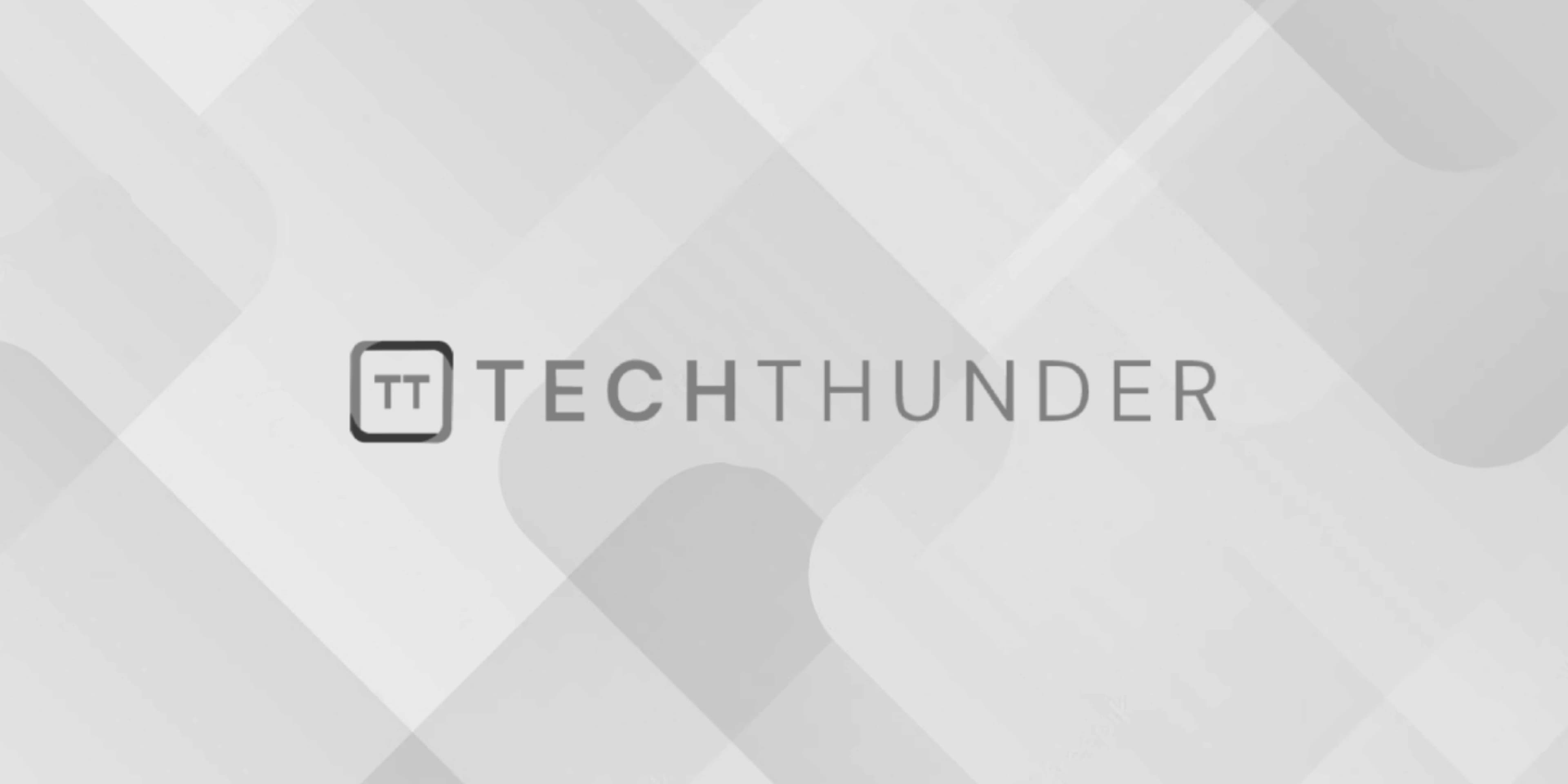
105 views
Java Final vs Finally vs Finalize
The final
, finally
, and finalize
in Java are three different concepts and keywords that serve distinct purposes:
final
:
final
is a keyword used to declare that a variable, method, or class cannot be further modified or extended.- When applied to a variable, it indicates that the variable’s value cannot be changed after it is initialized.
- When applied to a method, it indicates that the method cannot be overridden by subclasses.
- When applied to a class, it indicates that the class cannot be subclassed.
- Example:
final int constantValue = 10; public final void someMethod() { // Method implementation } final class FinalClass { // Class members }
finally
:
finally
is a block that follows atry
ortry
–catch
block and contains code that is executed regardless of whether an exception is thrown or caught.- It’s used for resource cleanup or ensuring that certain code is executed before a method exits, regardless of whether an exception occurred.
finally
is typically used in conjunction withtry
and optionallycatch
blocks.- Example:
try { // Code that may throw an exception } catch (Exception e) { // Exception handling } finally { // Code that will be executed no matter what }
finalize
:
finalize
is a method defined in theObject
class that’s called by the Java Virtual Machine (JVM) when an object is being garbage collected.- You can override the
finalize
method in your own classes to provide custom cleanup code that runs when an object is about to be reclaimed by the garbage collector. - However, it’s recommended to use other mechanisms (like
try
-with-resources or explicit resource management) for resource cleanup, as thefinalize
method is not guaranteed to be called promptly or at all. - Example:
public class MyResource { // Other members and methods @Override protected void finalize() throws Throwable { try { // Cleanup code } finally { super.finalize(); } } }
In summary:
final
is used to make a variable, method, or class unmodifiable.finally
is used in exception handling to ensure that specific code is executed regardless of whether an exception occurs.finalize
is a method that you can override to provide custom cleanup code when an object is being garbage collected, but its usage is limited due to uncertainty about when it will be called.