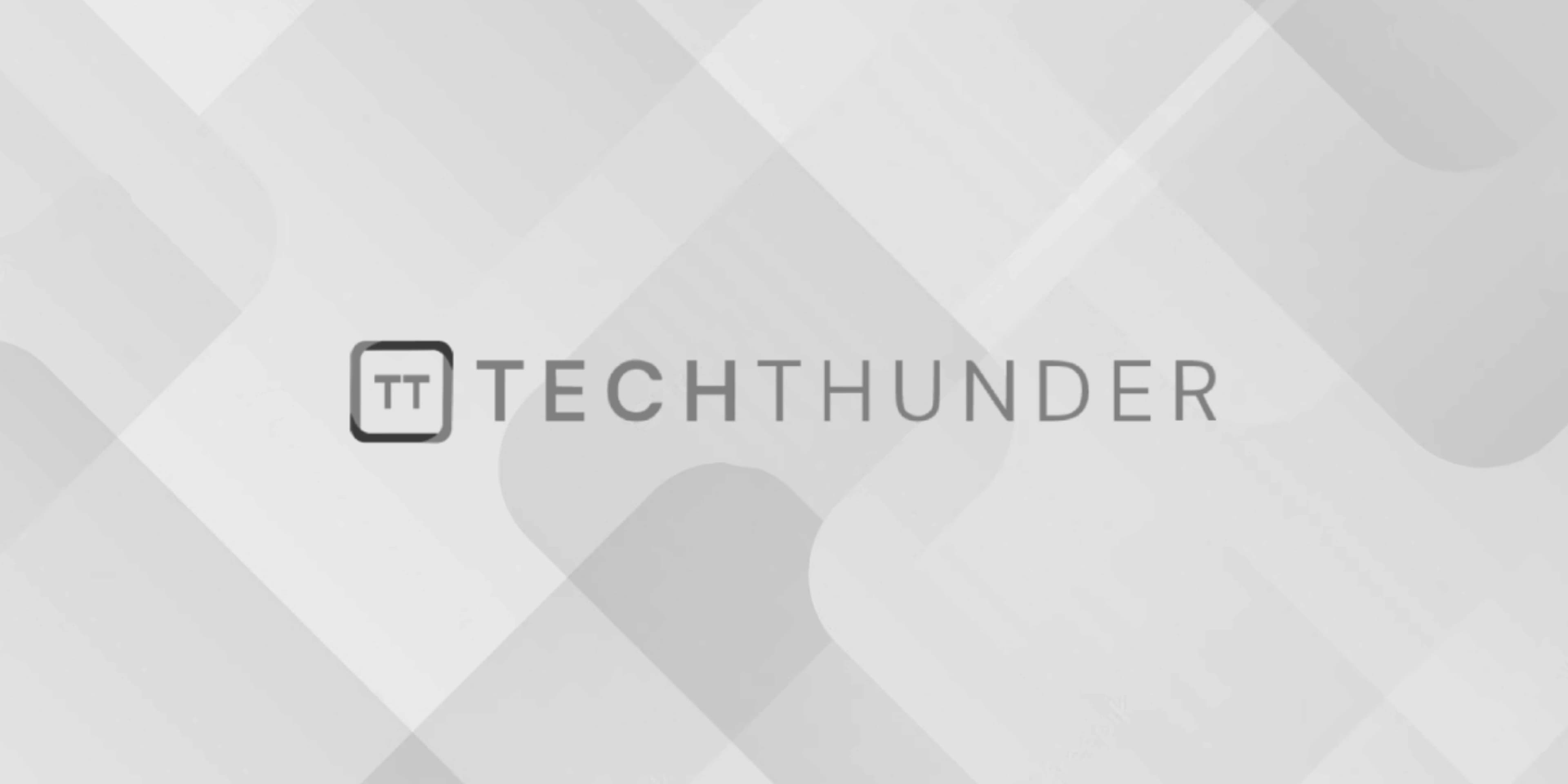
Java Interrupting Thread
In Java, interrupting a thread is a mechanism used to request the termination or interruption of a thread’s execution. When a thread is interrupted, it doesn’t forcefully stop or kill the thread but rather sets a flag on the thread to indicate that it has been interrupted. The interrupted thread can check this flag periodically to determine if it should stop its execution gracefully.
The Thread
class in Java provides methods to manage thread interruption:
- interrupt(): The
interrupt()
method is used to interrupt a thread. When this method is called on a thread, it sets the interrupted flag for that thread. - isInterrupted(): The
isInterrupted()
method is used to check if a thread has been interrupted. It returns a boolean value indicating whether the thread has been interrupted. - Thread.interrupted(): The
Thread.interrupted()
static method is used to check if the current thread has been interrupted. It also clears the interrupted flag for the current thread.
Here’s an example of how to use thread interruption:
public class InterruptExample {
public static void main(String[] args) {
Thread myThread = new Thread(() -> {
try {
while (!Thread.currentThread().isInterrupted()) {
System.out.println("Running...");
Thread.sleep(1000);
}
} catch (InterruptedException e) {
System.out.println("Thread interrupted!");
}
});
myThread.start();
try {
Thread.sleep(5000); // Wait for 5 seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
// Interrupt the thread after 5 seconds
myThread.interrupt();
}
}
In this example, we create a new thread myThread
that prints “Running…” every second until it is interrupted. The thread checks the interrupted flag using isInterrupted()
to determine when to stop its execution.
In the main
method, we start the thread and then sleep the main thread for 5 seconds. After 5 seconds, we call myThread.interrupt()
to interrupt the thread’s execution. The InterruptedException
is caught within the thread, and it prints “Thread interrupted!” before gracefully terminating its execution.
It’s important to note that thread interruption is a cooperative mechanism. It’s up to the thread to check the interrupted flag and decide when and how to stop its execution. In long-running tasks, you can periodically check the interrupted flag using isInterrupted()
to respond to the interrupt request and perform a clean shutdown.
Interrupting threads is a safer and more controlled way of handling thread termination compared to forcefully stopping a thread. It allows the thread to clean up resources, release locks, and gracefully exit, which helps avoid potential data corruption or other issues caused by abrupt termination.