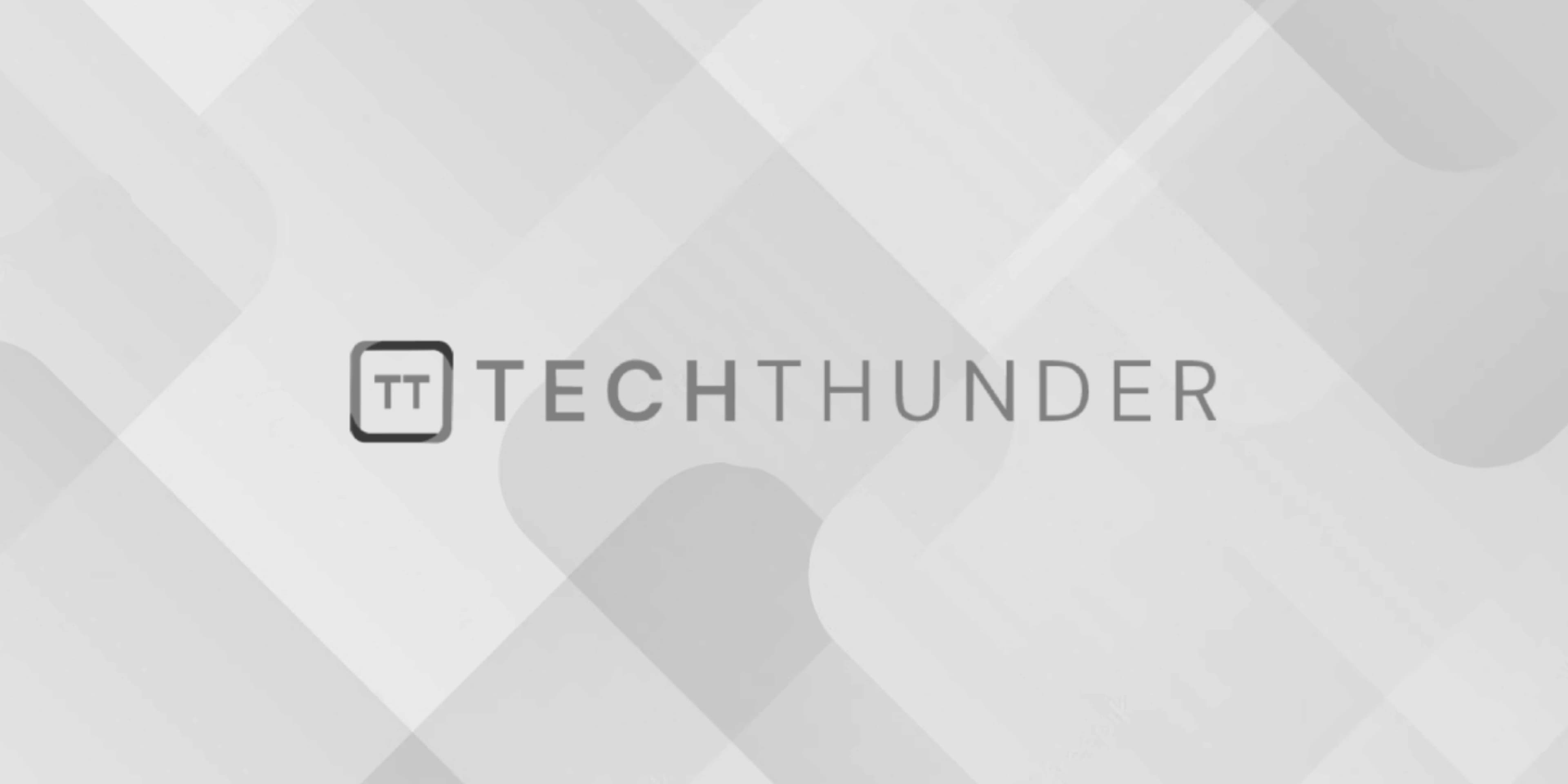
Spinner Widget in the kivy Library of Python
The Kivy library for Python, a Spinner widget is used to provide a dropdown menu or selection list of items. It allows users to choose one item from a list of options by clicking on the dropdown arrow and selecting from the available choices. The selected item is then displayed in the Spinner’s main area.
Here’s how you can create and use a Spinner widget in a Kivy application:
- Import Kivy Libraries: Make sure you import the necessary modules from the Kivy library:
from kivy.app import App
from kivy.uix.spinner import Spinner
from kivy.uix.boxlayout import BoxLayout
- Create a Kivy App Class: Define a Kivy App class that will contain the user interface components.
class SpinnerExample(App):
def build(self):
# Create the UI layout
layout = BoxLayout(orientation='vertical', padding=10, spacing=10)
# Create a Spinner widget
spinner = Spinner(
text='Select an item', # Default text displayed
values=('Option 1', 'Option 2', 'Option 3'), # List of options
size_hint=(None, None),
size=(200, 44)
)
# Bind a function to be called when an option is selected
spinner.bind(on_text=self.on_spinner_select)
# Add the Spinner to the layout
layout.add_widget(spinner)
return layout
- Define a Function for Handling Selection: Define a function that will be called when an item is selected from the Spinner. You can perform actions based on the selected item inside this function.
def on_spinner_select(self, instance, text):
# Print the selected item to the console
print(f"Selected: {text}")
- Run the Kivy App: Create an instance of your Kivy App class and run it.
if __name__ == '__main__':
SpinnerExample().run()
When you run the Kivy app, it will display a Spinner with the default text “Select an item.” Clicking on the Spinner will reveal the available options, and when you select an option, the on_spinner_select
function will be called, and the selected item will be printed to the console.
You can customize the appearance and behavior of the Spinner by modifying its properties and handling other events as needed. The Spinner widget is a versatile component for creating dropdown menus and selection lists in Kivy applications.