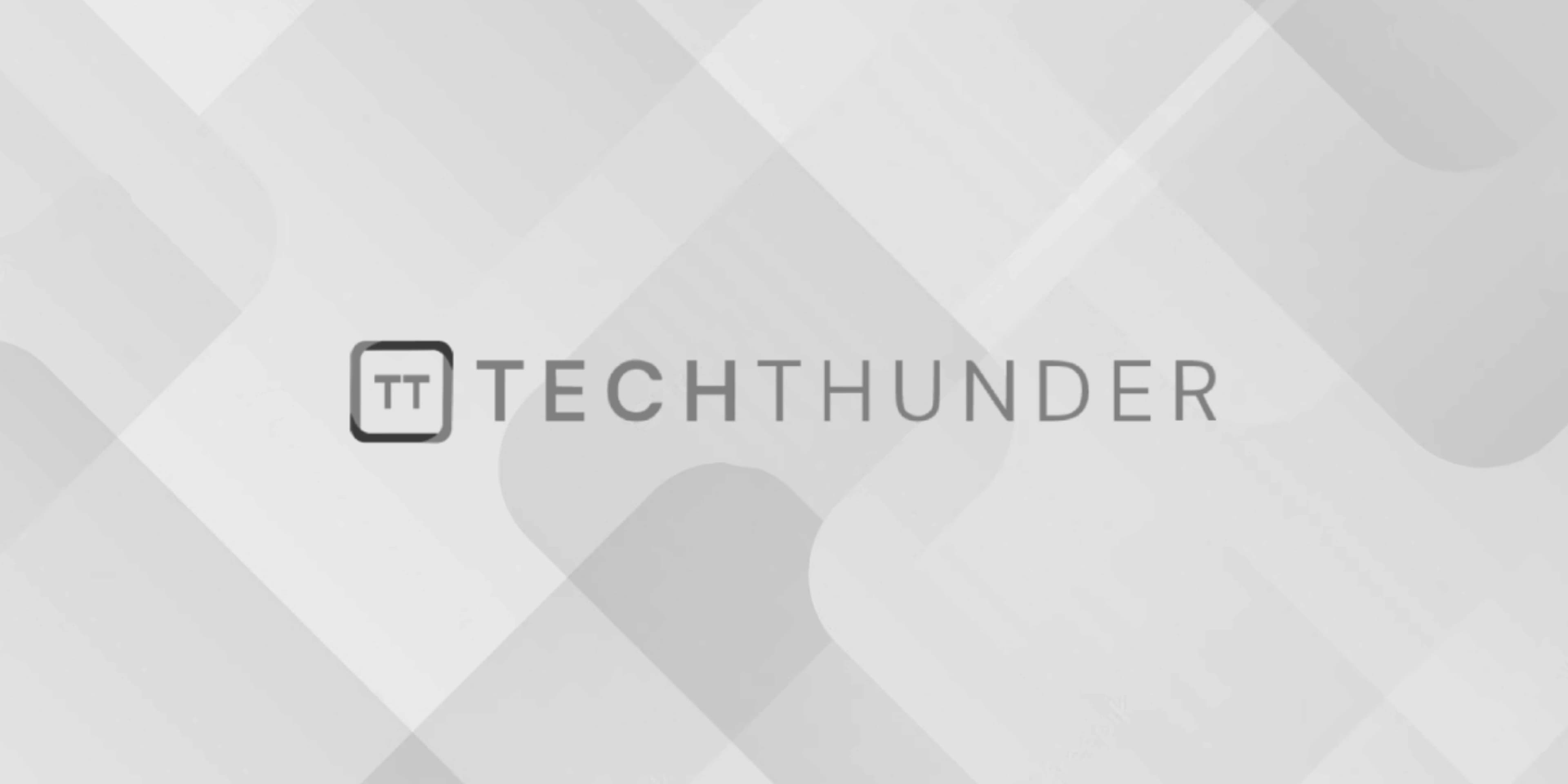
Java Inter-thread Comm
Inter-thread communication in Java refers to the mechanism by which threads can exchange information, coordinate their activities, and synchronize their operations in a multithreaded environment. Proper inter-thread communication is essential for avoiding race conditions, ensuring thread safety, and creating efficient and coordinated multithreaded applications.
Java provides several mechanisms for inter-thread communication, including:
- Object Locks (Synchronized Blocks): Java provides the
synchronized
keyword to create synchronized blocks or methods. By synchronizing on an object, a thread can acquire the object’s monitor lock, allowing it to access shared resources in a thread-safe manner. Other threads attempting to access the synchronized block will be blocked until the lock is released. - Wait and Notify (NotifyAll): Threads can use the
wait()
,notify()
, andnotifyAll()
methods from theObject
class to communicate and coordinate with each other. When a thread callswait()
, it releases the lock on the object and enters a waiting state. Other threads can notify the waiting thread usingnotify()
ornotifyAll()
to wake it up when certain conditions are met. - Java Concurrency Utilities: The
java.util.concurrent
package provides higher-level abstractions and utilities for inter-thread communication. For example,CountDownLatch
,Semaphore
,CyclicBarrier
, andBlockingQueue
can be used to synchronize threads and control their execution. - Thread Joining: The
join()
method allows one thread to wait for the completion of another thread. When a thread callsjoin()
on another thread, it waits until that thread finishes its execution before continuing its own execution. - Thread Signaling: Developers can use custom signaling mechanisms, such as flags or signals shared between threads, to communicate and coordinate their activities.
Here’s a simple example of using inter-thread communication with wait and notify:
public class InterThreadCommunicationExample {
public static void main(String[] args) {
final Message message = new Message();
// Create and start the producer thread
Thread producerThread = new Thread(() -> {
String[] messages = { "Hello", "World", "Bye" };
for (String msg : messages) {
message.putMessage(msg);
}
message.putMessage("exit"); // Signal to terminate the consumer thread
});
// Create and start the consumer thread
Thread consumerThread = new Thread(() -> {
while (true) {
String msg = message.getMessage();
if (msg.equals("exit")) {
break; // Terminate the loop when the exit signal is received
}
System.out.println("Received: " + msg);
}
});
// Start both threads
producerThread.start();
consumerThread.start();
}
}
class Message {
private String message;
private boolean empty = true;
public synchronized void putMessage(String msg) {
while (!empty) {
try {
wait(); // Wait until the consumer consumes the previous message
} catch (InterruptedException e) {
e.printStackTrace();
}
}
this.message = msg;
empty = false;
notify(); // Notify the consumer that a message is available
}
public synchronized String getMessage() {
while (empty) {
try {
wait(); // Wait until a message is produced
} catch (InterruptedException e) {
e.printStackTrace();
}
}
empty = true;
notify(); // Notify the producer that the message has been consumed
return message;
}
}
In this example, we have a simple Message
class that allows a producer thread to put a message, and a consumer thread to retrieve and print the message. The producer and consumer threads communicate and coordinate with each other using the wait()
and notify()
methods.
Inter-thread communication is crucial for designing efficient, coordinated, and thread-safe multithreaded applications in Java. Proper synchronization and communication mechanisms help avoid race conditions and ensure that threads work together harmoniously.