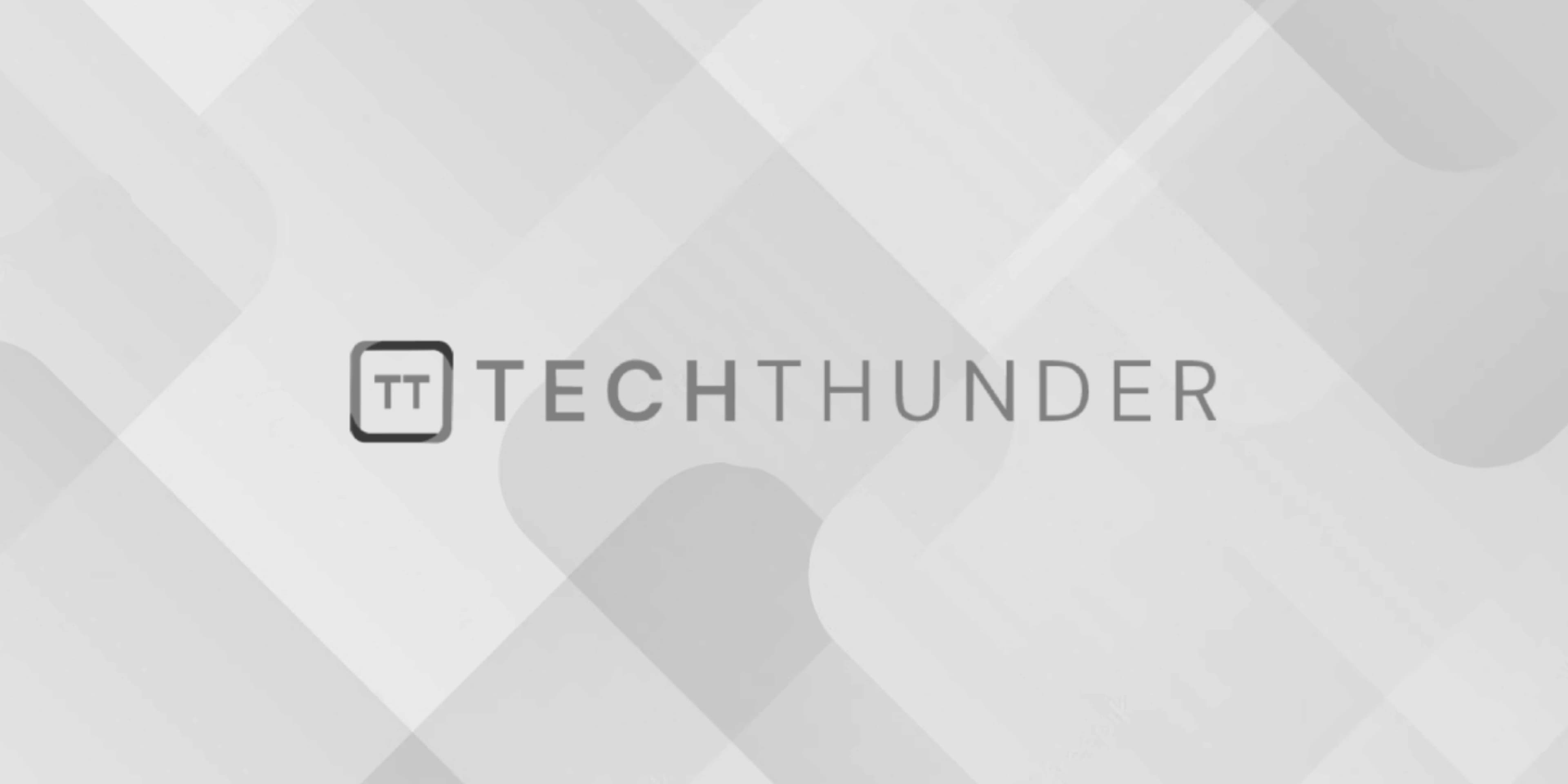
Thread Pool in java
The thread pool in java is a pool of pre-initialized threads that are ready to perform tasks. Thread pools are used to manage and control the number of threads in a concurrent application, which can help improve performance and resource utilization. Creating and destroying threads can be expensive, so thread pools are particularly useful in scenarios where you have many short-lived tasks to perform.
Java provides built-in support for creating thread pools through the java.util.concurrent
package. The most commonly used class for creating thread pools is ExecutorService
. Here’s how you can create and use a thread pool in Java:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Create a thread pool with a fixed number of threads
ExecutorService executorService = Executors.newFixedThreadPool(5);
// Submit tasks to the thread pool
for (int i = 0; i < 10; i++) {
Runnable task = new Task(i);
executorService.submit(task);
}
// Shutdown the thread pool when done
executorService.shutdown();
}
}
class Task implements Runnable {
private int taskId;
public Task(int taskId) {
this.taskId = taskId;
}
@Override
public void run() {
System.out.println("Task " + taskId + " is executing on thread " + Thread.currentThread().getName());
}
}
In the example above:
- We import the necessary classes from the
java.util.concurrent
package. - We create a fixed-size thread pool with five threads using
Executors.newFixedThreadPool(5)
. - We submit ten tasks to the thread pool using the
submit
method. Each task is an instance of theTask
class, which implements theRunnable
interface. - After submitting all tasks, we call
executorService.shutdown()
to shut down the thread pool when all tasks are completed.
Common thread pool configurations in Java include:
newFixedThreadPool(int n)
: Creates a fixed-size thread pool withn
threads.newCachedThreadPool()
: Creates a thread pool that can grow as needed and shrink when idle.newSingleThreadExecutor()
: Creates a thread pool with a single worker thread.newScheduledThreadPool(int corePoolSize)
: Creates a thread pool for scheduling tasks at fixed-rate intervals.
Using thread pools helps manage the overhead of creating and destroying threads, prevents resource exhaustion, and allows you to efficiently utilize available CPU cores in multi-core systems. It’s important to choose the appropriate thread pool configuration based on your application’s requirements and workload.