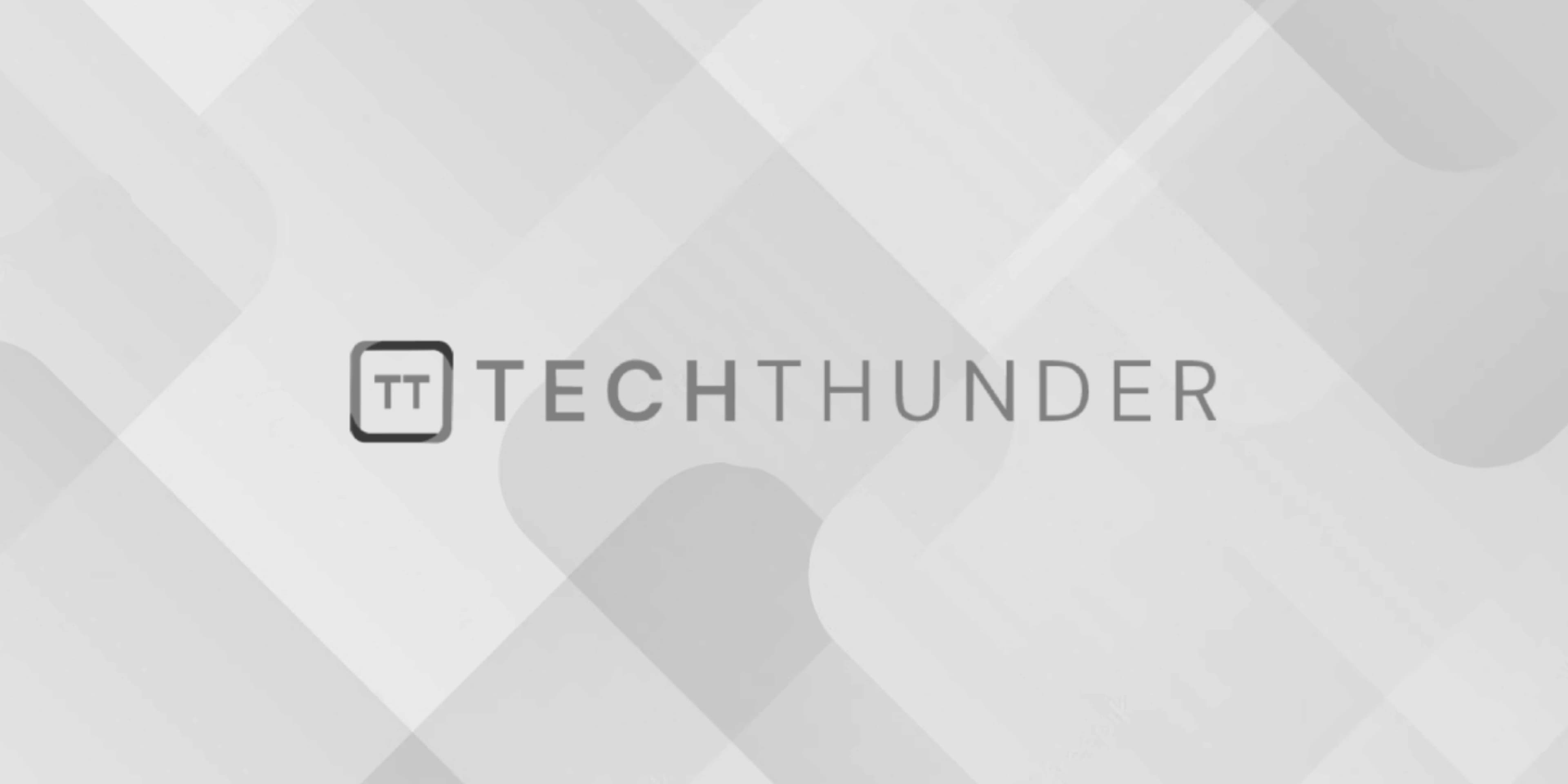
How to create a dictionary in Python
The Python, you can create a dictionary using curly braces {}
and specifying key-value pairs separated by colons :
. Here’s the basic syntax for creating a dictionary:
my_dict = {
"key1": "value1",
"key2": "value2",
"key3": "value3"
}
In this example, my_dict
is a dictionary containing three key-value pairs. The keys ("key1"
, "key2"
, "key3"
) are strings, and the values ("value1"
, "value2"
, "value3"
) can be of any data type (strings, numbers, lists, other dictionaries, etc.).
Here are a few more examples of creating dictionaries with different data types as values:
# Dictionary with integer values
person = {
"name": "Alice",
"age": 30,
"city": "New York"
}
# Dictionary with lists as values
fruits = {
"fruits_list": ["apple", "banana", "cherry"],
"colors_list": ["red", "yellow", "red"]
}
# Dictionary with another dictionary as a value
student = {
"name": "Bob",
"grades": {
"math": 95,
"science": 88,
"history": 75
}
}
You can also create an empty dictionary and add key-value pairs to it later using assignment:
empty_dict = {}
empty_dict["key1"] = "value1"
empty_dict["key2"] = "value2"
Or you can use the dict()
constructor to create a dictionary:
my_dict = dict(key1="value1", key2="value2", key3="value3")
The dict()
constructor allows you to create a dictionary with keyword arguments, where the keys are the argument names and the values are the argument values.
Dictionaries are flexible data structures that allow you to store and access data using key-value pairs, making them a powerful tool for various programming tasks.