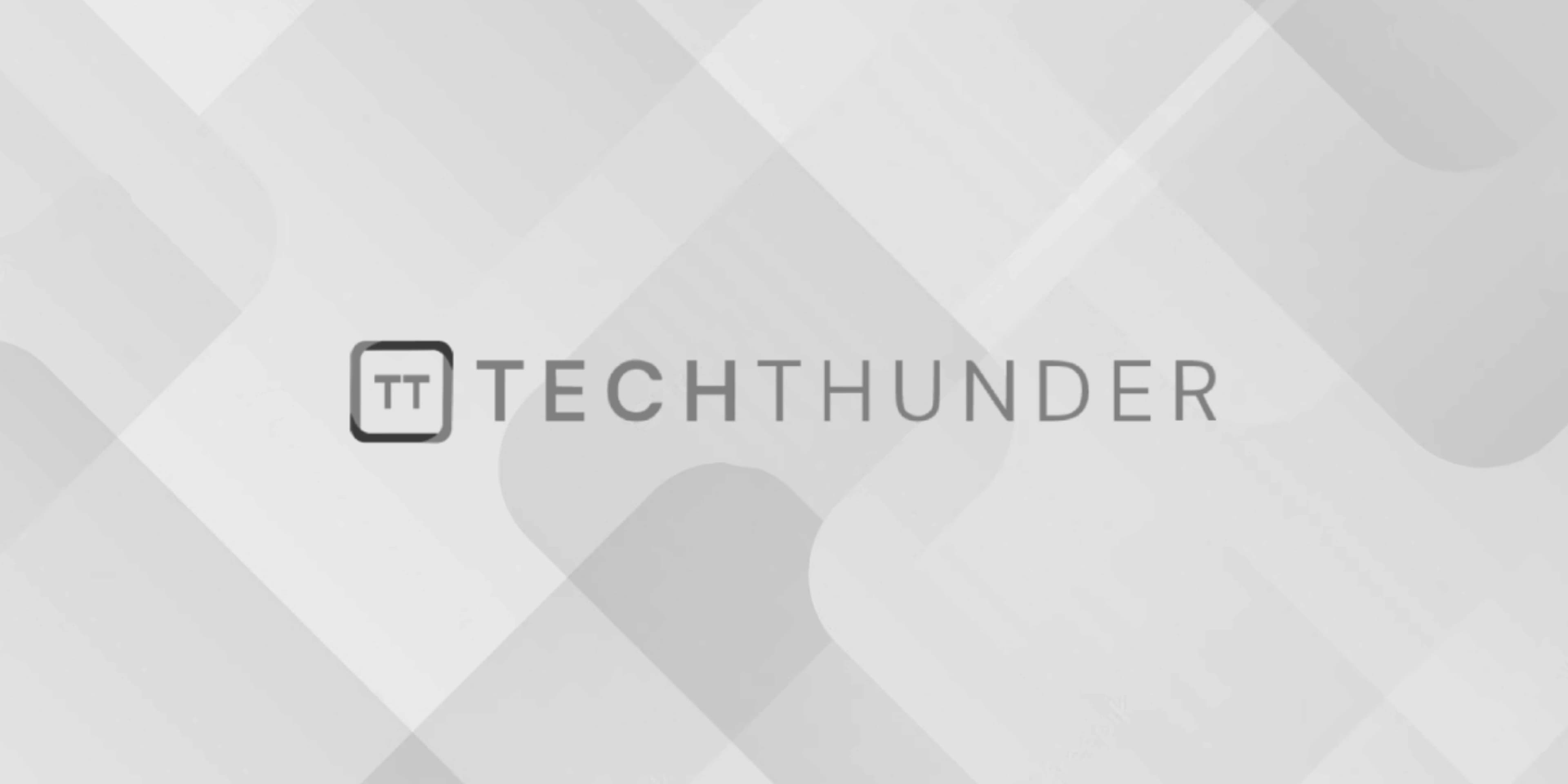
Thread Priority in java
The Java thread priority is a way to indicate the importance or urgency of a thread’s execution. Each thread is assigned a priority value, which is an integer between 1 and 10, with 1 being the lowest priority and 10 being the highest priority. Threads with higher priorities are given preference by the thread scheduler in terms of CPU time. However, the exact behavior of thread priority can vary between different Java Virtual Machine (JVM) implementations and operating systems.
Here are some key points about thread priority in Java:
- Thread Priorities:
- Threads are assigned a priority by using the
setPriority(int priority)
method, wherepriority
is an integer from 1 to 10. - The default priority of a thread is usually inherited from its parent thread. For example, if a thread is created by another thread, it typically inherits the same priority.
- Thread Scheduling:
- The thread scheduler in the JVM uses thread priorities to determine which thread should run when multiple threads are in the runnable state. Higher-priority threads are given preferential treatment by the scheduler, but it’s important to note that thread scheduling is platform-dependent and not guaranteed to be strictly followed.
- Thread Prioritization:
- While thread priorities are a tool for indicating preference, they do not guarantee that a high-priority thread will always execute before a low-priority thread. Other factors, such as the operating system’s thread scheduler and system load, also play a role.
- Use with Caution:
- It’s generally best to use thread priorities sparingly and avoid relying on them for critical synchronization or timing requirements. Instead, consider using higher-level synchronization mechanisms, such as locks and semaphores.
- Thread.MIN_PRIORITY and Thread.MAX_PRIORITY:
- The
Thread
class provides constants for the minimum and maximum priority values:Thread.MIN_PRIORITY
is 1.Thread.MAX_PRIORITY
is 10.
- Thread.getPriority():
- You can use the
getPriority()
method to retrieve the priority of a thread.
Here’s an example of setting and getting thread priorities:
public class ThreadPriorityExample {
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
System.out.println("Thread 1 is running.");
});
Thread thread2 = new Thread(() -> {
System.out.println("Thread 2 is running.");
});
// Set thread priorities
thread1.setPriority(Thread.MIN_PRIORITY); // Priority 1
thread2.setPriority(Thread.MAX_PRIORITY); // Priority 10
// Start the threads
thread1.start();
thread2.start();
// Get thread priorities
int priority1 = thread1.getPriority();
int priority2 = thread2.getPriority();
System.out.println("Thread 1 priority: " + priority1);
System.out.println("Thread 2 priority: " + priority2);
}
}
In this example, we create two threads (thread1
and thread2
) and set their priorities to the minimum and maximum values, respectively. After starting the threads, we retrieve and display their priorities using the getPriority()
method. Keep in mind that thread priority should be used with caution and for specific cases where it is truly needed. Most applications can be developed without relying heavily on thread priorities.