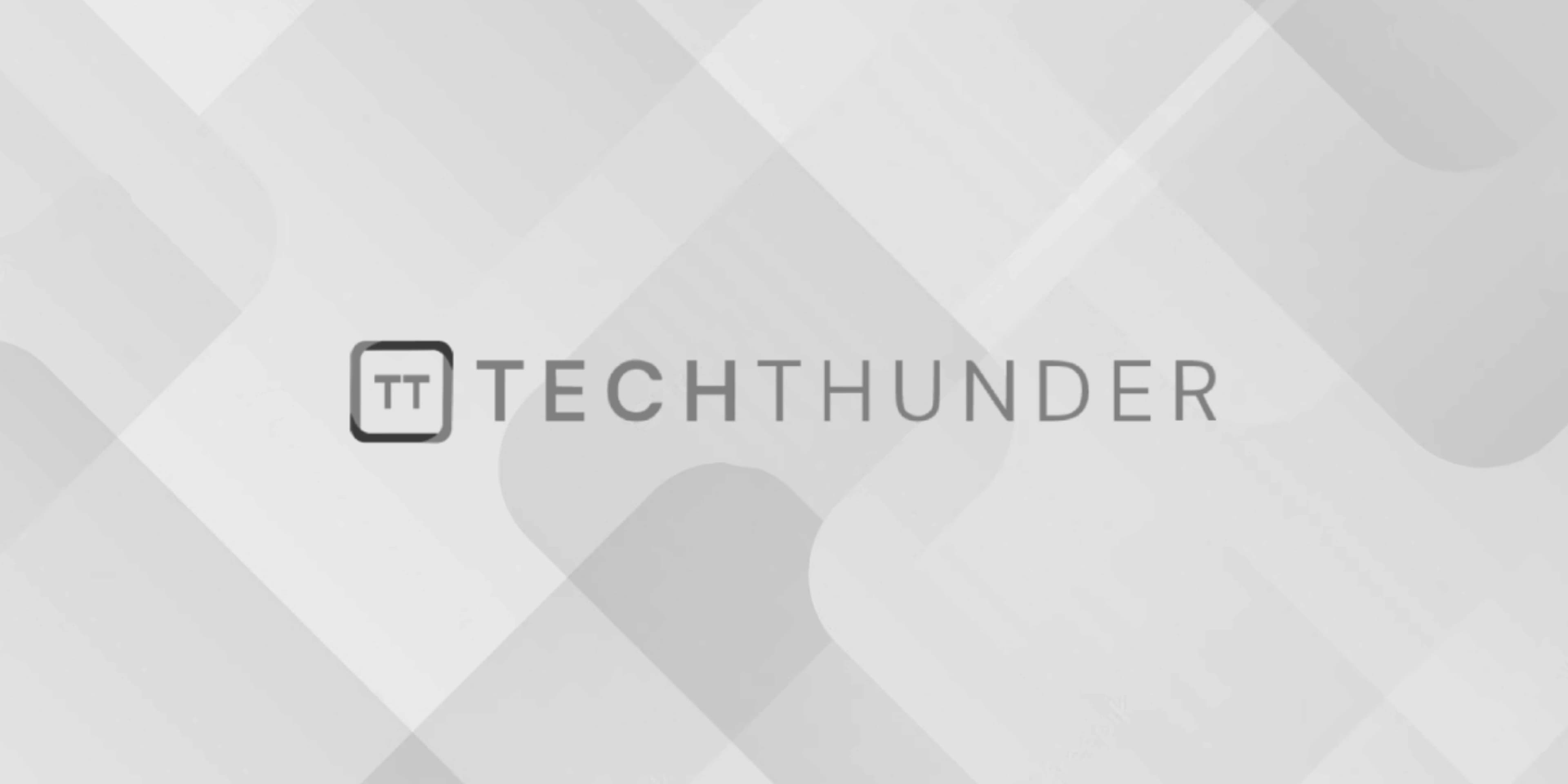
Java Nested try
The nested try
blocks to handle exceptions in a hierarchical manner. This means that you can have an outer try
block that encompasses one or more inner try
blocks. Each try
block can have its own catch
and finally
blocks, allowing you to handle exceptions at different levels of granularity. Here’s how you can use nested try
blocks:
try {
// Outer try block
// Code that may throw exceptions
try {
// Inner try block
// More code that may throw exceptions
} catch (InnerExceptionType ex1) {
// Handle exceptions specific to the inner try block
} finally {
// Inner finally block
// Cleanup code specific to the inner try block
}
} catch (OuterExceptionType ex2) {
// Handle exceptions specific to the outer try block
} finally {
// Outer finally block
// Cleanup code specific to the outer try block
}
In this structure, the outer try
block encapsulates both the inner try
block and the code that it surrounds. The inner try
block can handle exceptions specific to its enclosed code, and the outer try
block can handle exceptions specific to its enclosed code.
Here’s a concrete example:
public class NestedTryExample {
public static void main(String[] args) {
try {
int[] numbers = new int[3];
numbers[4] = 10; // This will throw an ArrayIndexOutOfBoundsException
try {
String text = null;
int length = text.length(); // This will throw a NullPointerException
} catch (NullPointerException ex1) {
System.out.println("Inner NullPointerException: " + ex1.getMessage());
} finally {
System.out.println("Inner finally block");
}
} catch (ArrayIndexOutOfBoundsException ex2) {
System.out.println("Outer ArrayIndexOutOfBoundsException: " + ex2.getMessage());
} finally {
System.out.println("Outer finally block");
}
System.out.println("Program continues"); // This line will be reached
}
}
In this example, the outer try
block catches an ArrayIndexOutOfBoundsException
, and the inner try
block catches a NullPointerException
. The respective catch
blocks and finally
blocks handle the exceptions at different levels of the nested structure. Regardless of the exceptions, the program continues executing after the nested try
–catch
blocks.