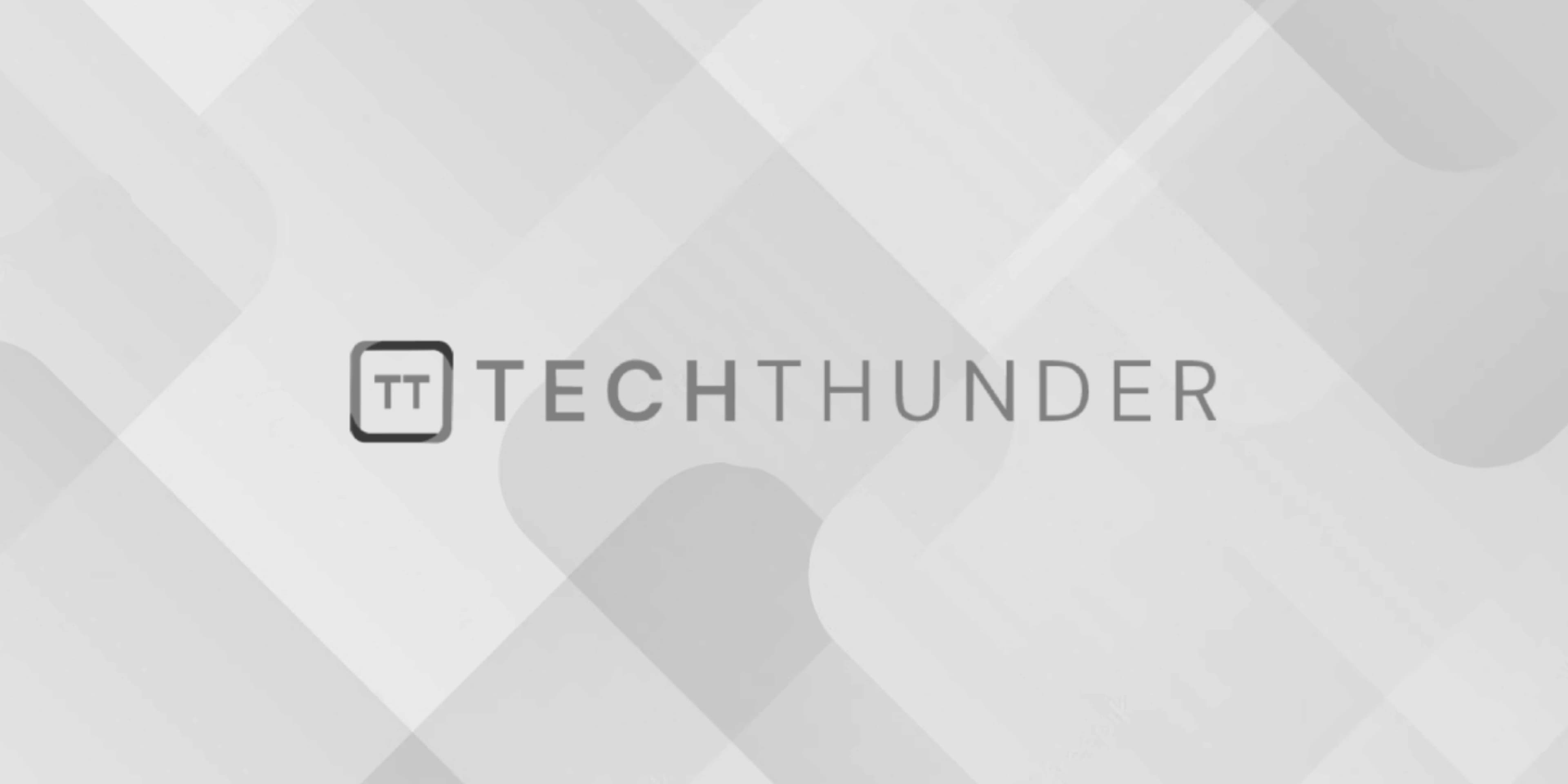
Java Reentrant Monitor
In Java, the concept of a “monitor” refers to a synchronization mechanism used to control access to shared resources in a multithreaded environment. It allows multiple threads to coordinate and cooperate with each other while accessing critical sections of code, ensuring that only one thread can execute the synchronized section at a time.
Java provides built-in support for monitors through the synchronized
keyword and the wait()
, notify()
, and notifyAll()
methods. These mechanisms are essential for creating thread-safe applications and preventing data races and other concurrency-related issues.
Here’s an overview of the key components of the Java Reentrant Monitor:
- synchronized Keyword: In Java, the
synchronized
keyword is used to mark a method or a code block as a critical section. When a thread encounters asynchronized
block, it must acquire the monitor lock associated with the object before entering the block. Only one thread can hold the monitor lock at a time, ensuring exclusive access to the critical section. - Monitor Lock: The monitor lock is associated with an object. When a thread enters a synchronized block, it acquires the monitor lock for the object on which the block is synchronized. If another thread tries to enter the same synchronized block, it will be blocked until the first thread releases the monitor lock by exiting the block.
- Reentrant Behavior: Java monitors are reentrant, which means that a thread can acquire the same monitor lock multiple times without getting blocked. Reentrant behavior allows a thread that already holds the monitor lock to enter the synchronized block without any issues. This feature enables thread-safe recursion within synchronized methods.
- wait() and notify()/notifyAll(): The
wait()
,notify()
, andnotifyAll()
methods are used for inter-thread communication within a monitor. Threads can callwait()
to release the monitor lock and enter a waiting state until another thread callsnotify()
ornotifyAll()
to wake them up.notify()
wakes up a single waiting thread, whilenotifyAll()
wakes up all waiting threads.
Here’s a simple example of using the Java Reentrant Monitor:
public class ReentrantMonitorExample {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In this example, we have a class ReentrantMonitorExample
with two synchronized methods: increment()
and getCount()
. Both methods are synchronized on the instance of the ReentrantMonitorExample
object. This ensures that only one thread can access either method at a time, preventing concurrent modification of the count
variable.
Remember that the synchronized keyword applies only to the methods and code blocks in the same instance of the object. For different instances of the object, the synchronized blocks work independently.
Using Java’s Reentrant Monitor effectively helps manage shared resources and ensures thread safety in multithreaded applications. It’s essential to use synchronized blocks judiciously to avoid potential performance bottlenecks and deadlocks in the application.