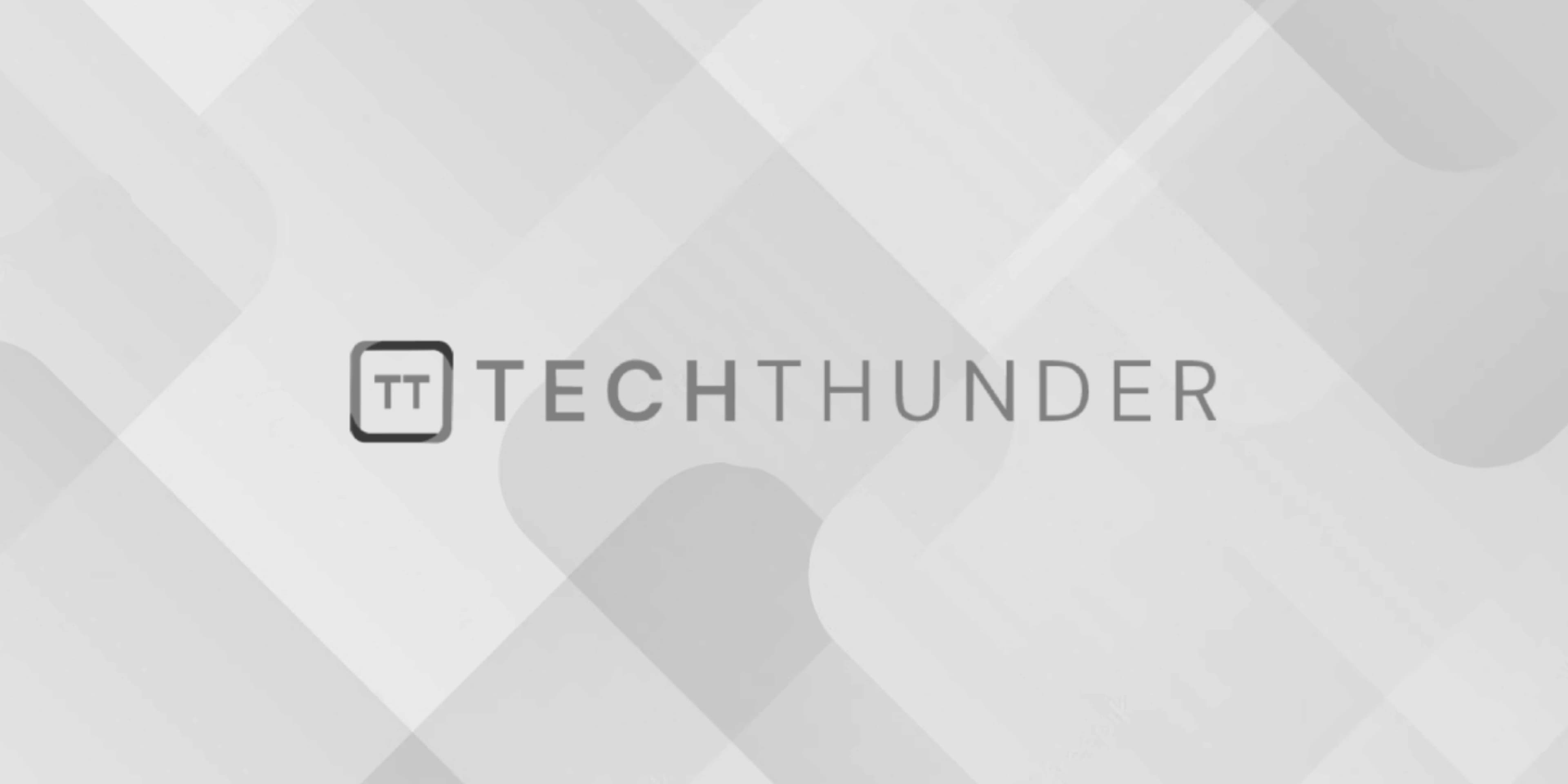
145 views
NumPy Functions in Python
NumPy is a powerful library for numerical and scientific computing in Python. It provides a wide range of functions and features for working with arrays, matrices, and numerical data. Here are some commonly used NumPy functions and features:
1. Creating Arrays:
numpy.array()
: Create a NumPy array from a list or other iterable.numpy.arange()
: Create an array with evenly spaced values within a specified range.numpy.linspace()
: Create an array with evenly spaced values over a specified interval.numpy.zeros()
: Create an array filled with zeros.numpy.ones()
: Create an array filled with ones.numpy.eye()
: Create a 2D identity matrix.numpy.random.rand()
: Create an array with random values from a uniform distribution.numpy.random.randn()
: Create an array with random values from a standard normal distribution.numpy.random.randint()
: Create an array with random integers within a specified range.
2. Array Operations:
- Arithmetic operations: NumPy arrays support element-wise arithmetic operations (+, -, *, /, **).
numpy.dot()
: Compute the dot product of two arrays (matrix multiplication).numpy.transpose()
: Transpose an array or matrix.numpy.sum()
,numpy.mean()
,numpy.median()
,numpy.min()
,numpy.max()
: Compute statistics on arrays.numpy.sort()
: Sort an array.numpy.argmax()
,numpy.argmin()
: Find the indices of the maximum and minimum values.
3. Indexing and Slicing:
- NumPy arrays support indexing and slicing similar to Python lists.
- You can use integer indexing, slicing with colon notation, and boolean indexing to access and manipulate elements.
4. Reshaping and Manipulating Arrays:
numpy.reshape()
: Change the shape of an array.numpy.concatenate()
: Join arrays along an existing axis.numpy.split()
: Split an array into multiple sub-arrays.numpy.append()
,numpy.insert()
,numpy.delete()
: Add, insert, or remove elements from an array.numpy.unique()
: Find the unique elements of an array.
5. Mathematical and Statistical Functions:
numpy.sin()
,numpy.cos()
,numpy.exp()
,numpy.log()
,numpy.sqrt()
: Perform various mathematical operations on arrays element-wise.numpy.sum()
,numpy.mean()
,numpy.median()
,numpy.var()
,numpy.std()
: Compute statistics on arrays.numpy.correlate()
,numpy.cov()
: Calculate the correlation or covariance between two arrays.
6. Broadcasting:
- NumPy allows operations between arrays of different shapes through broadcasting.
- Broadcasting automatically expands smaller arrays to match the shape of larger arrays for element-wise operations.
7. File I/O:
numpy.save()
: Save an array to a binary file.numpy.load()
: Load an array from a binary file.numpy.savetxt()
: Save an array to a text file.numpy.loadtxt()
: Load an array from a text file.
8. Linear Algebra:
numpy.linalg.inv()
: Compute the inverse of a matrix.numpy.linalg.det()
: Calculate the determinant of a matrix.numpy.linalg.eig()
: Compute eigenvalues and eigenvectors of a matrix.numpy.linalg.solve()
: Solve a linear system of equations.
These are just a few examples of the many functions and capabilities that NumPy provides. NumPy is a fundamental library for data manipulation and scientific computing in Python, and it forms the foundation for many other data science libraries and tools.