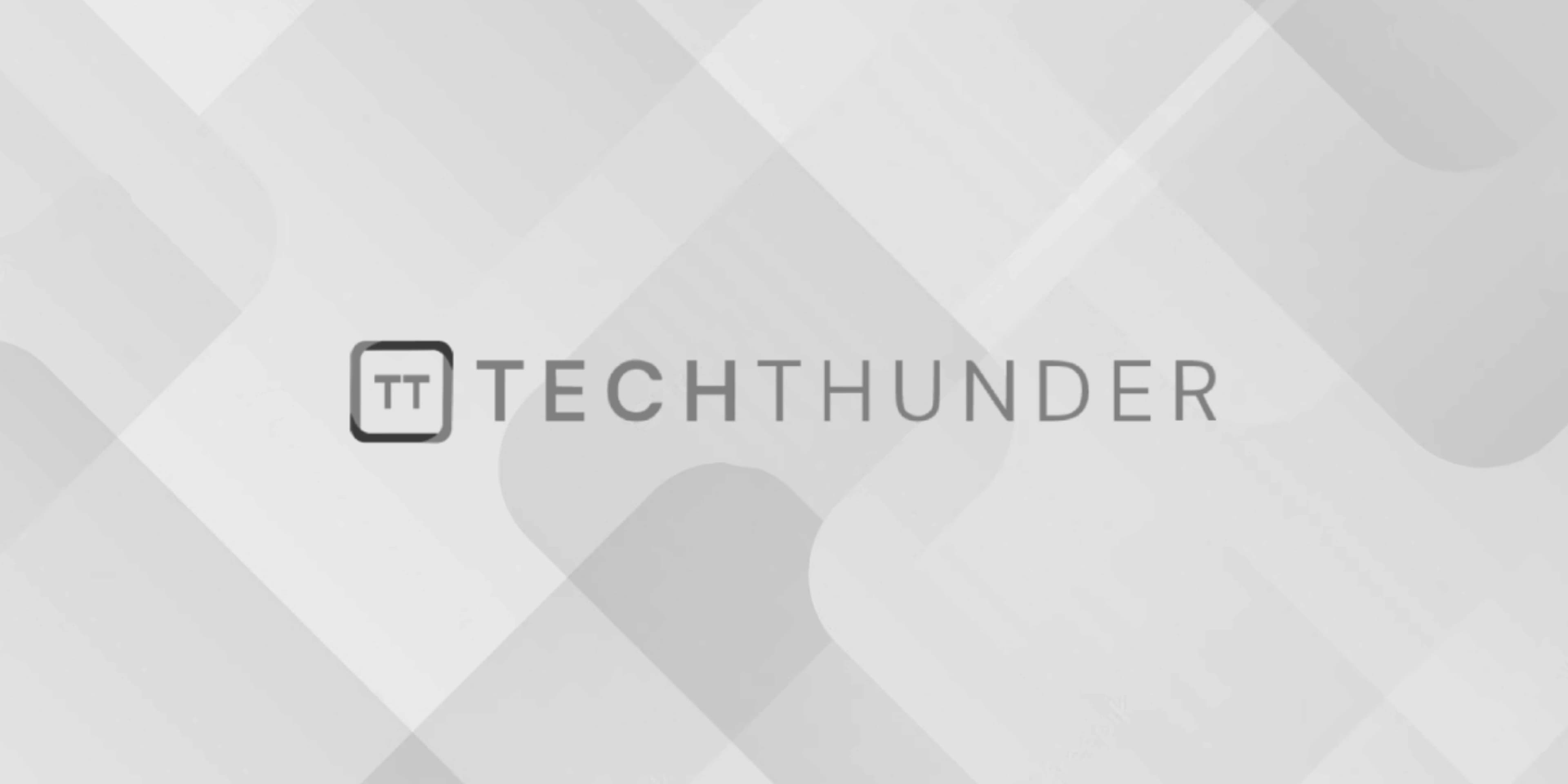
112 views
Return Two Values from a Function Python
The Python can return multiple values from a function by using one of the following methods:
- Using a Tuple:
- You can return multiple values as a tuple, which is a collection of ordered, immutable elements enclosed in parentheses.
Python
def return_multiple_values():
value1 = 42
value2 = "Hello, World!"
return value1, value2
result = return_multiple_values()
print(result) # Output: (42, 'Hello, World!')
You can unpack the returned tuple into separate variables, like this:
Python
value1, value2 = return_multiple_values()
print(value1) # Output: 42
print(value2) # Output: 'Hello, World!'
- Using a List:
- You can also return multiple values as a list, which is a collection of ordered, mutable elements enclosed in square brackets.
Python
def return_multiple_values():
value1 = 42
value2 = "Hello, World!"
return [value1, value2]
result = return_multiple_values()
print(result) # Output: [42, 'Hello, World!']
You can access the elements of the returned list by indexing:
Python
value1 = result[0] # 42
value2 = result[1] # 'Hello, World!'
- Using Named Tuples:
- Named tuples are similar to tuples but allow you to give names to elements, making it more readable and self-documenting.
Python
from collections import namedtuple
def return_multiple_values():
MyTuple = namedtuple("MyTuple", ["value1", "value2"])
return MyTuple(value1=42, value2="Hello, World!")
result = return_multiple_values()
print(result.value1) # Output: 42
print(result.value2) # Output: 'Hello, World!'
- Using a Dictionary:
- You can return multiple values as a dictionary, where each value is associated with a specific key.
Python
def return_multiple_values():
return {"value1": 42, "value2": "Hello, World!"}
result = return_multiple_values()
print(result["value1"]) # Output: 42
print(result["value2"]) # Output: 'Hello, World!'
Each of these methods allows you to return multiple values from a function, and you can choose the one that best fits your use case and coding style.