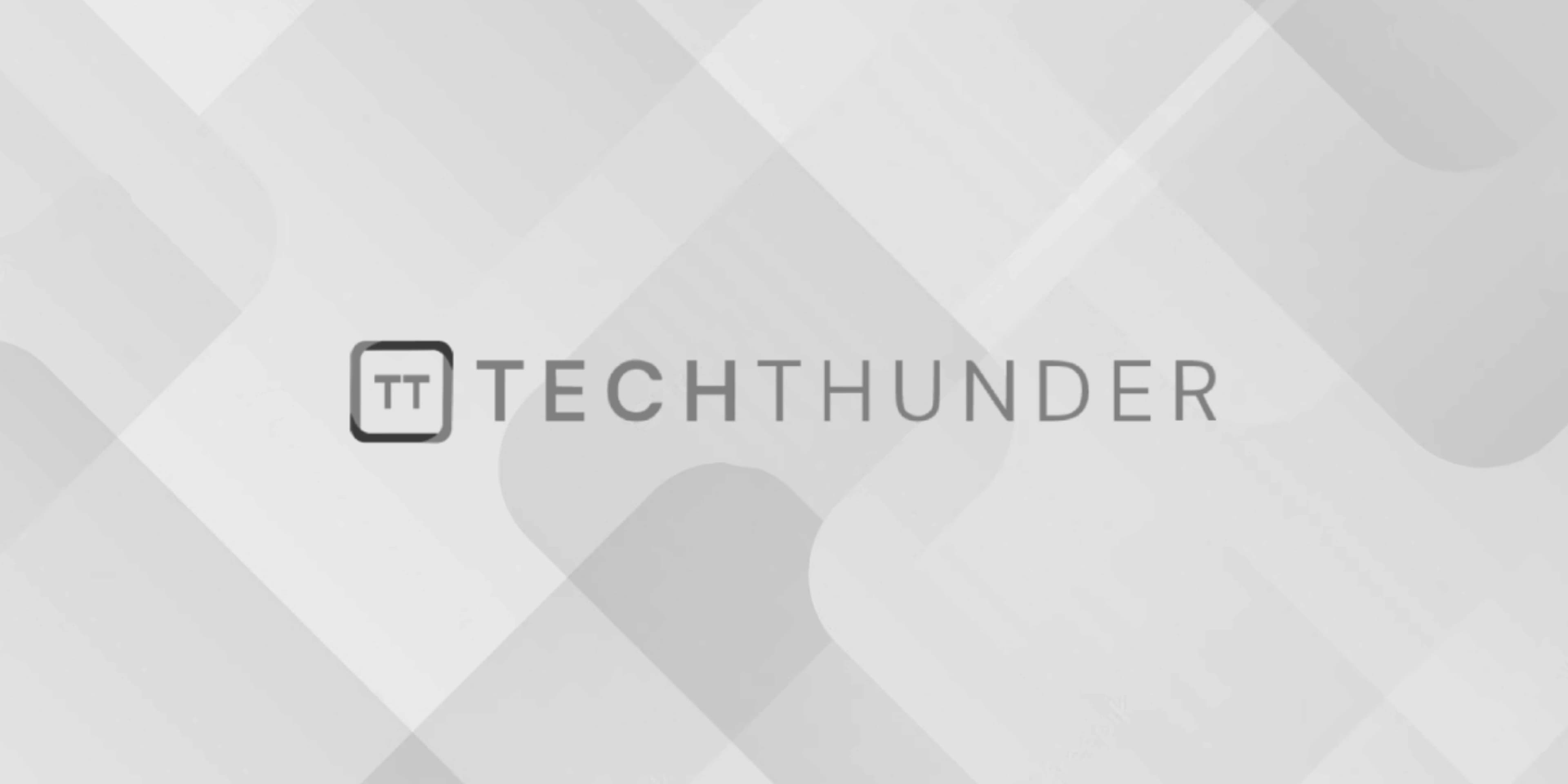
Python Arrays
The Python, arrays are not a built-in data type like lists, tuples, or dictionaries. However, you can work with arrays using the array
module or, more commonly, using the more powerful and flexible numpy
library.
- Using the
array
Module: Thearray
module provides a basic array data structure that is more memory-efficient than Python lists for certain types of operations. To work with arrays using this module, you need to import it:
import array
Here’s an example of creating an array and performing some basic operations:
import array
# Create an array of integers
arr = array.array('i', [1, 2, 3, 4, 5])
# Access elements
print(arr[0]) # Prints the first element (1)
# Append an element
arr.append(6)
# Remove an element
arr.remove(3)
# Iterate through elements
for element in arr:
print(element)
The 'i'
argument to array.array()
specifies that we are creating an array of integers.
- Using the
numpy
Library:numpy
(Numerical Python) is a widely used library for working with arrays, matrices, and numerical computations. It provides a powerfulndarray
(n-dimensional array) data structure and a wide range of mathematical operations for array manipulation. To work withnumpy
, you need to install it first:
pip install numpy
Here’s an example of creating and working with numpy
arrays:
import numpy as np
# Create a 1D array
arr = np.array([1, 2, 3, 4, 5])
# Access elements
print(arr[0]) # Prints the first element (1)
# Perform array operations
arr_squared = arr ** 2
# Slicing
sub_arr = arr[1:4] # Get elements at index 1, 2, and 3
# Array functions
mean = np.mean(arr)
std_dev = np.std(arr)
numpy
provides a rich set of functions for performing mathematical and statistical operations on arrays. It is widely used in scientific and data analysis applications.
While Python lists are more flexible and commonly used for general-purpose programming, arrays (from the array
module or numpy
arrays) are preferred when you need to work with large datasets, perform numerical computations, and take advantage of efficient array operations.