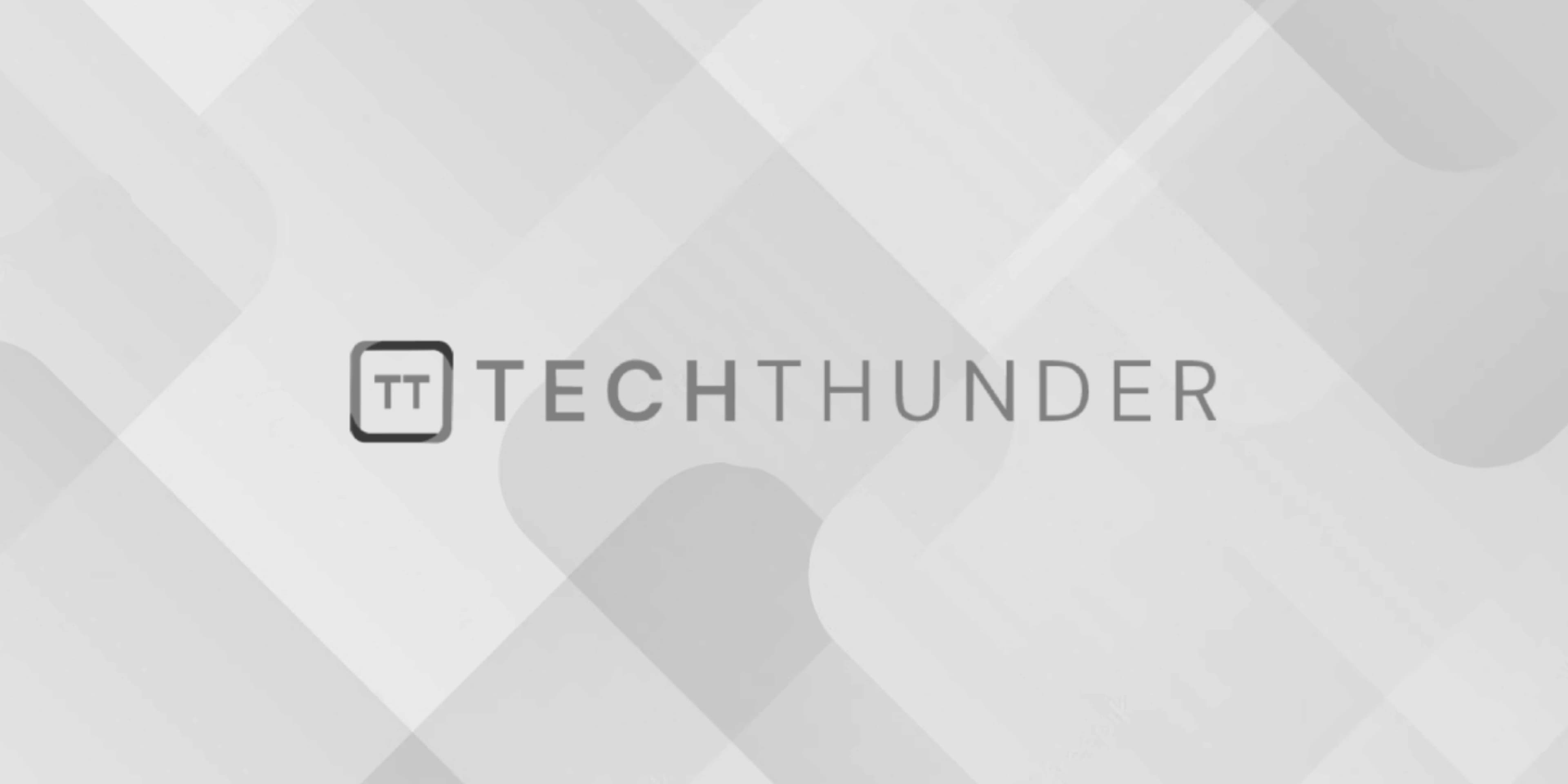
Implementing Artificial Neural Network Training Process in Python
Training an Artificial Neural Network (ANN) in Python involves several steps, including data preparation, model design, training, evaluation, and fine-tuning. Below is a basic example of how to implement the training process of an ANN using the popular deep learning library, TensorFlow, and its high-level API, Keras. This example demonstrates a simple feedforward neural network for a classification task:
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
# 1. Data Preparation
# Load your dataset, split it into training and testing sets, and preprocess the data.
# Example dataset
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train, x_test = x_train / 255.0, x_test / 255.0 # Normalize pixel values
# 2. Model Design
# Define the architecture of your neural network.
model = keras.Sequential([
layers.Flatten(input_shape=(28, 28)), # Input layer (Flatten 28x28 images)
layers.Dense(128, activation='relu'), # Hidden layer with ReLU activation
layers.Dropout(0.2), # Dropout layer to reduce overfitting
layers.Dense(10, activation='softmax') # Output layer with softmax activation (10 classes)
])
# 3. Compile the Model
# Specify the loss function, optimizer, and evaluation metrics.
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 4. Model Training
# Train the model on the training data.
model.fit(x_train, y_train, epochs=5) # Adjust the number of epochs as needed
# 5. Model Evaluation
# Evaluate the model's performance on the test data.
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f"Test accuracy: {test_acc}")
# 6. Model Prediction
# Use the trained model to make predictions.
predictions = model.predict(x_test)
# 7. Fine-Tuning (Optional)
# You can fine-tune hyperparameters, model architecture, and other aspects to improve performance.
# Example: Adjust the learning rate of the optimizer
custom_optimizer = keras.optimizers.Adam(learning_rate=0.001)
model.compile(optimizer=custom_optimizer, loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Continue training if needed
model.fit(x_train, y_train, epochs=5)
# Save the trained model if desired
model.save("my_model.h5")
This example demonstrates a simple feedforward neural network for classifying handwritten digits from the MNIST dataset. You can replace this dataset with your own data and customize the architecture and hyperparameters to fit your specific task.
The key steps in training an ANN are data preparation, model design, compilation, training, evaluation, and fine-tuning. Depending on your specific problem, you may need to adjust the architecture, loss function, optimizer, and other parameters to achieve the best performance.