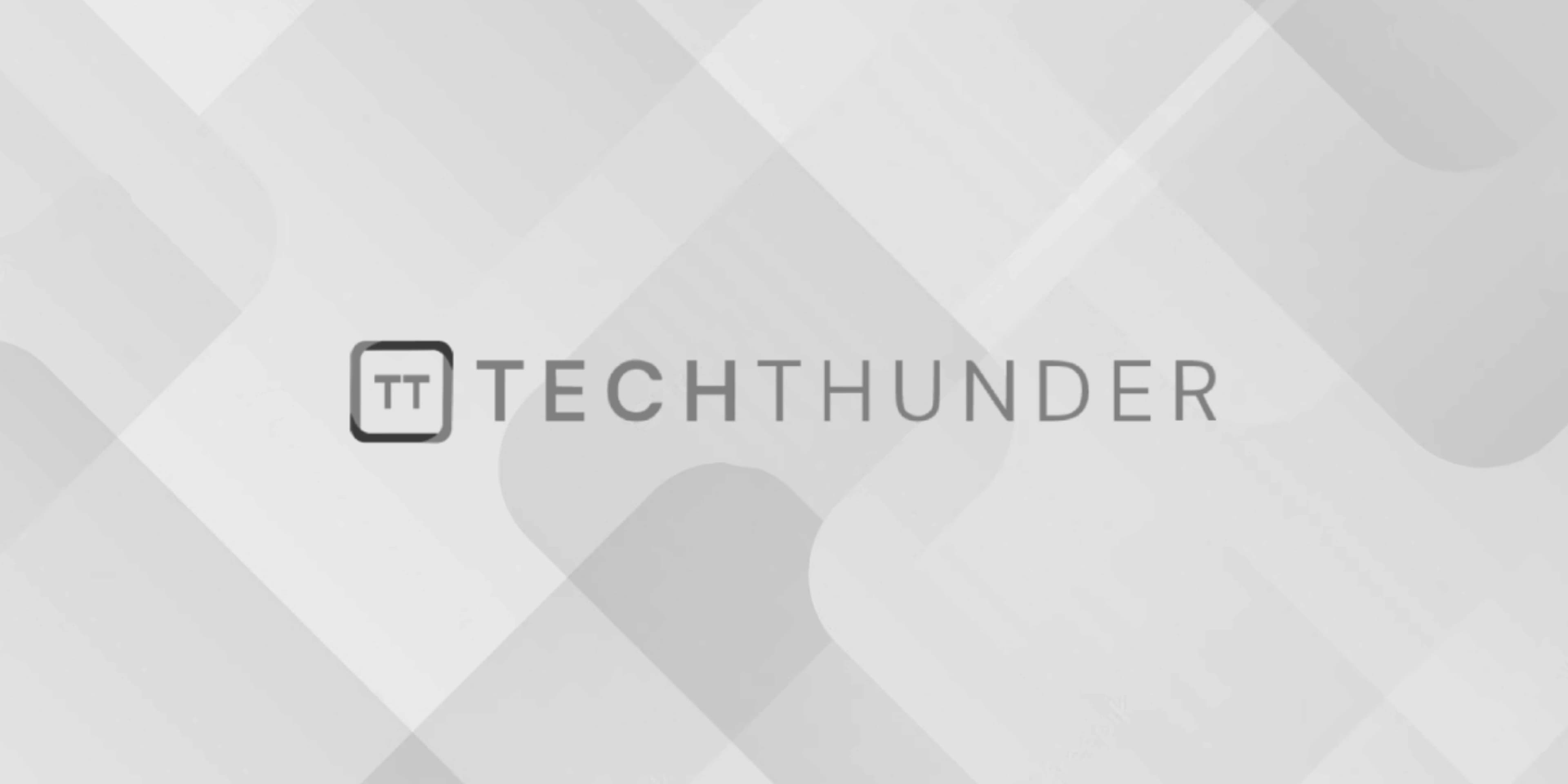
Runtime class in java
In Java, the Runtime
class is a built-in class in the java.lang
package that provides access to the runtime environment in which the Java application is running. It is a singleton class, meaning that only one instance of the Runtime
class exists in a Java Virtual Machine (JVM) instance.
The Runtime
class allows developers to interact with the JVM and operating system to perform tasks such as executing system commands, managing process resources, controlling the garbage collector, and getting information about the available processors and memory.
To obtain an instance of the Runtime
class, you use the static method Runtime.getRuntime()
, which returns the singleton instance of the Runtime
class for the current JVM.
Here are some of the commonly used methods provided by the Runtime
class:
- exec(String command): This method is used to execute a system command as a separate process. It allows Java applications to run external programs or shell commands.
- availableProcessors(): This method returns the number of available processors in the system, which can be useful for designing multithreaded applications.
- totalMemory(): This method returns the total amount of memory available to the JVM.
- freeMemory(): This method returns the amount of free memory available in the JVM.
- gc(): This method is used to explicitly request the JVM to run the garbage collector to reclaim unused memory.
- exit(int status): This method is used to terminate the JVM with the specified exit status. It can be used to exit the Java application programmatically.
Here’s an example demonstrating the use of some of the methods of the Runtime
class:
public class RuntimeExample {
public static void main(String[] args) {
// Get the runtime instance
Runtime runtime = Runtime.getRuntime();
// Execute a system command
try {
Process process = runtime.exec("ls -l");
process.waitFor();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
// Get the number of available processors
int availableProcessors = runtime.availableProcessors();
System.out.println("Available Processors: " + availableProcessors);
// Get memory information
long totalMemory = runtime.totalMemory();
long freeMemory = runtime.freeMemory();
long usedMemory = totalMemory - freeMemory;
System.out.println("Total Memory: " + totalMemory + " bytes");
System.out.println("Free Memory: " + freeMemory + " bytes");
System.out.println("Used Memory: " + usedMemory + " bytes");
// Request garbage collection
runtime.gc();
}
}
In this example, we obtain the instance of the Runtime
class using Runtime.getRuntime()
. We then use the exec()
method to execute the system command “ls -l”, which lists the files and directories in the current directory. The availableProcessors()
method returns the number of available processors, and the totalMemory()
and freeMemory()
methods provide memory-related information.
Keep in mind that not all methods of the Runtime
class may be supported on all platforms, and certain security restrictions may apply to some methods. Additionally, running external commands using exec()
requires proper handling of input and output streams to avoid potential security vulnerabilities.
Overall, the Runtime
class is a useful tool for interacting with the JVM and the operating system, enabling Java applications to perform various system-related tasks.