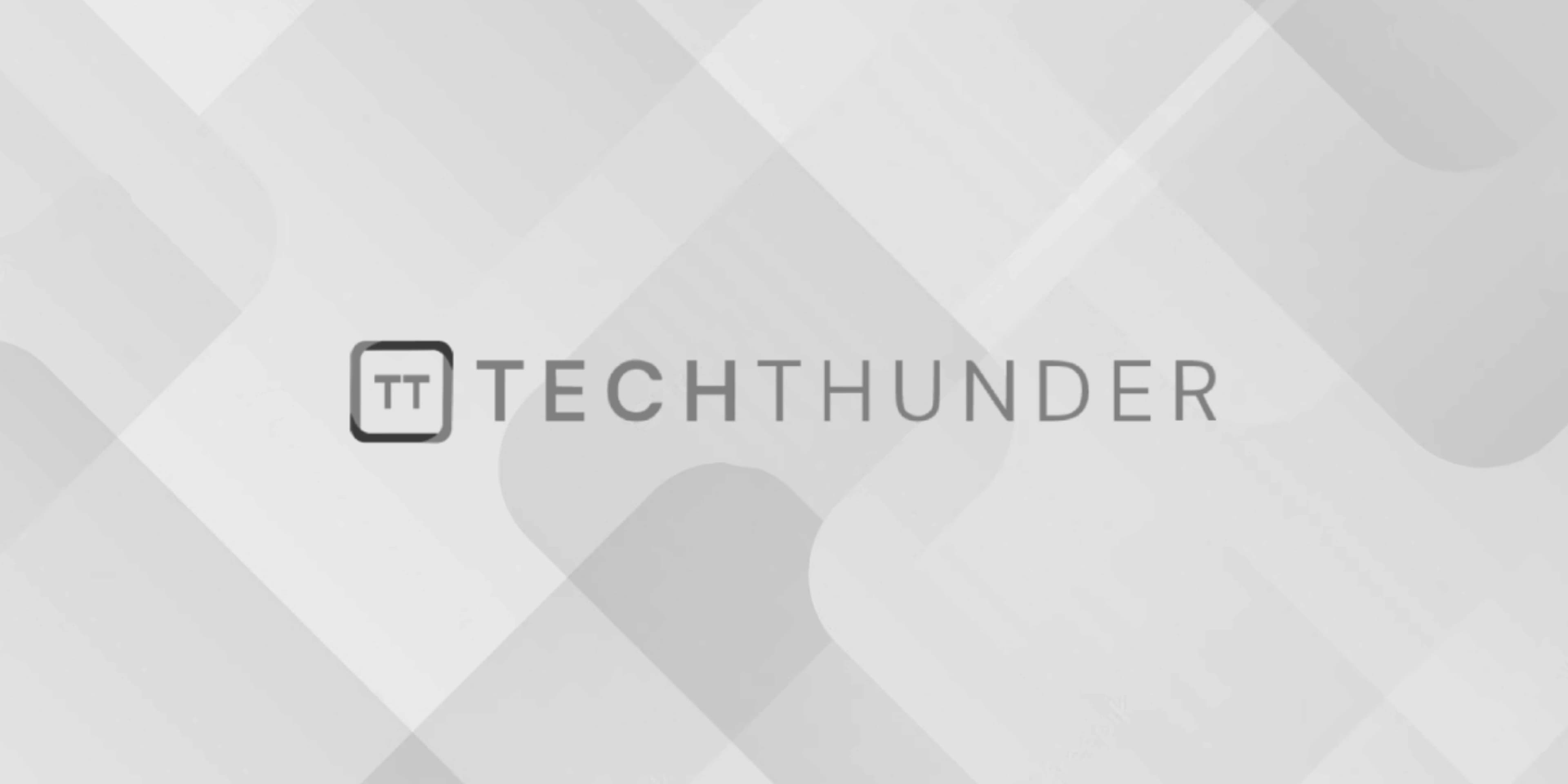
Performing Transactions in Python
The Python, you can perform transactions on a database using database libraries that correspond to your chosen database management system (DBMS), such as SQLite or PostgreSQL. Transactions are used to group a series of SQL statements into a single unit of work that is either completed successfully or not at all. This helps maintain the integrity of the database. I’ll provide examples for performing transactions in both SQLite and PostgreSQL.
Performing Transactions in SQLite:
SQLite is a lightweight and built-in database system in Python. You can perform transactions in SQLite as follows:
- Import the SQLite Module:
import sqlite3
- Connect to the SQLite Database:
conn = sqlite3.connect('mydatabase.db')
Replace 'mydatabase.db'
with the name of your SQLite database file.
- Create a Cursor Object:
cursor = conn.cursor()
- Start a Transaction: To start a transaction, use the
begin()
method on the connection object:
conn.begin()
Alternatively, you can use the begin()
method as a context manager to ensure that the transaction is committed or rolled back automatically:
with conn:
# SQL statements within the transaction
cursor.execute("INSERT INTO users (username, email) VALUES (?, ?)", ("Alice", "alice@example.com"))
cursor.execute("UPDATE users SET email = ? WHERE username = ?", ("new_email@example.com", "Alice"))
The above code will automatically commit the changes if the code block completes successfully or roll back the changes if an exception occurs.
- Commit or Rollback the Transaction: To commit the changes made during the transaction, use the
commit()
method:
conn.commit()
To roll back the transaction and discard any changes, use the rollback()
method:
conn.rollback()
- Close the Connection:
conn.close()
Performing Transactions in PostgreSQL:
PostgreSQL is a popular open-source relational database management system. To perform transactions in PostgreSQL, you’ll need to use the psycopg2
library, which is a PostgreSQL adapter for Python. You can install it using pip
:
pip install psycopg2
Here’s how you can perform transactions in PostgreSQL:
- Import the
psycopg2
Module:
import psycopg2
- Connect to the PostgreSQL Database:
conn = psycopg2.connect(
host="your_host",
database="your_database",
user="your_user",
password="your_password"
)
Replace "your_host"
, "your_database"
, "your_user"
, and "your_password"
with your PostgreSQL server details.
- Create a Cursor Object:
cursor = conn.cursor()
- Start a Transaction: To start a transaction, use the
begin()
method on the connection object:
conn.begin()
Alternatively, you can use the begin()
method as a context manager to ensure that the transaction is committed or rolled back automatically:
with conn:
# SQL statements within the transaction
cursor.execute("INSERT INTO users (username, email) VALUES (%s, %s)", ("Alice", "alice@example.com"))
cursor.execute("UPDATE users SET email = %s WHERE username = %s", ("new_email@example.com", "Alice"))
The above code will automatically commit the changes if the code block completes successfully or roll back the changes if an exception occurs.
- Commit or Rollback the Transaction: To commit the changes made during the transaction, use the
commit()
method:
conn.commit()
To roll back the transaction and discard any changes, use the rollback()
method:
conn.rollback()
- Close the Connection:
conn.close()
Remember to replace the placeholders with your actual database connection details when using PostgreSQL. Using transactions is essential for maintaining data consistency and integrity in a database, especially when multiple SQL statements need to be executed as a single unit of work.