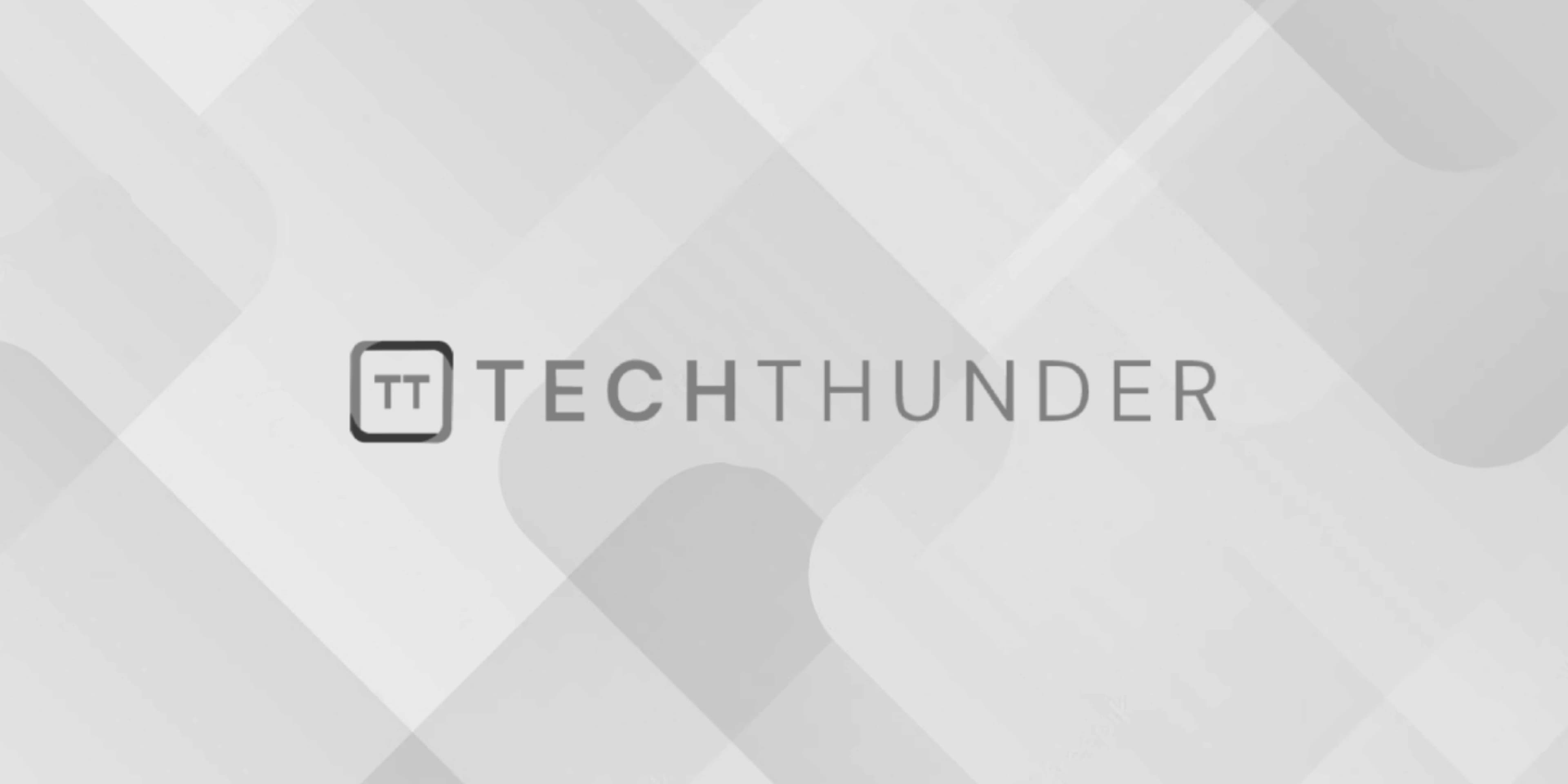
Java Exception Propagation
Exception propagation in Java refers to the process by which exceptions are passed from one method to another, or from one part of the program to another, until they are either caught and handled or the program terminates due to an uncaught exception. This propagation is crucial for maintaining the integrity of the program and ensuring that exceptions are appropriately handled at the appropriate level of code.
Here’s how exception propagation works in Java:
- Throwing Exceptions:
When an exception occurs within a method, you can use thethrow
keyword to create an instance of an exception class and pass it up the call stack. - Method Signatures and
throws
:
If a method can potentially throw a checked exception (exceptions that are subclasses ofException
but notRuntimeException
), you need to declare this in the method’s signature using thethrows
keyword. This informs the calling code that it needs to handle or propagate the exception further.
public void someMethod() throws SomeException {
// ...
}
- Propagation through Call Stack:
When a method throws an exception, the exception travels up the call stack to the nearest enclosingtry
–catch
block that can handle that type of exception. If the exception is not caught within the current method, the control is passed to the caller method and so on, until the exception is caught or the top-level of the program is reached. - Uncaught Exceptions:
If an exception propagates all the way to the top-level of the program (i.e., themain
method) without being caught, the program terminates, and information about the exception is printed to the standard error stream. - Catching Exceptions:
You can catch exceptions usingtry
–catch
blocks. If you catch an exception and handle it within a method, the propagation stops at that point, and the program continues executing from where the exception was caught.
Here’s an example demonstrating exception propagation:
public class ExceptionPropagationExample {
public static void main(String[] args) {
try {
methodA();
} catch (Exception e) {
System.out.println("Caught in main: " + e.getMessage());
}
}
public static void methodA() throws Exception {
methodB();
}
public static void methodB() throws Exception {
methodC();
}
public static void methodC() throws Exception {
throw new Exception("Exception in methodC");
}
}
In this example, an exception is thrown in methodC
. The exception is then propagated up through methodB
, methodA
, and finally caught in the main
method.
Understanding exception propagation is essential for writing robust and error-handling code in Java. It allows you to control how exceptions are handled at various levels of your application, ensuring that your program behaves predictably even when errors occur.