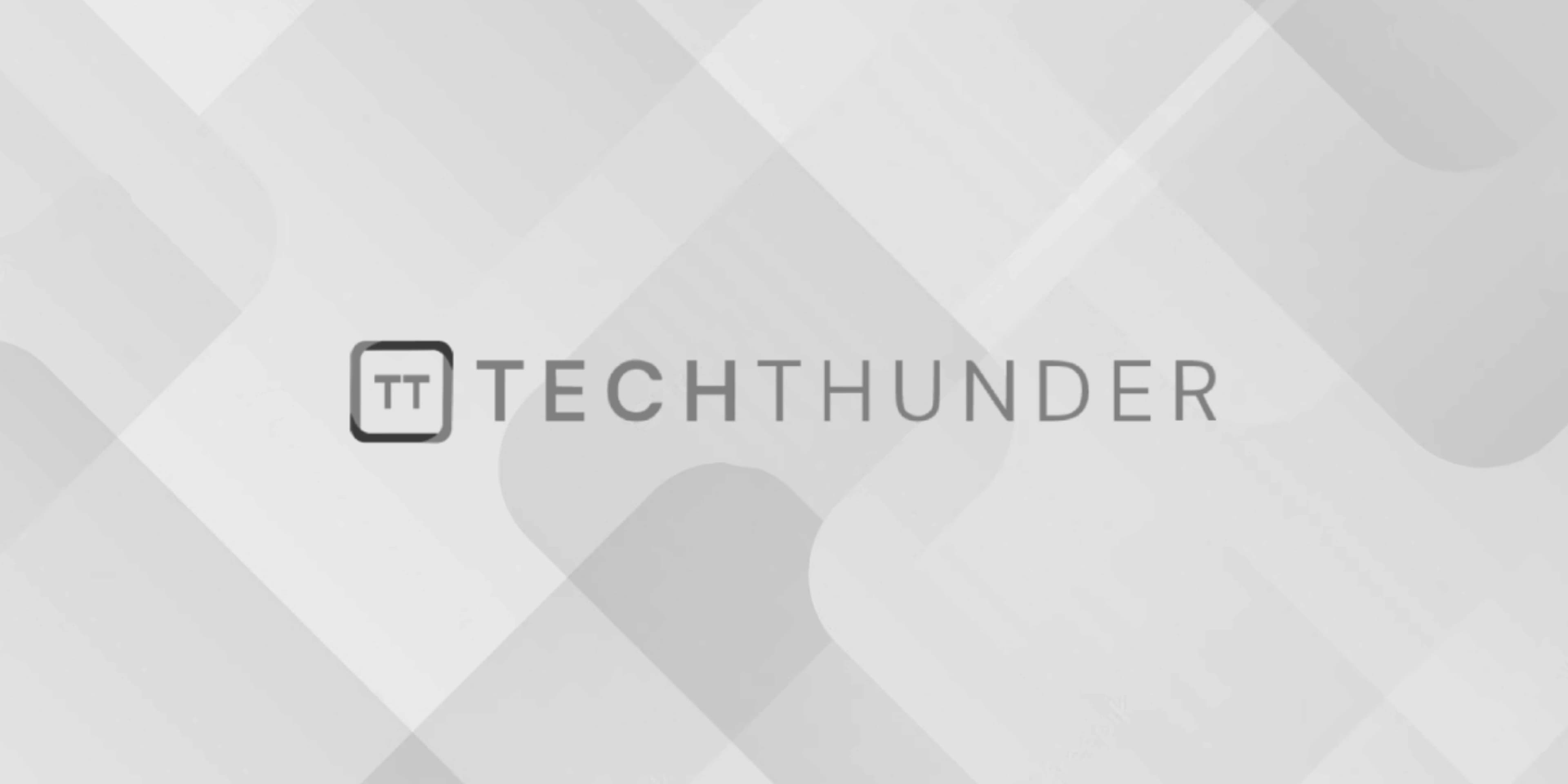
Daemon Thread in java
The daemon thread in java is a type of thread that runs in the background and provides support for other non-daemon threads. The primary difference between daemon threads and non-daemon threads (user threads) is that the Java Virtual Machine (JVM) does not wait for daemon threads to complete before terminating the program. In contrast, the JVM will wait for all non-daemon threads to finish before shutting down.
Here are some key characteristics and uses of daemon threads in Java:
- Daemon Status: You can set a thread as a daemon thread by calling the
setDaemon(true)
method before starting it. By default, threads are non-daemon.
Thread daemonThread = new Thread(new MyRunnable());
daemonThread.setDaemon(true);
daemonThread.start();
- Example Use Cases:
- Daemon threads are commonly used for tasks that should not prevent the JVM from exiting when the main program or other non-daemon threads have completed their work.
- Examples of daemon threads include background services like garbage collection, periodic maintenance tasks, and monitoring tasks.
- Termination Behavior:
- When all non-daemon threads in a Java program have completed, the JVM will terminate, regardless of whether daemon threads are still running.
- If a daemon thread is still executing when the JVM is terminated, it is abruptly stopped, and its
run
method is interrupted.
- Daemon Threads and Resource Cleanup:
- Daemon threads should not be used for tasks that involve critical resource cleanup or data consistency, as they may be abruptly terminated by the JVM.
- Non-daemon threads are typically used for tasks that require proper termination and cleanup procedures.
Here’s a simple example of creating and using a daemon thread:
public class DaemonThreadExample {
public static void main(String[] args) {
Thread daemonThread = new Thread(new DaemonTask());
daemonThread.setDaemon(true);
daemonThread.start();
System.out.println("Main thread is running...");
// Sleep to allow the daemon thread to run for a while
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Main thread is exiting.");
}
}
class DaemonTask implements Runnable {
@Override
public void run() {
while (true) {
System.out.println("Daemon thread is running...");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
The DaemonThreadExample
program starts a daemon thread (DaemonTask
) that prints messages every second. While the main thread is running, the daemon thread continues to execute. When the main thread completes, the JVM exits, and the daemon thread is abruptly terminated.
Daemon threads are a useful feature when you have tasks that should run in the background without preventing your application from gracefully shutting down. However, it’s crucial to be aware of their termination behavior and use them for appropriate tasks.