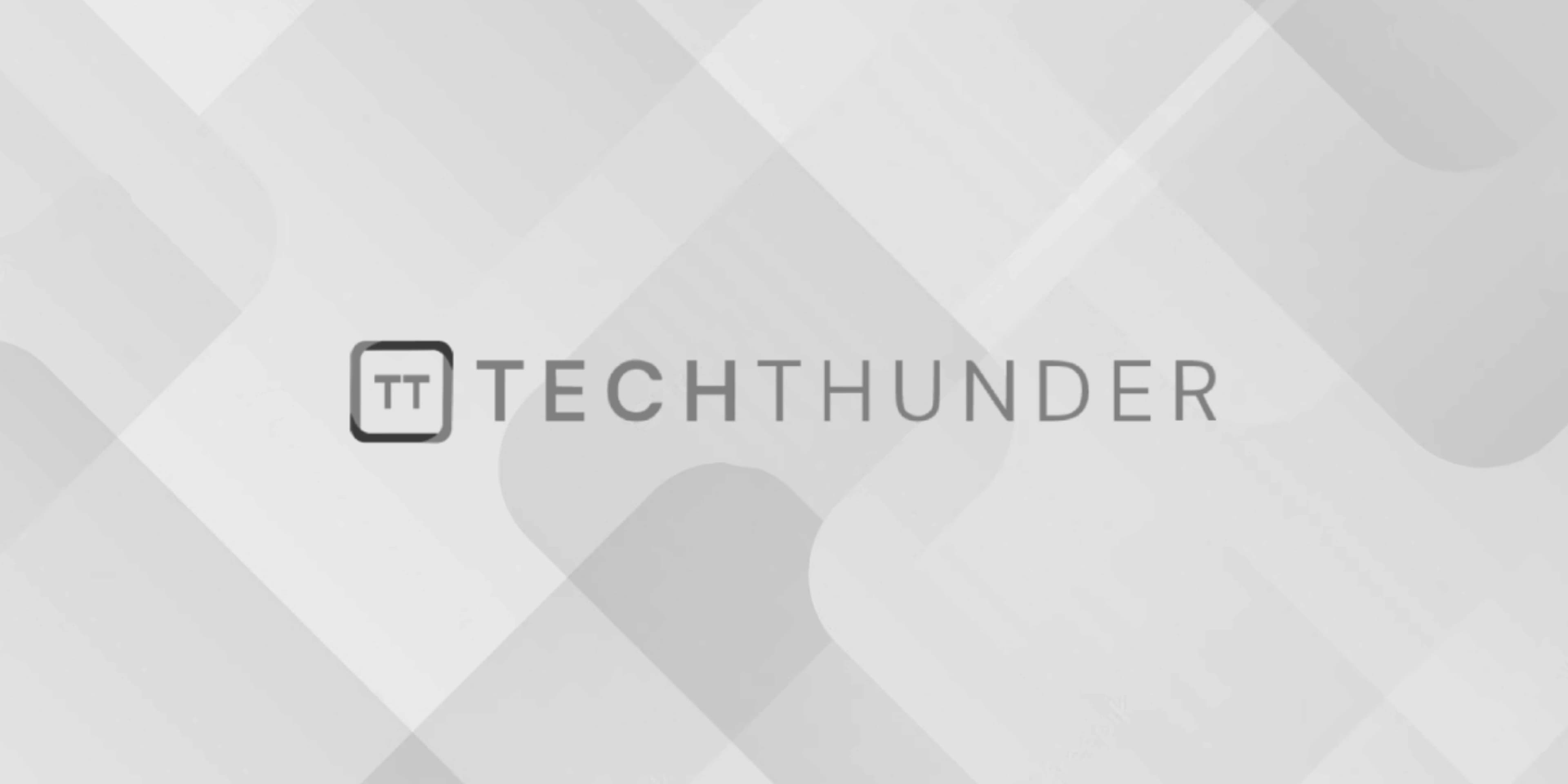
Java Throw Keyword
The throw
keyword in Java is used to explicitly throw an exception within your code. Exceptions are events that occur during the execution of a program that disrupt the normal flow of the program. By throwing an exception, you indicate that a problem or error has occurred, and the program should handle it appropriately.
The syntax for using the throw
keyword is as follows:
throw exception;
Here, exception
is an instance of a class that derives from the Throwable
class. This can be either a built-in exception class like RuntimeException
, IOException
, etc., or a custom exception class that you define by extending Exception
or one of its subclasses.
Here’s a simple example demonstrating how to use the throw
keyword:
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
public class Example {
public static void main(String[] args) {
try {
int num = -5;
if (num < 0) {
throw new CustomException("Negative numbers are not allowed.");
}
System.out.println("Number is: " + num);
} catch (CustomException e) {
System.out.println("Caught an exception: " + e.getMessage());
}
}
}
In this example, the CustomException
class extends the Exception
class, creating a custom exception type. In the main
method of the Example
class, if the value of num
is negative, a CustomException
is thrown. The catch block then catches this exception and prints an error message.
Remember that when you throw an exception, you are interrupting the normal flow of your program, and it’s important to handle exceptions appropriately using try
–catch
blocks or by declaring the exceptions that a method might throw using the throws
clause. This helps maintain the stability and reliability of your program, as well as providing information about errors for debugging purposes.