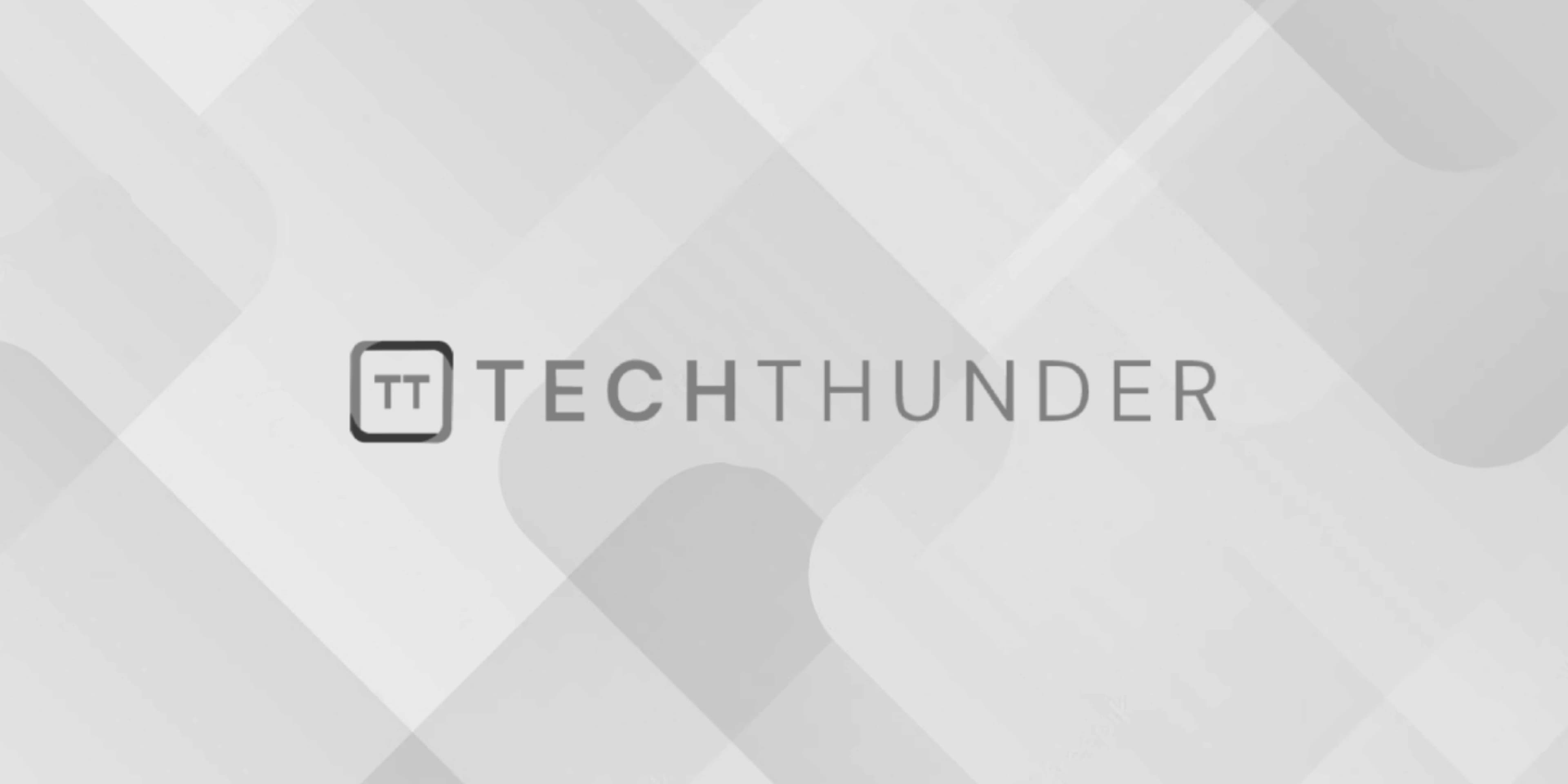
Java Multiple Catch Block
The multiple catch
blocks to handle different types of exceptions in java that might be thrown within a single try
block. This allows you to provide specific error-handling code for each type of exception that might occur. Here’s how you can use multiple catch
blocks:
try {
// Code that may throw exceptions
} catch (ExceptionType1 ex1) {
// Code to handle ExceptionType1
} catch (ExceptionType2 ex2) {
// Code to handle ExceptionType2
} catch (ExceptionType3 ex3) {
// Code to handle ExceptionType3
} catch (Exception ex) {
// Code to handle any other exceptions not caught by the previous catch blocks
}
In this structure, each catch
block specifies a different type of exception that it can handle. The Java runtime will evaluate each catch
block in order and execute the code within the first catch
block whose exception type matches the type of the thrown exception. If no match is found among the specific exception types, the code within the generic catch (Exception ex)
block will be executed.
Here’s a more concrete example:
public class MultipleCatchExample {
public static void main(String[] args) {
try {
int[] numbers = new int[3];
numbers[4] = 10; // This will throw an ArrayIndexOutOfBoundsException
} catch (ArrayIndexOutOfBoundsException ex1) {
System.out.println("Array index out of bounds: " + ex1.getMessage());
} catch (NullPointerException ex2) {
System.out.println("NullPointerException: " + ex2.getMessage());
} catch (Exception ex3) {
System.out.println("Generic exception: " + ex3.getMessage());
}
System.out.println("Program continues"); // This line will be reached
}
}
In this example, we catch an ArrayIndexOutOfBoundsException
and a NullPointerException
separately using different catch
blocks. If any other type of exception occurs, it will be caught by the generic catch (Exception ex)
block. Regardless of which exception is caught, the program continues executing after the try
–catch
block.
Using multiple catch
blocks allows you to tailor your error-handling logic to the specific types of exceptions that might arise, resulting in more precise and informative error handling.