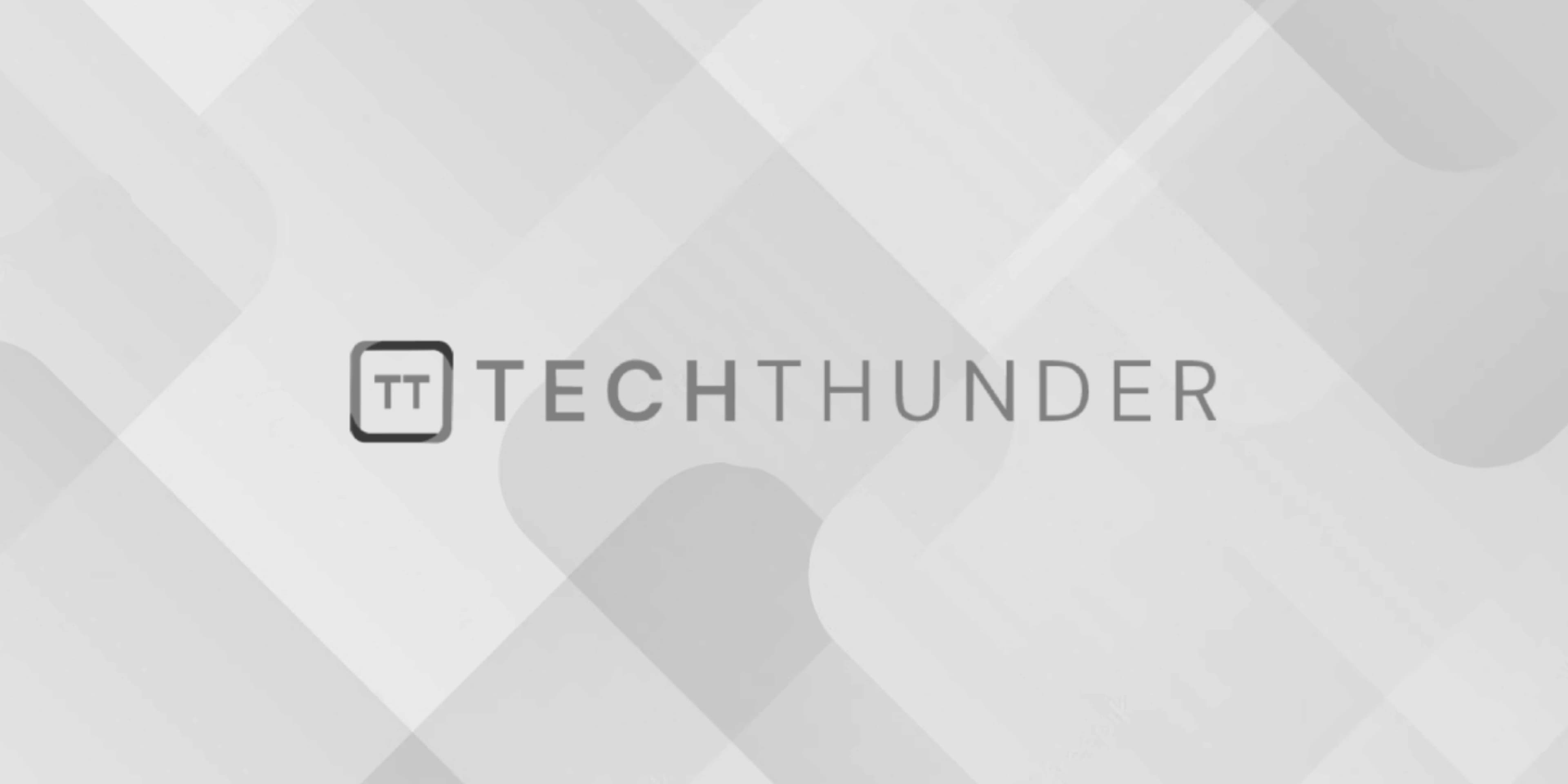
Deadlock in Java
Deadlock is a common issue that can occur in multithreaded Java applications when two or more threads are blocked, each waiting for a resource that another thread holds. As a result, the threads end up in a circular wait, and the program can’t make any progress, leading to a deadlock situation.
Deadlocks can happen due to improper use of synchronization and concurrent access to shared resources. To understand deadlock, let’s consider a classic example using two threads and two resources:
public class DeadlockExample {
private static final Object resource1 = new Object();
private static final Object resource2 = new Object();
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
synchronized (resource1) {
System.out.println("Thread 1: Holding resource 1...");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread 1: Waiting for resource 2...");
synchronized (resource2) {
System.out.println("Thread 1: Acquired resource 2!");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (resource2) {
System.out.println("Thread 2: Holding resource 2...");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread 2: Waiting for resource 1...");
synchronized (resource1) {
System.out.println("Thread 2: Acquired resource 1!");
}
}
});
thread1.start();
thread2.start();
}
}
In this example, we have two threads, thread1
and thread2
, and two resources, resource1
and resource2
. Each thread acquires one resource and then tries to acquire the other. Since both threads wait for the resource the other thread holds, they end up in a deadlock.
When you run this code, it may result in a deadlock, and the program will be stuck with no further progress. Deadlocks are complex issues and can be difficult to debug because they often occur sporadically based on the timing of thread scheduling.
To prevent deadlocks, it’s essential to follow some best practices for concurrent programming:
- Avoid Circular Wait: Ensure that threads acquire shared resources in a fixed and consistent order. This avoids the possibility of circular waits.
- Acquire Locks in a Single Operation: Use a single synchronized block to acquire multiple locks simultaneously, if required, to avoid the possibility of deadlocks.
- Use Timeout Mechanisms: If possible, use timeout mechanisms when acquiring locks to prevent threads from waiting indefinitely and take appropriate actions if the lock cannot be acquired.
- Minimize Lock Contention: Minimize the duration for which a thread holds a lock to reduce the likelihood of contention.
By following these best practices and carefully managing concurrent access to shared resources, you can minimize the risk of deadlocks in your Java multithreaded applications.