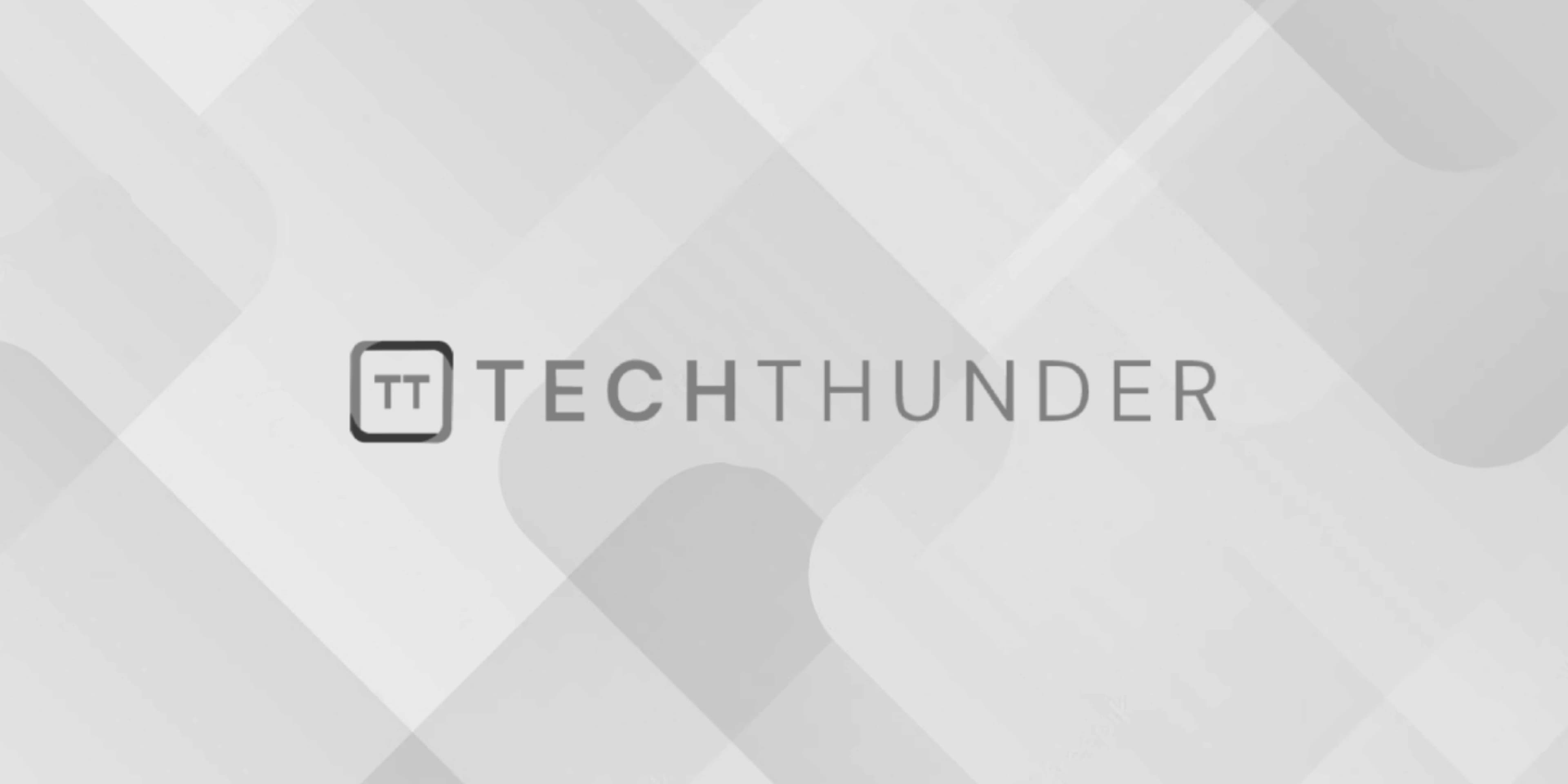
ShutdownHook in java
In Java, a shutdown hook is a special thread that allows developers to perform cleanup actions or tasks just before the Java Virtual Machine (JVM) terminates or shuts down. It provides a way to gracefully shutdown an application and release any resources or perform necessary cleanup operations before the program exits.
To register a shutdown hook, you use the Runtime.addShutdownHook(Thread hook)
method or the java.lang.System
class’ addShutdownHook(Thread hook)
method. These methods allow you to attach a Thread
object as a shutdown hook, and the thread’s run()
method will be executed when the JVM is about to shut down.
Here’s an example of using a shutdown hook:
public class ShutdownHookExample {
public static void main(String[] args) {
// Create and register a shutdown hook
Thread shutdownHook = new Thread(() -> {
System.out.println("Shutdown hook is executing...");
// Perform cleanup tasks here, like closing resources or saving data
System.out.println("Cleanup tasks completed.");
});
Runtime.getRuntime().addShutdownHook(shutdownHook);
// Simulate some application work
for (int i = 1; i <= 5; i++) {
System.out.println("Working... " + i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Unregister the shutdown hook (optional)
// Runtime.getRuntime().removeShutdownHook(shutdownHook);
}
}
In this example, we create a Thread
named shutdownHook
that performs cleanup tasks in its run()
method. We then use Runtime.getRuntime().addShutdownHook(shutdownHook)
to register the shutdown hook. The run()
method will be executed when the JVM is about to shut down.
During the execution of the main thread, we simulate some application work with a loop and a 1-second delay. Once the application completes its work, the JVM will begin its shutdown process, and the registered shutdown hook will be executed.
It’s important to note the following points when using shutdown hooks:
- The order of execution of multiple registered shutdown hooks is not guaranteed. They will run concurrently during the shutdown process.
- Avoid performing tasks in the shutdown hook that might take too long to complete, as it can delay the JVM shutdown process.
- The shutdown hook should not rely on the state of other threads, as other threads may have already terminated or be in the process of shutting down.
- Avoid unconditionally calling
System.exit()
from the shutdown hook, as it can lead to an infinite loop of shutdown hooks.
Shutdown hooks are particularly useful for releasing resources, saving data, or gracefully shutting down components before the application exits. They provide a way to ensure that necessary cleanup tasks are performed, even if the application is terminated unexpectedly.