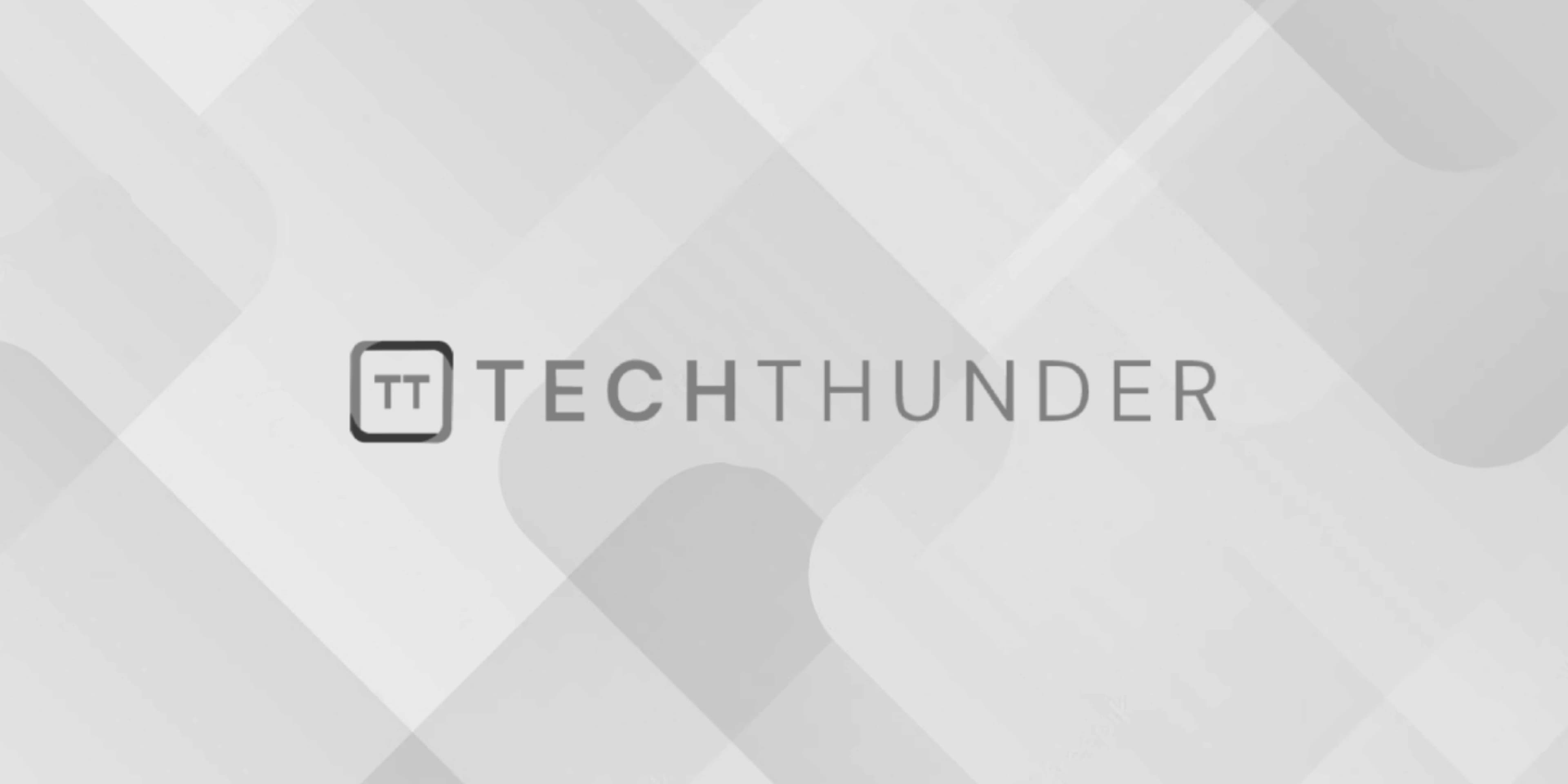
Convert the Column Type from String to Datetime Format in Pandas DataFrame in Python
To convert a column type from a string to a datetime format in a Pandas DataFrame, you can use the pd.to_datetime()
function. This function allows you to convert string representations of dates and times into actual datetime objects in Pandas. Here’s how to do it:
Assuming you have a DataFrame named df
with a column named 'date_column'
containing date values as strings:
import pandas as pd
# Sample DataFrame
data = {'date_column': ['2023-10-05', '2023-10-06', '2023-10-07']}
df = pd.DataFrame(data)
# Convert the 'date_column' to datetime format
df['date_column'] = pd.to_datetime(df['date_column'])
# Check the updated DataFrame
print(df)
In this example, we:
- Import the Pandas library.
- Create a sample DataFrame (
df
) with a column named'date_column'
containing date values as strings. - Use the
pd.to_datetime()
function to convert the'date_column'
to datetime format and assign the result back to the same column in the DataFrame.
After running this code, the 'date_column'
will be converted to a datetime format, and you can perform various datetime operations on it. If the date strings have a time component, the resulting datetime objects will include both date and time information.
Ensure that the date strings are in a format that Pandas can interpret correctly. If the format is different from the standard ‘YYYY-MM-DD’, you can specify the format using the format
parameter of pd.to_datetime()
. For example:
# Convert date strings in a custom format to datetime
df['date_column'] = pd.to_datetime(df['date_column'], format='%d/%m/%Y')
In this case, the format
parameter specifies the custom date format used in the date strings. Adjust it according to your data’s format.