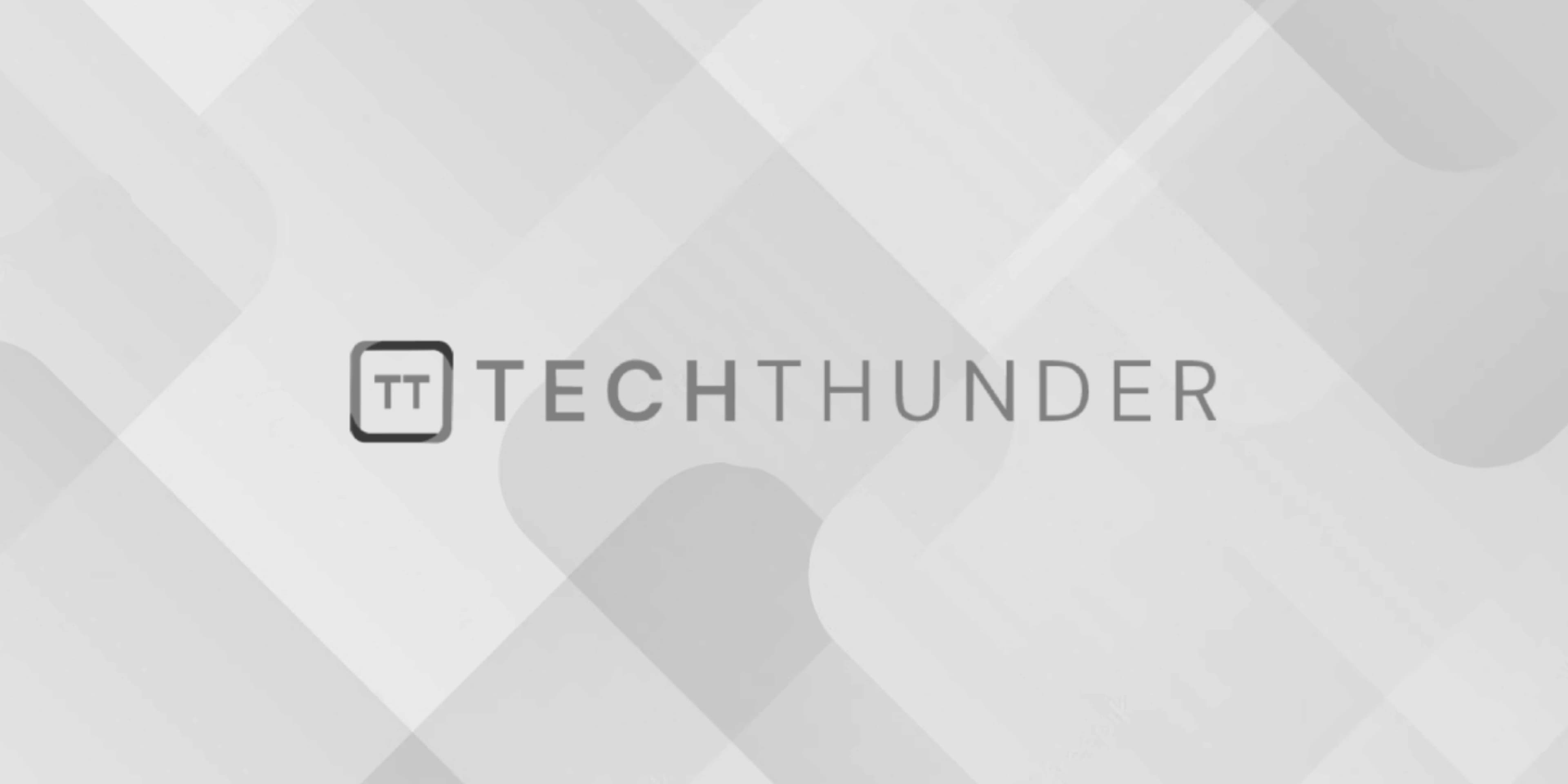
Java Finally Block
The finally
block in java is used in conjunction with try
–catch
blocks to provide a section of code that is executed regardless of whether an exception is thrown or not. The finally
block ensures that certain cleanup or resource-releasing operations are performed, making it useful for scenarios where you need to ensure certain actions are taken, regardless of the success or failure of the code within the try
block.
Here’s the basic structure of a try
–catch
block with a finally
block:
try {
// Code that may throw exceptions
} catch (ExceptionType ex) {
// Code to handle the exception
} finally {
// Code that will be executed regardless of whether an exception was thrown
}
Key points to remember about the finally
block:
- The
finally
block follows all thecatch
blocks (if any) in thetry
–catch
structure. - The code within the
finally
block is executed regardless of whether an exception is thrown or caught. It provides a way to ensure that certain actions, like resource cleanup, are taken even if exceptions occur. - The
finally
block is typically used for tasks that should be performed no matter what, such as releasing resources like file handles, closing database connections, or cleaning up memory.
Here’s an example demonstrating the use of the finally
block:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FinallyBlockExample {
public static void main(String[] args) {
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader("myfile.txt"));
String line = reader.readLine();
System.out.println("Read line: " + line);
} catch (IOException ex) {
System.out.println("Error reading the file: " + ex.getMessage());
} finally {
// Always close the reader, even if an exception occurred
if (reader != null) {
try {
reader.close();
} catch (IOException ex) {
System.out.println("Error closing the reader: " + ex.getMessage());
}
}
}
}
}
In this example, the BufferedReader
is opened to read a file, and the finally
block ensures that the reader is closed even if an exception is thrown during the reading process. This helps prevent resource leaks and ensures that the code behaves correctly under different scenarios.