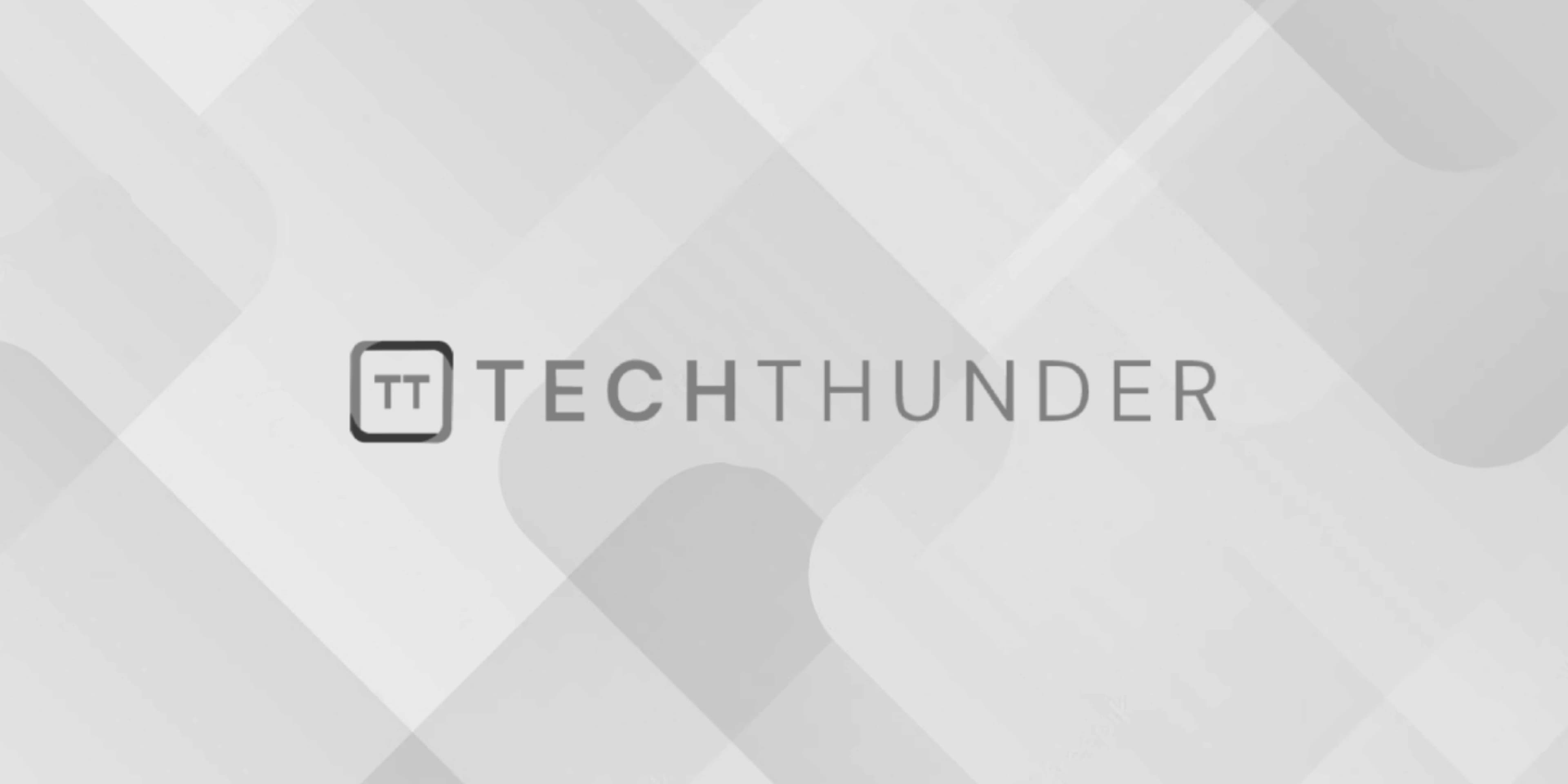
Synchronization in java
Synchronization in Java is a technique used to control access to shared resources or critical sections of code in a multi-threaded environment. When multiple threads access shared resources concurrently, there is a risk of race conditions and data inconsistencies, leading to unpredictable behavior and incorrect results.
Java provides built-in mechanisms for synchronization to ensure that only one thread at a time can access critical sections of code or shared resources. These mechanisms help maintain data integrity, prevent race conditions, and create thread-safe applications.
There are two primary ways to achieve synchronization in Java:
- Synchronized Methods: The
synchronized
keyword is used to declare a method as synchronized. When a thread calls a synchronized method, it acquires the intrinsic lock (also known as the monitor lock) associated with the object on which the method is called. Other threads trying to access the same synchronized method on the same object will be blocked until the lock is released.
public class SynchronizedExample {
private int counter = 0;
public synchronized void increment() {
counter++;
}
}
- Synchronized Blocks: In addition to synchronized methods, you can use synchronized blocks to protect critical sections of code. Synchronized blocks allow more fine-grained control over synchronization compared to synchronized methods.
public class SynchronizedBlockExample {
private Object lock = new Object();
private int counter = 0;
public void increment() {
synchronized (lock) {
counter++;
}
}
}
In both cases, the synchronized
keyword ensures that only one thread can access the critical section of code (i.e., the synchronized method or the code block synchronized on a specific object) at a time.
When should you use synchronization?
- Use synchronization when multiple threads access shared data or resources.
- Use it to protect data integrity and prevent race conditions when updating shared variables.
- Use it when you need to ensure that certain operations are performed atomically, as a single unit of work, without interference from other threads.
While synchronization is essential for creating thread-safe applications, it comes with some overhead, as threads may have to wait for the lock to be released. Overuse of synchronization can lead to contention and reduced performance. Therefore, it’s essential to use synchronization judiciously, only where necessary, and consider other concurrency mechanisms like the java.util.concurrent
package for more complex scenarios.
In summary, synchronization in Java is a vital tool for controlling access to shared resources in multi-threaded applications. Properly synchronized code ensures data integrity and avoids concurrency-related issues, leading to reliable and predictable behavior in concurrent environments.