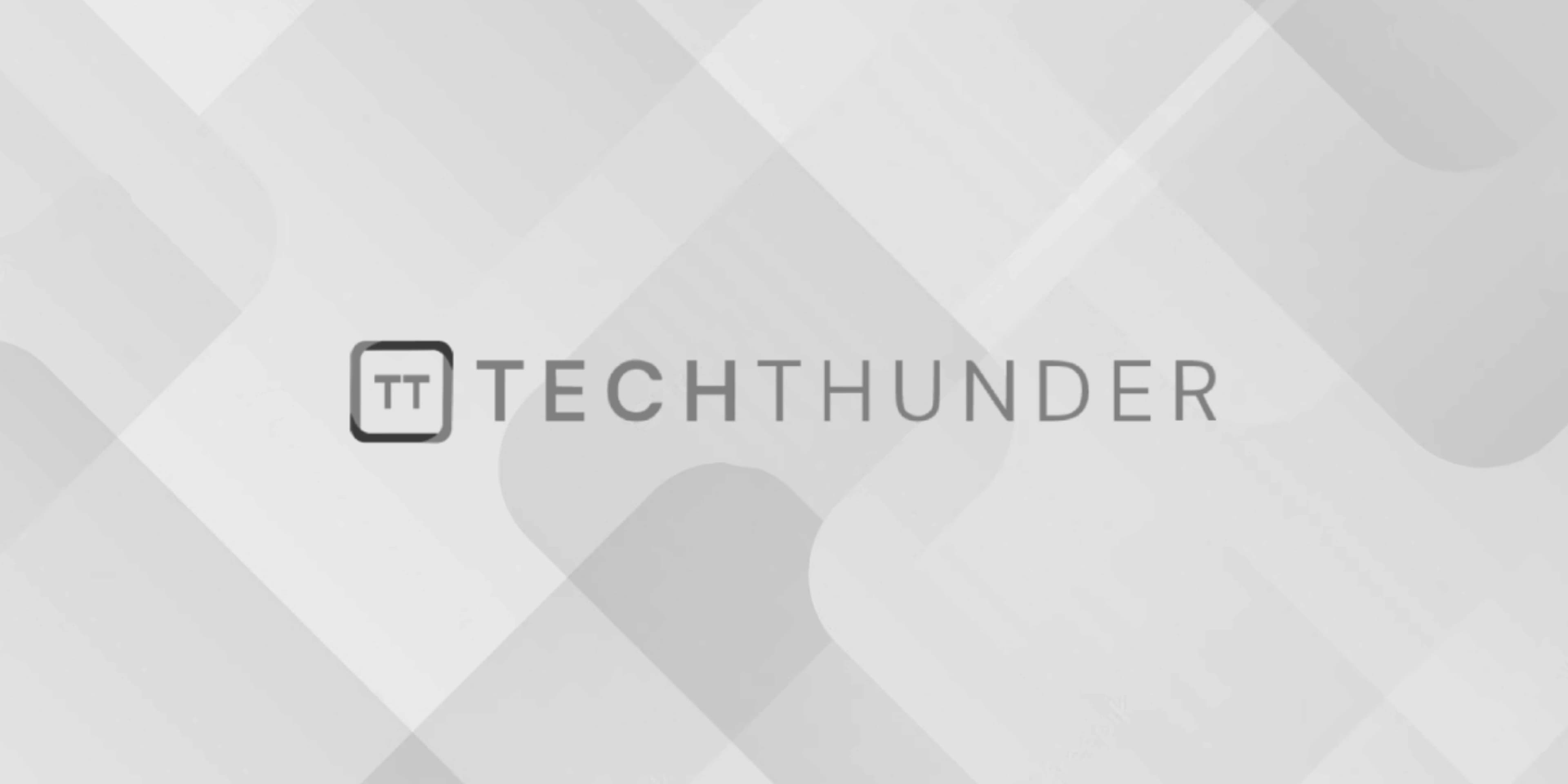
222 views
Java Exception Handling with Method Overriding
Exception handling in Java with method overriding involves understanding how exceptions are propagated and handled when a subclass overrides a method declared in its superclass. Here are the key points to consider when dealing with exception handling and method overriding:
- Method Overriding and Exception Types:
- When a subclass overrides a method from its superclass, the subclass method can throw the same exceptions as the overridden method or subclasses of those exceptions (covariant return types).
- The subclass method is allowed to throw fewer exceptions than the overridden method (or no exceptions at all). However, it cannot throw broader exceptions that are not in the same exception hierarchy.
- Checked Exceptions:
- If the overridden method throws a checked exception, the overriding method is allowed to throw the same exception or a subclass of it, or no exception at all.
- If the overriding method throws a broader checked exception or a new checked exception that the overridden method does not throw, a compilation error will occur.
- Unchecked Exceptions (Runtime Exceptions):
- The overriding method can throw any unchecked exception (runtime exception) without any restrictions, even if the overridden method does not declare the same exception.
- Example:
class Superclass {
void method() throws Exception {
// Some code that throws an exception
}
}
class Subclass extends Superclass {
// It can throw the same exception or a subclass of it, or no exception
@Override
void method() throws SubclassException {
// Overridden method implementation
}
}
class SubclassException extends Exception {
// Custom exception
}
- Method Invocation and Exception Handling:
- When you invoke an overridden method using a reference of the superclass, the compiler checks the exception declarations based on the reference type (superclass) rather than the actual object type (subclass).
- If the overridden method in the subclass throws an exception that is not allowed by the reference type, you need to catch or declare that exception.
- Example:
public class Main {
public static void main(String[] args) {
Superclass instance = new Subclass();
try {
instance.method(); // Compiler checks exception based on Superclass type
} catch (SubclassException e) {
// Handle SubclassException
}
}
}
In summary, when dealing with method overriding and exception handling, subclasses can throw the same exceptions or subclasses of those exceptions as the overridden methods, following the rules of exception hierarchy and checked/unchecked exceptions. When invoking overridden methods, the compiler checks the exception compatibility based on the reference type of the object, which affects the need for catching or declaring exceptions.