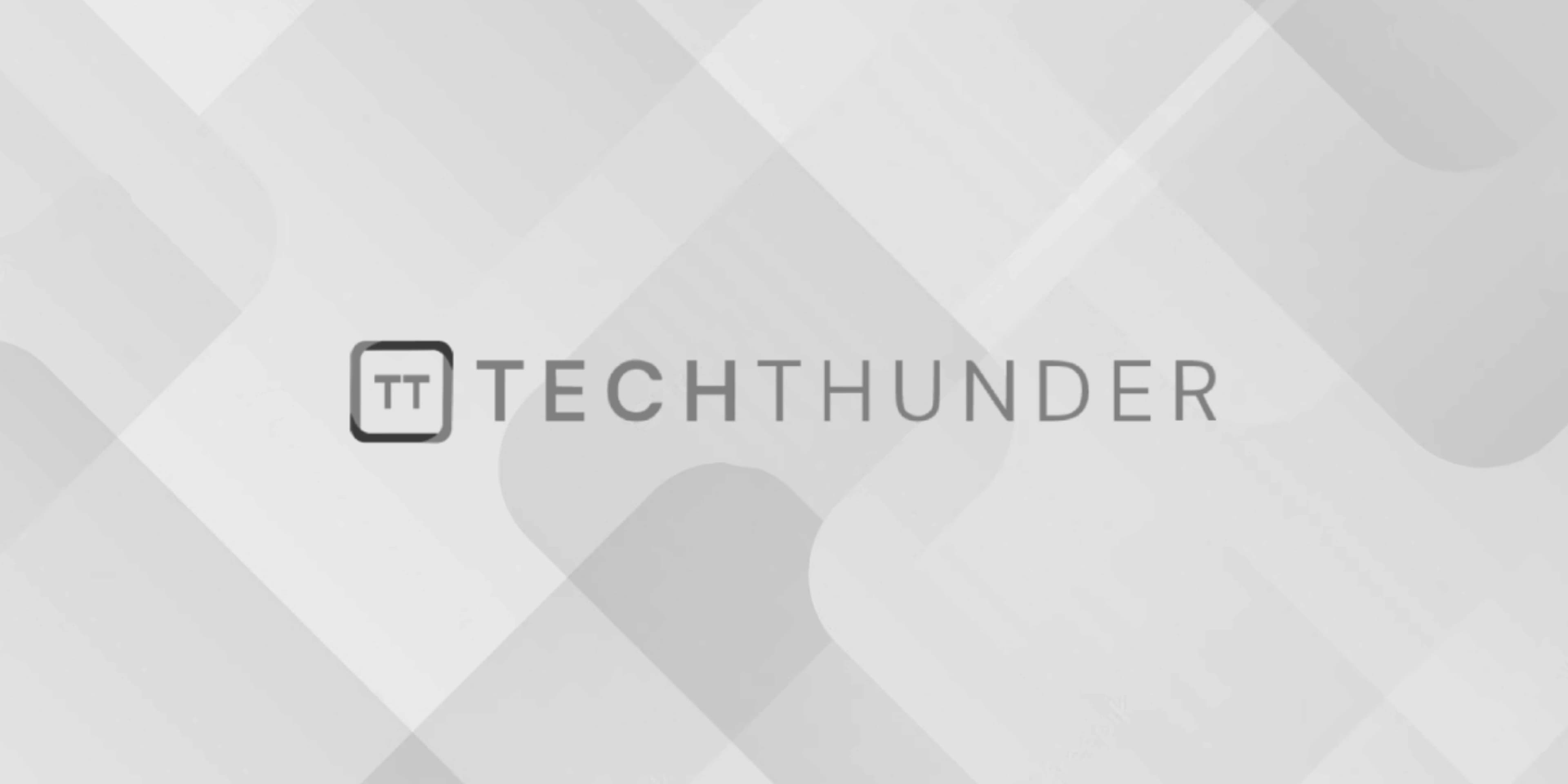
197 views
How to Change Plot Size in Matplotlib in Python
You can change the size of a plot in Matplotlib by setting the figure size before creating the plot. The figure size is specified in inches, and you can adjust both the width and height to control the overall size of the plot.
Here’s how to change the plot size in Matplotlib:
import matplotlib.pyplot as plt
# Set the figure size (width, height) in inches
plt.figure(figsize=(8, 6)) # Adjust the values as needed
# Create a simple plot
x = [1, 2, 3, 4, 5]
y = [10, 15, 13, 17, 8]
plt.plot(x, y)
# Add labels and title (optional)
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.title("Sample Plot")
# Show the plot
plt.show()
In this example:
- We import the
matplotlib.pyplot
module, commonly used for creating plots in Matplotlib. - We use
plt.figure(figsize=(8, 6))
to set the figure size. You can adjust thefigsize
parameter to your preferred width and height values in inches. - We create a simple plot using
plt.plot()
. - Optionally, we add labels to the axes and a title using
plt.xlabel()
,plt.ylabel()
, andplt.title()
. - Finally, we display the plot using
plt.show()
.
By adjusting the figsize
parameter, you can control the size of the plot to fit your requirements. The values specified in figsize
correspond to the width and height of the figure in inches.