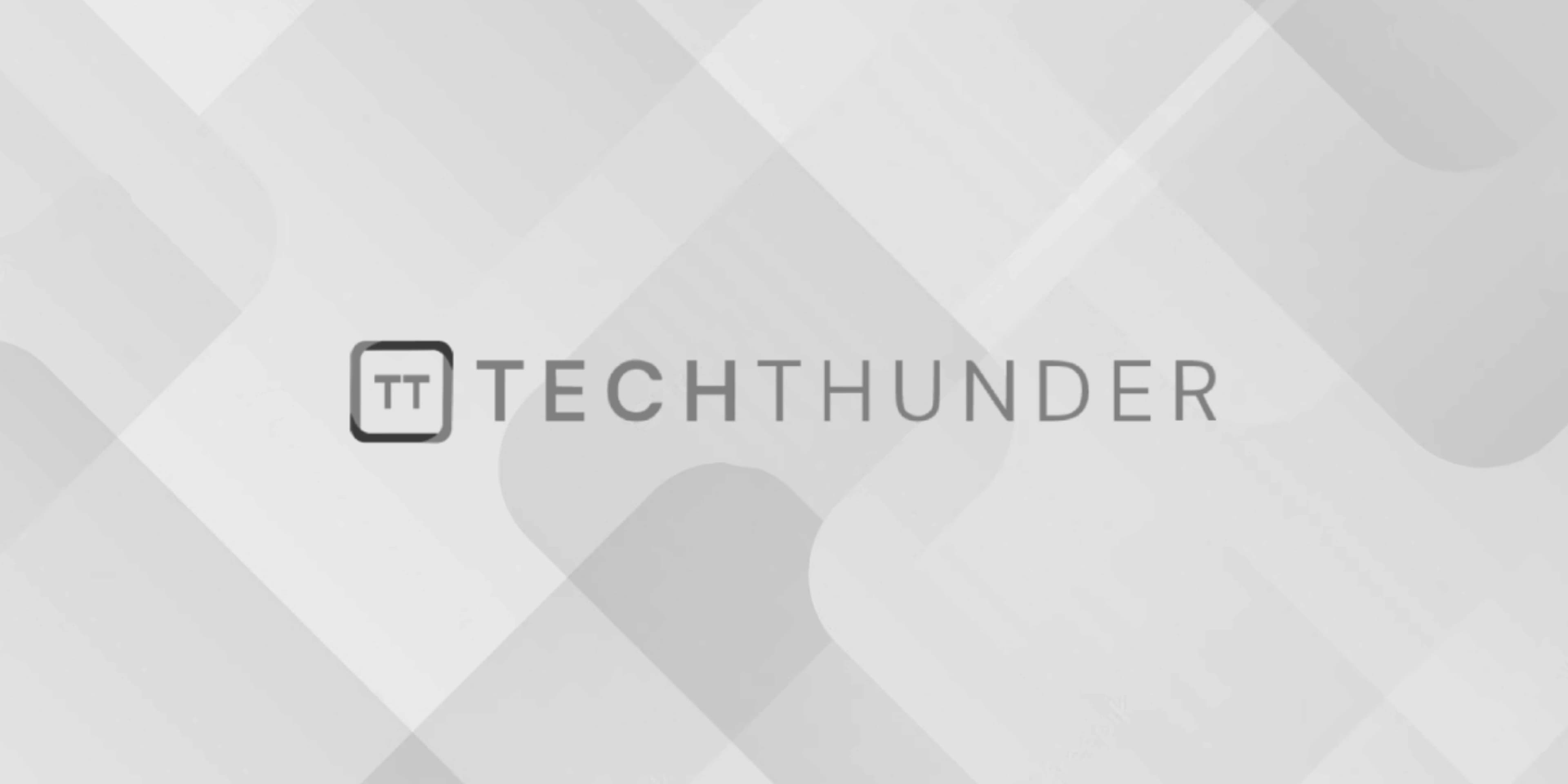
188 views
Java Throw vs Throws
The throw
and throws
are two related but distinct keywords in Java that are used in the context of exceptions. Here’s a breakdown of their differences:
throw
:
throw
is used to explicitly throw an exception within a method.- It is used when you want to create and throw an instance of an exception class, signaling that an exceptional condition has occurred.
- You use it followed by an instance of an exception class:
throw new SomeException();
. - It is used within the body of a method to indicate a specific exceptional scenario.
- Example:
public void someMethod(int value) { if (value < 0) { throw new IllegalArgumentException("Value must be positive"); } // Rest of the method's logic }
throws
:
throws
is used in a method signature to declare that the method may throw certain types of exceptions.- It is used to indicate to the caller that the method could potentially encounter exceptions of the specified types and that the caller needs to handle or propagate those exceptions.
- You use it followed by the names of exception classes:
public void someMethod() throws SomeException, AnotherException { ... }
. - It is used in the method declaration to inform the caller about the possible exceptions that need to be handled.
- Example:
public void readFile(String filename) throws IOException { // Code to read a file }
In summary, throw
is used within a method to actually throw an exception when a certain condition is met, whereas throws
is used in the method declaration to indicate the types of exceptions that the method might throw, requiring callers to handle or propagate those exceptions.