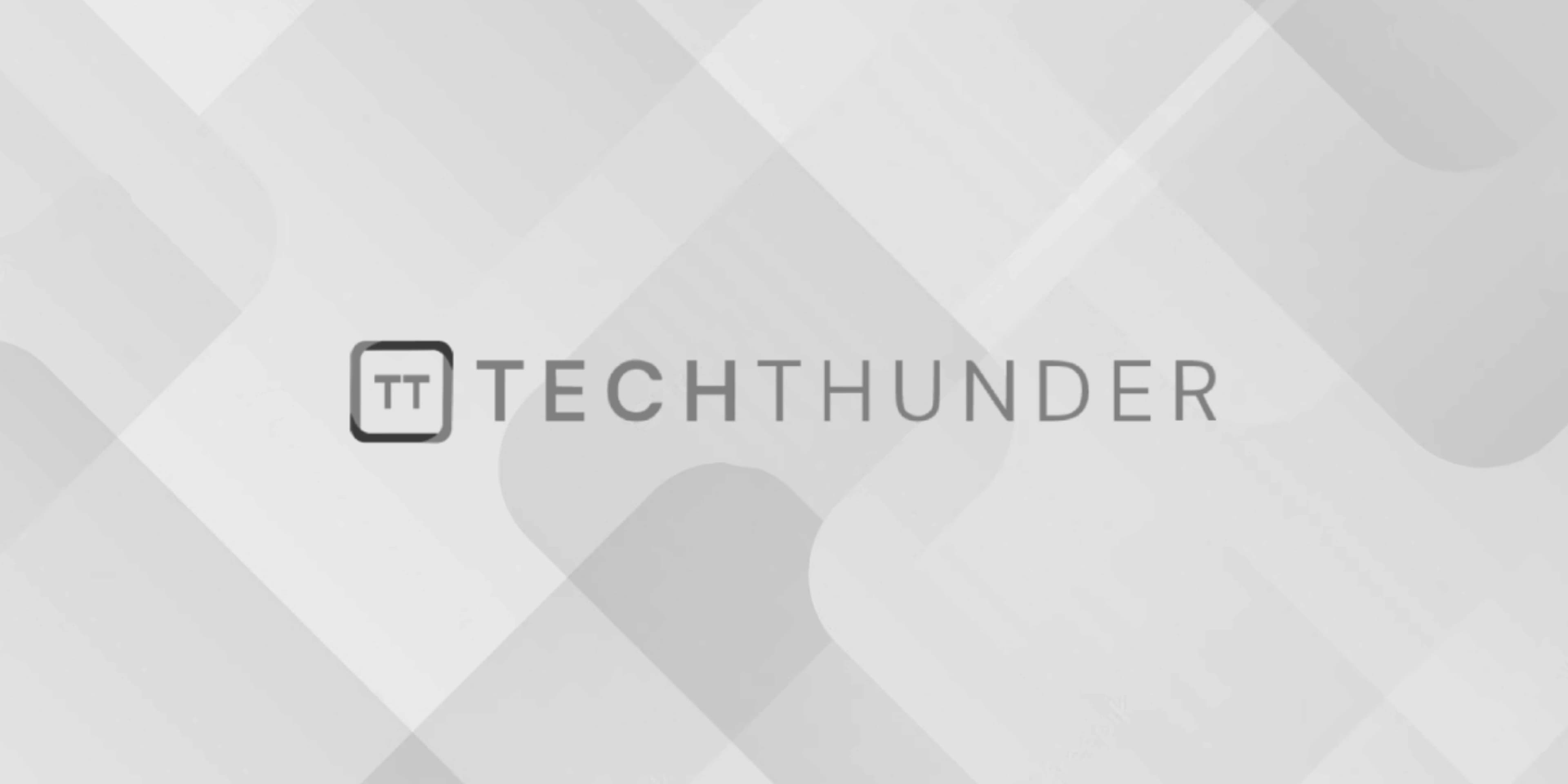
Thread Group in java
The Java thread group is a way to group related threads together for easier management and monitoring. Thread groups provide a hierarchical structure for organizing threads in a Java application. You can perform various operations on a thread group, such as setting thread priorities, interrupting threads, and uncaught exception handling, for all threads in the group. Thread groups can be useful for managing threads with common characteristics or behavior.
Here are the key aspects of thread groups in Java:
- Creating a Thread Group:
- You can create a new thread group by instantiating the
ThreadGroup
class with a name. For example:
ThreadGroup myGroup = new ThreadGroup("MyThreadGroup");
- Adding Threads to a Thread Group:
- When creating threads, you can specify a thread group for the thread in the constructor. Threads created without specifying a group are assigned to the same group as their parent thread.
Thread myThread = new Thread(myGroup, () -> {
// Thread's task
});
- Getting Thread Group Information:
- You can obtain information about a thread group, such as its name, parent group, active count, and maximum priority, using various methods provided by the
ThreadGroup
class.
- Enumerating Threads:
- You can enumerate all the threads in a thread group using the
enumerate(Thread[] tarray)
method. This allows you to inspect the threads within the group.
- Enumerating Subgroups:
- Thread groups can have subgroups, forming a hierarchical structure. You can enumerate the subgroups within a thread group using the
enumerate(ThreadGroup[] garray)
method.
- Setting Thread Priorities:
- You can set the priorities of all threads within a thread group using the
setMaxPriority(int priority)
method of theThreadGroup
class. This can be used to enforce a maximum priority for all threads in the group.
- Uncaught Exception Handling:
- You can set an uncaught exception handler for a thread group using the
setUncaughtExceptionHandler(Thread.UncaughtExceptionHandler eh)
method. This allows you to specify a handler for uncaught exceptions in threads within the group.
- Thread Group Destruction:
- Thread groups can be destroyed using the
destroy()
method. When a group is destroyed, all threads and subgroups within it are also destroyed.
Thread groups can be helpful for organizing and managing threads in a structured manner, especially in situations where you need to handle a set of threads with a shared purpose, such as in thread pooling or task management. However, keep in mind that thread groups have been somewhat deprecated and are less commonly used in modern Java applications. Higher-level concurrency constructs and libraries are often preferred for managing threads and tasks in a more controlled and scalable way.