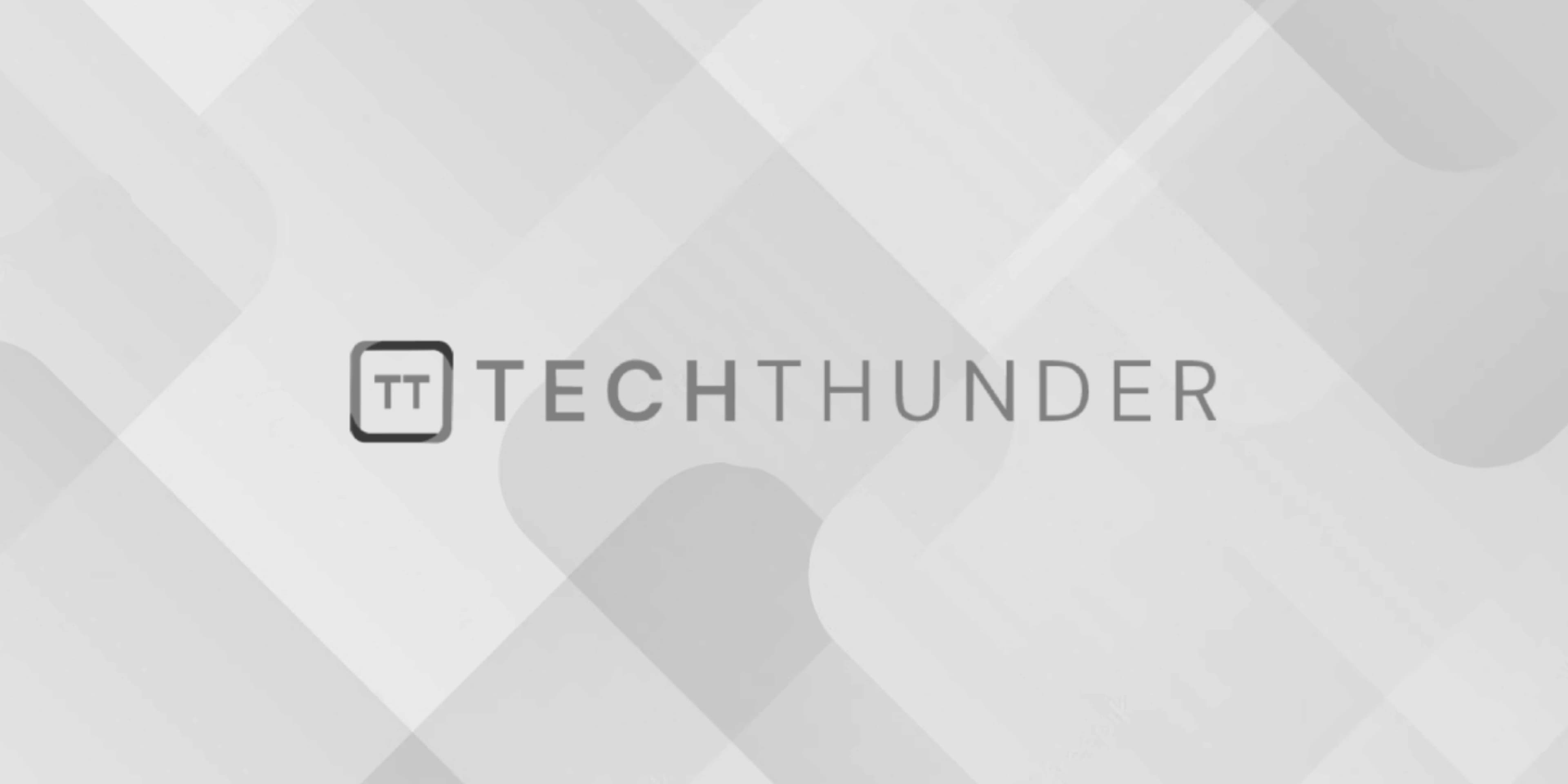
Java Throws Keyword
The throws
keyword in Java is used to declare that a method may throw one or more types of exceptions. This declaration is part of the method signature and informs the caller of the method that they need to handle or propagate those exceptions when calling the method.
Here’s the syntax for using the throws
keyword in a method signature:
returnType methodName(parameters) throws ExceptionType1, ExceptionType2, ... {
// Method implementation
}
returnType
: The data type of the value that the method returns. It can be any valid Java data type orvoid
if the method doesn’t return anything.methodName
: The name of the method.parameters
: The parameters that the method accepts (if any).ExceptionType1
,ExceptionType2
, etc.: The types of exceptions that the method may throw. These should be classes that extend fromThrowable
, such as built-in exception classes or custom exception classes.
Here’s an example of how the throws
keyword is used:
import java.io.IOException;
public class FileHandler {
public void readFile(String filename) throws IOException {
// Code to read a file
}
}
In this example, the readFile
method may throw an IOException
. By declaring throws IOException
in the method signature, you’re informing the caller that they should be prepared to handle this exception when using the readFile
method.
When you call a method that declares exceptions with the throws
keyword, you need to either catch those exceptions using a try
–catch
block or propagate them further using the throws
keyword in the calling method’s signature.
public class Main {
public static void main(String[] args) {
FileHandler handler = new FileHandler();
try {
handler.readFile("example.txt");
} catch (IOException e) {
System.out.println("An error occurred while reading the file: " + e.getMessage());
}
}
}
In this example, the readFile
method is called, and since it declares throws IOException
, the calling code wraps the method call within a try
–catch
block to handle the potential IOException
.
The throws
keyword helps in creating a clear contract between the method and its callers, indicating which exceptions may be thrown by the method and allowing for proper handling and propagation of exceptions throughout your code.