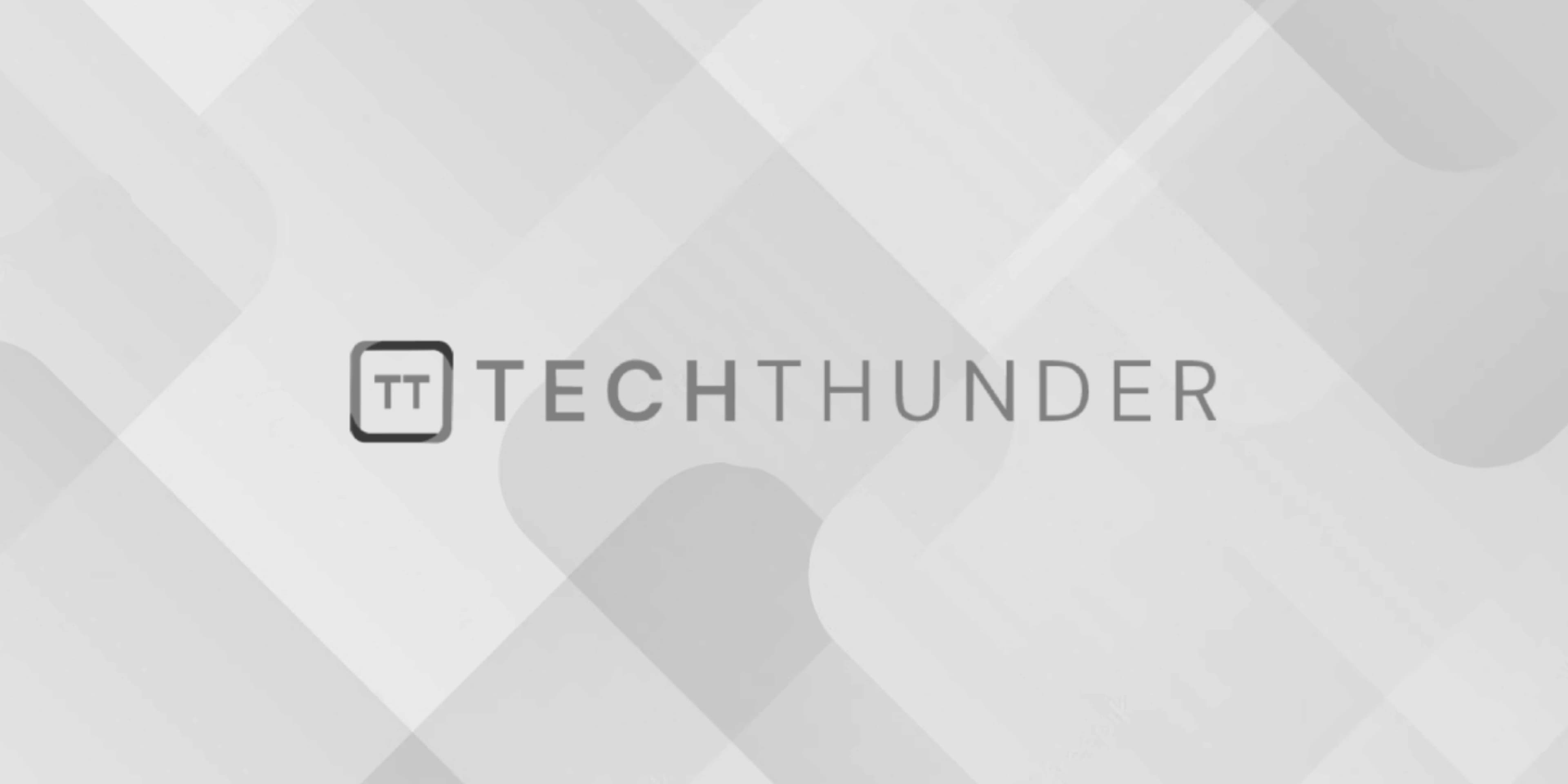
221 views
Java Exceptions
The exceptions in Java are a mechanism for handling runtime errors and abnormal conditions that can occur during the execution of a program. Exceptions provide a way to gracefully handle errors and failures, making your code more robust and maintainable. Java divides exceptions into two main categories: checked exceptions and unchecked exceptions.
- Checked Exceptions:
- Checked exceptions are exceptions that the compiler requires you to handle or declare using the
throws
keyword in the method signature. - These exceptions are typically related to situations where the program can reasonably anticipate and recover from the error.
- Examples of checked exceptions include
IOException
,SQLException
, andClassNotFoundException
.
- Unchecked Exceptions:
- Unchecked exceptions (also known as runtime exceptions) are exceptions that the compiler does not force you to handle or declare.
- These exceptions usually result from programming errors like invalid array indices, null references, or improper type casting.
- Examples of unchecked exceptions include
NullPointerException
,ArrayIndexOutOfBoundsException
, andIllegalArgumentException
.
Here’s a basic overview of how exceptions are used in Java:
- Throwing Exceptions:
Use thethrow
keyword to explicitly throw an exception when an exceptional situation occurs within a method.
if (condition) {
throw new SomeException("This is an exceptional situation");
}
- Catching Exceptions:
Use thetry
–catch
block to catch exceptions and handle them gracefully. Thecatch
block contains code that’s executed when an exception of the specified type occurs.
try {
// Code that may throw an exception
} catch (SomeException ex) {
// Handle the exception
}
- Finally Block:
You can use thefinally
block to provide code that executes regardless of whether an exception was thrown. This block is often used for cleanup operations.
try {
// Code that may throw an exception
} catch (SomeException ex) {
// Handle the exception
} finally {
// Cleanup or finalization code
}
- Declaring Exceptions:
When a method can throw checked exceptions, you need to declare them using thethrows
keyword in the method signature.
public void readFile(String filename) throws IOException {
// Code to read a file
}
- Creating Custom Exceptions:
You can create your own custom exception classes by extending existing exception classes or implementing theThrowable
interface.
public class CustomException extends Exception {
// Custom exception code
}
By effectively using exceptions in your Java code, you can enhance the robustness and maintainability of your programs, making them better equipped to handle various error scenarios.