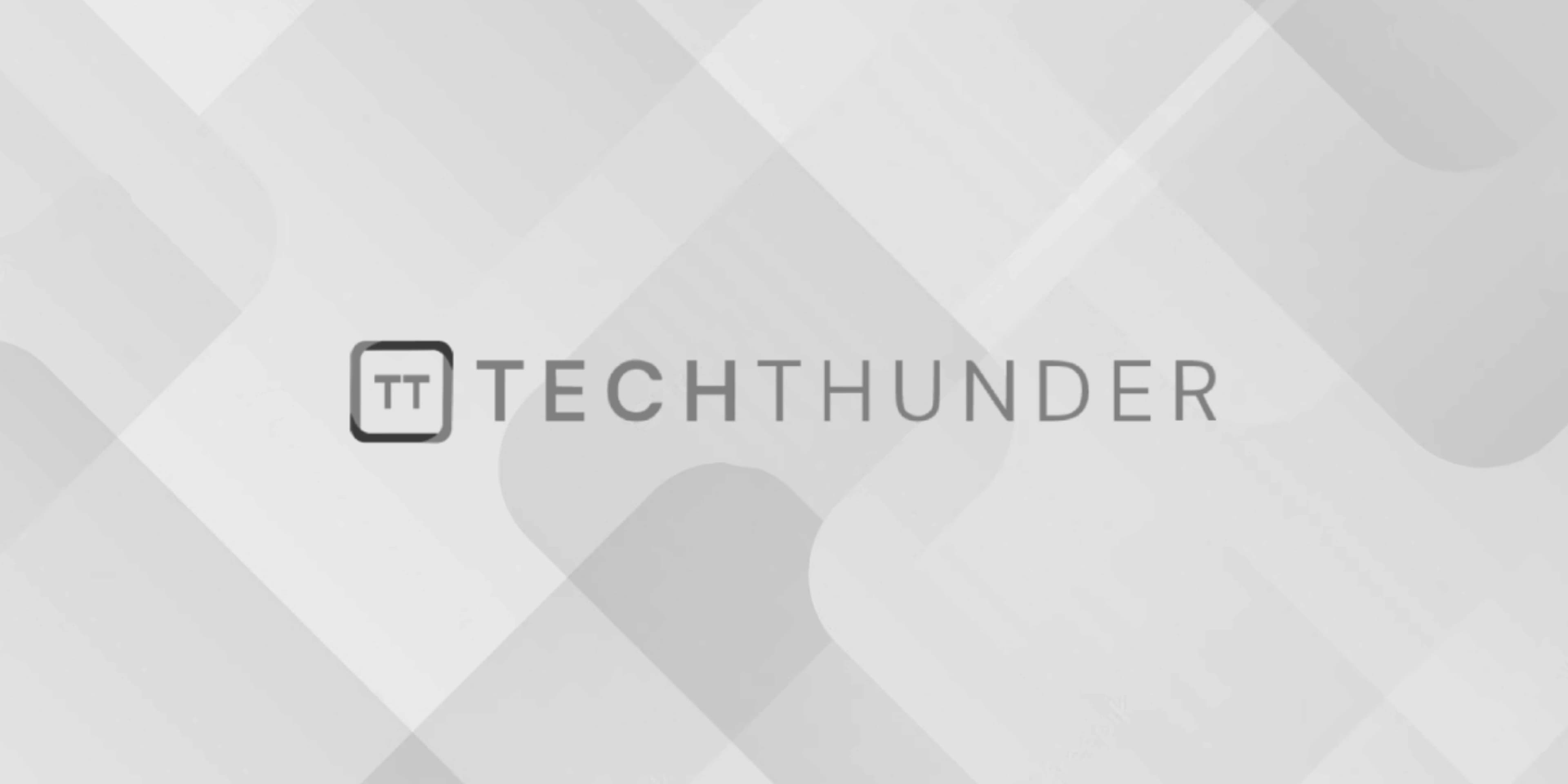
Java synchronized block
The synchronized
keyword in Java is used to create a critical section or a synchronized block of code. It is often used to manage access to shared resources in a multithreaded environment, ensuring that only one thread can execute the synchronized block at a time. This helps prevent race conditions and ensures thread safety.
The basic syntax of a synchronized block is as follows:
synchronized (object) {
// Synchronized code block
// Only one thread can execute this block at a time
}
Here’s a breakdown of how it works:
- You provide an object reference within the parentheses after the
synchronized
keyword. This object is known as the “monitor” or “lock” object. It’s what the threads synchronize on. - When a thread enters the synchronized block, it attempts to acquire the lock associated with the specified object. If the lock is available (no other thread is holding it), the thread will acquire the lock and execute the code inside the synchronized block.
- While a thread is executing the synchronized block, other threads that also attempt to enter the same synchronized block with the same lock object will be blocked. They’ll wait until the lock becomes available.
- Once the executing thread completes the synchronized block, it releases the lock, allowing one of the waiting threads to acquire it and enter the synchronized block.
Here’s a simple example to illustrate the concept:
public class SynchronizedExample {
private int count = 0;
private Object lock = new Object();
public void increment() {
synchronized (lock) {
count++;
}
}
public int getCount() {
synchronized (lock) {
return count;
}
}
}
In this example, the increment
and getCount
methods are synchronized using the lock
object. This ensures that only one thread can either increment the count or read the count at any given time, preventing concurrency issues.
It’s important to note that while synchronized
blocks are a powerful tool for ensuring thread safety, they can also introduce potential performance bottlenecks in highly concurrent applications. In modern Java, other synchronization mechanisms like java.util.concurrent
classes and explicit locks are often used for more fine-grained control over synchronization and improved performance.