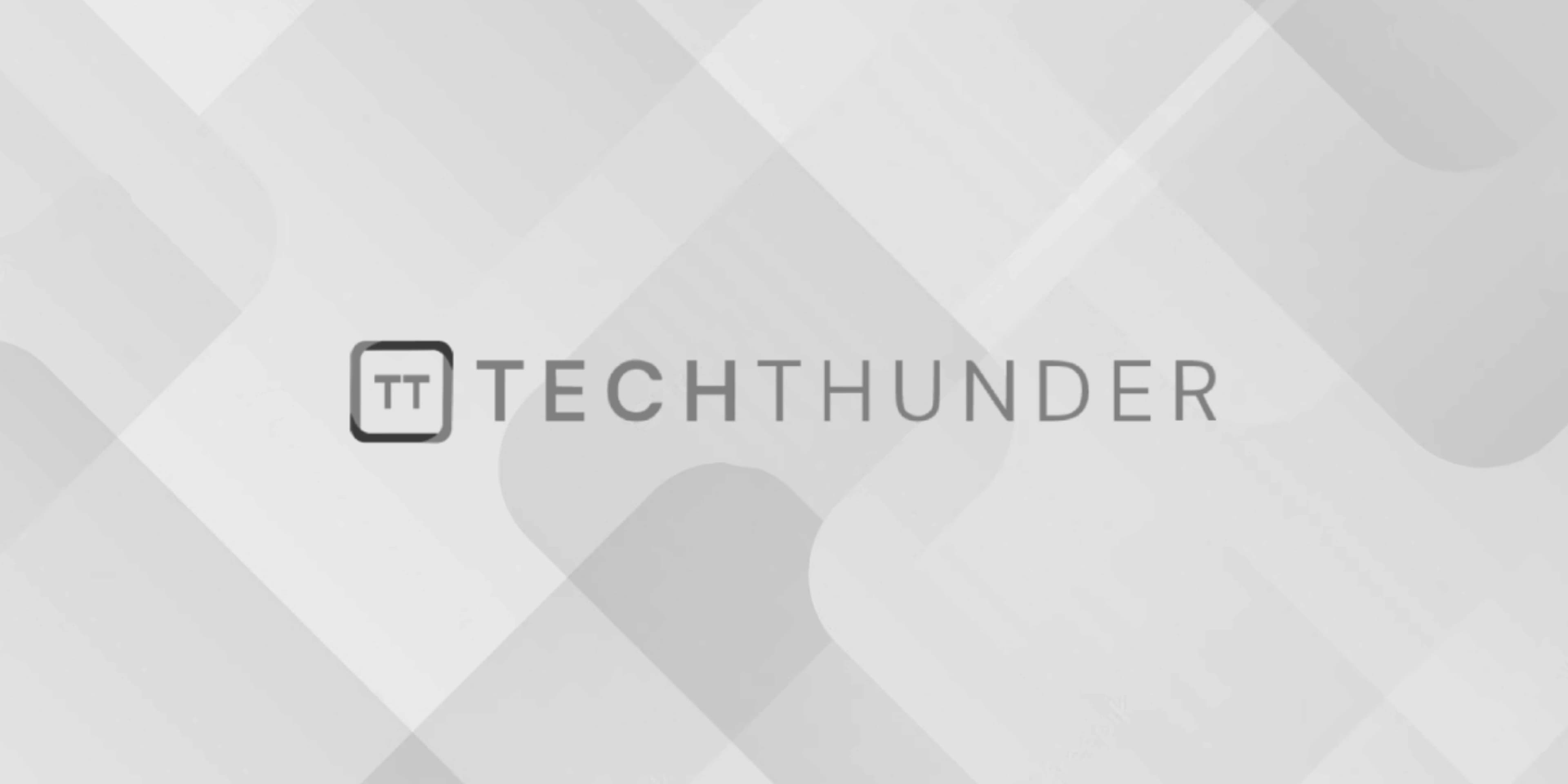
Sleeping thread in java
The Java can make a thread sleep for a specified amount of time using the Thread.sleep()
method. Sleeping a thread temporarily suspends its execution, allowing other threads to run. Here’s how you can use Thread.sleep()
:
public class SleepExample {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
System.out.println("Task " + i + " is in progress...");
try {
// Sleep the current thread for 1 second (1000 milliseconds)
Thread.sleep(1000);
} catch (InterruptedException e) {
// Handle the exception (e.g., if interrupted)
e.printStackTrace();
}
}
System.out.println("All tasks completed.");
}
}
In this example, we have a loop that simulates five tasks. Inside the loop, we use Thread.sleep(1000)
to make the current thread sleep for one second (1000 milliseconds) on each iteration. After the sleep period, the thread continues its execution. The Thread.sleep()
method can throw an InterruptedException
, so it’s a good practice to handle this exception, especially if your thread can be interrupted.
Keep in mind that calling Thread.sleep()
on a thread will pause that specific thread’s execution, allowing other threads to run concurrently if your program is multithreaded. If your program is single-threaded, it will pause the entire program’s execution for the specified duration.
Here are some key points to note:
- The time passed to
Thread.sleep()
is in milliseconds. You can specify the sleep duration as needed. Thread.sleep()
should be used for non-critical, time-based delays (e.g., waiting between periodic tasks), and it’s generally not a precise way to implement time-sensitive operations due to potential variations in system timers.- In modern Java development, it’s often recommended to use higher-level concurrency utilities and thread synchronization mechanisms to manage thread scheduling and coordination more precisely, as these provide better control and robustness in multithreaded applications.