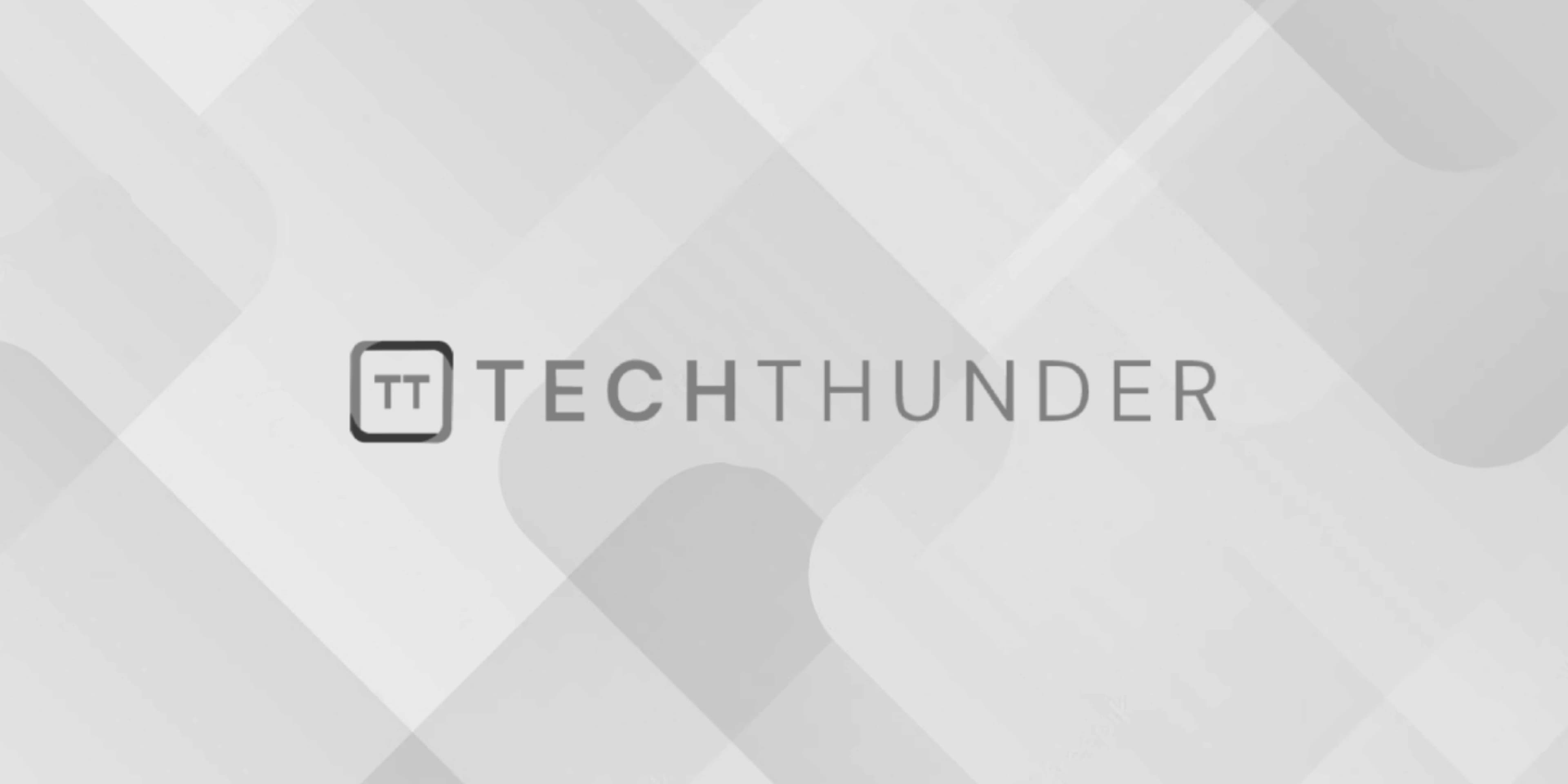
Start thread twice in java
The Java, you cannot start a thread more than once using the start()
method. Once a thread has started and finished its execution (either by completing its task or encountering an unhandled exception), it cannot be restarted. Attempting to restart a thread that has already been started will result in an IllegalThreadStateException
.
If you want to perform a task multiple times using a thread, you can create a new thread instance for each execution. Here’s an example of how you can create and start a new thread for each execution of a task:
public class MyThread extends Thread {
@Override
public void run() {
System.out.println("Thread is running...");
}
public static void main(String[] args) {
for (int i = 0; i < 2; i++) {
MyThread thread = new MyThread(); // Create a new thread instance
thread.start(); // Start the new thread
}
}
}
In this example, we create a new MyThread
instance for each execution of the task in a loop, and we start each thread separately. This allows you to perform the task multiple times by creating and starting a new thread for each execution.