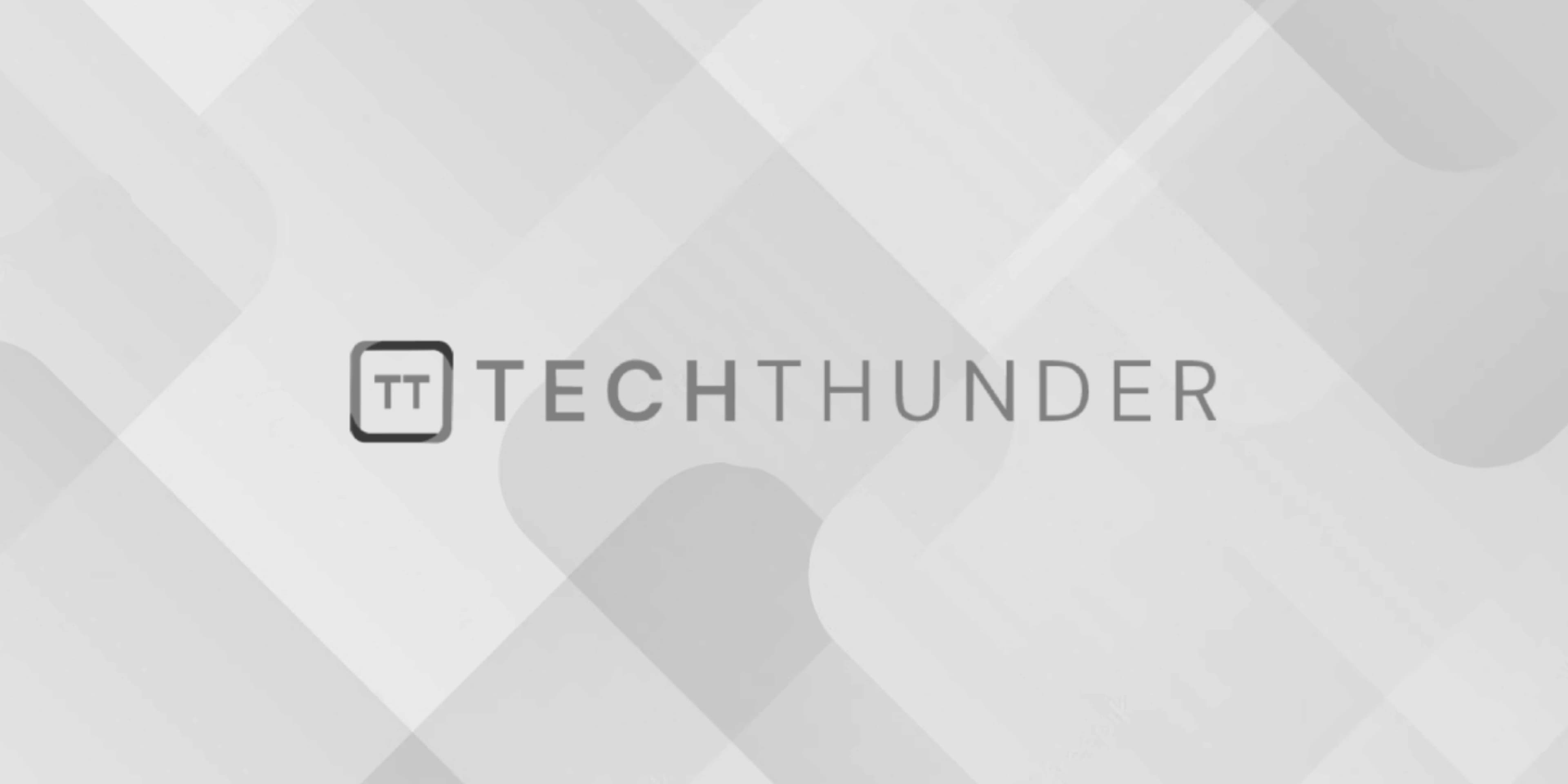
168 views
Java Try-catch block
The try
–catch
block in Java is a fundamental construct used for exception handling. It allows you to write code that can potentially throw exceptions and then provide specific error-handling code to deal with those exceptions gracefully. Here’s how the try
–catch
block works:
try
Block:
- The
try
block encloses the code that might throw an exception. - The code within the
try
block is monitored for exceptions. - If an exception occurs within the
try
block, the normal flow of execution is immediately interrupted, and control is transferred to the appropriatecatch
block.
catch
Block:
- The
catch
block follows thetry
block and specifies the type of exception it can catch. - If the exception thrown within the
try
block matches the type specified in thecatch
block, the code within thecatch
block is executed. - The
catch
block can access the caught exception using the exception parameter, allowing you to perform error-specific actions or logging.
- Example:
try {
// Code that may throw an exception
} catch (SomeException ex) {
// Code to handle SomeException
}
finally
Block:
- Optionally, you can use a
finally
block after thecatch
block. - The code within the
finally
block is executed regardless of whether an exception was thrown or not. - It’s often used for cleanup operations that need to occur regardless of the exception situation.
- Example with
finally
:
try {
// Code that may throw an exception
} catch (SomeException ex) {
// Code to handle SomeException
} finally {
// Cleanup or finalization code
}
Here’s a complete example illustrating the use of try
–catch
blocks:
public class ExceptionExample {
public static void main(String[] args) {
try {
int numerator = 10;
int denominator = 0;
int result = numerator / denominator; // This will throw an ArithmeticException
System.out.println("Result: " + result); // This line won't be reached
} catch (ArithmeticException ex) {
System.out.println("Error: " + ex.getMessage());
} finally {
System.out.println("Cleanup code here"); // This will be executed regardless of exception
}
System.out.println("Program continues"); // This line will be reached
}
}
In this example, a division by zero operation will throw an ArithmeticException
. The catch
block catches this exception and prints an error message. The finally
block is used for cleanup. Even though an exception occurred, the program continues to execute after the try
–catch
block.