custom Exception:
When calling the method that might throw your custom exception, you need to catch it using atry
–catch
block or declare it with thethrows
keyword in the method’s signature.
public class Main {
public static void main(String[] args) {
Example example = new Example();
try {
example.doSomething(-5);
} catch (CustomException e) {
System.out.println("Caught custom exception: " + e.getMessage());
}
}
}
By creating custom exceptions, you can make your code more organized and provide meaningful error handling. Custom exceptions can also be useful for creating a hierarchy of exception types that reflect the structure of your application’s errors. This makes it easier to distinguish between different types of issues and handle them appropriately.