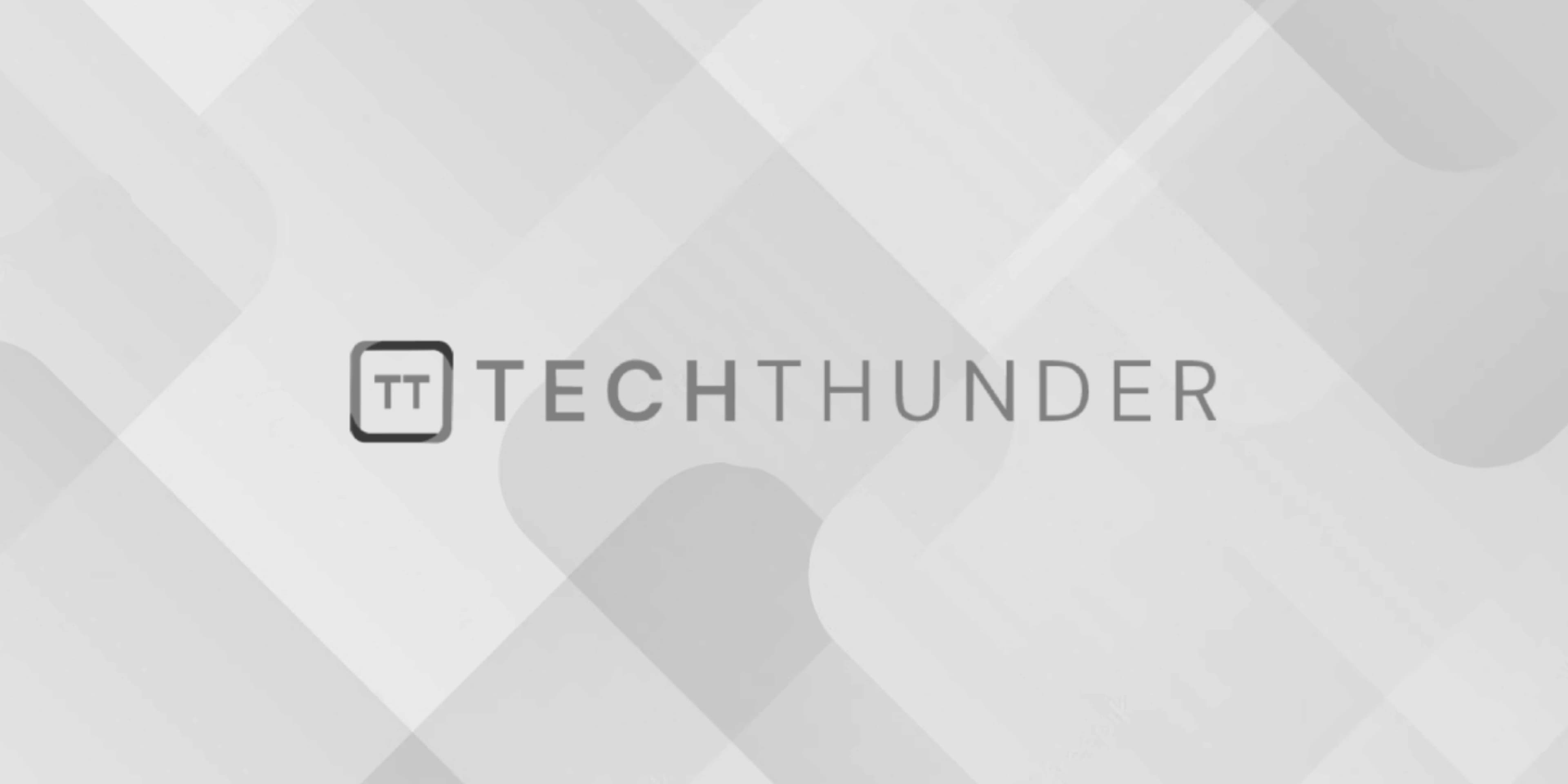
Garbage Collection in java
Garbage collection in Java is an automatic memory management mechanism that automatically reclaims memory from objects that are no longer in use. It is a critical part of the Java Virtual Machine (JVM) that helps prevent memory leaks and ensures efficient memory utilization in Java applications.
In Java, objects are created dynamically using the new
keyword, and the memory for these objects is allocated from the heap memory. The JVM automatically manages the allocation and deallocation of memory for objects. When an object is no longer reachable or referenced by any part of the program, it becomes eligible for garbage collection.
The garbage collection process consists of the following steps:
- Marking: The garbage collector traverses all the objects in the memory and marks those that are reachable from the root objects (e.g., objects referenced directly by the application’s stack and static variables). Any object not marked during this process is considered unreachable and eligible for garbage collection.
- Sweeping: The garbage collector sweeps through the heap memory and reclaims memory occupied by the unreachable objects. It releases the memory back to the heap and marks the space as available for future allocations.
- Compacting: Some garbage collectors perform an additional step of compacting the memory. In this step, the remaining live objects are moved closer together to reduce fragmentation and optimize memory usage.
Java provides different garbage collectors, known as garbage collection algorithms, which are suitable for various types of applications and memory usage patterns. The default garbage collector used by most JVMs is the Garbage-First (G1) garbage collector.
Developers typically don’t need to explicitly trigger garbage collection, as the JVM automatically determines the appropriate time to run the garbage collector based on factors like memory usage, heap size, and application activity. However, you can suggest to the JVM to run garbage collection using the System.gc()
method, but it’s not guaranteed to execute the garbage collector immediately.
Garbage collection offers several benefits:
- Simplified Memory Management: Developers don’t have to manually allocate and deallocate memory for objects, reducing the risk of memory leaks and segmentation faults.
- Improved Memory Utilization: Garbage collection ensures efficient memory usage by automatically reclaiming memory from unused objects.
- Increased Application Performance: Well-tuned garbage collectors help optimize application performance by minimizing the overhead of memory management.
- Reduced Risk of Memory Errors: Garbage collection helps prevent common memory-related bugs, such as accessing deallocated memory (dangling pointers) or memory leaks.
However, garbage collection does come with some overhead. The garbage collection process takes CPU time and can cause brief pauses in the application’s execution, especially in cases of large-scale garbage collection.
In summary, garbage collection is a fundamental feature of Java that enables automatic memory management, making Java applications more robust, secure, and easier to develop. It eliminates the need for manual memory management, which is a common source of bugs and vulnerabilities in other programming languages.