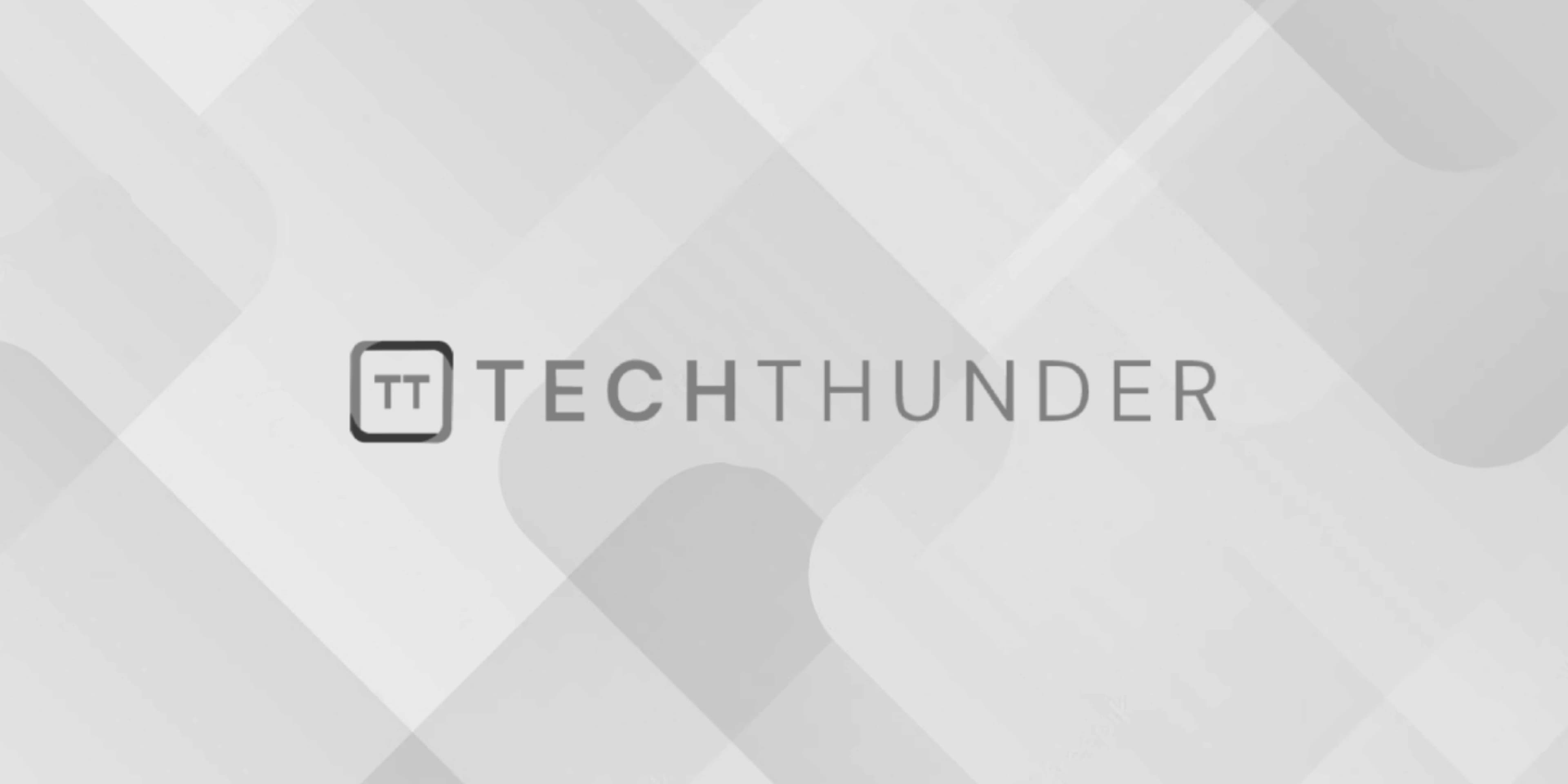
Shallow Copy and Deep Copy in Python
The Python, when you create a copy of an object, you can create either a shallow copy or a deep copy, depending on whether you want to create a new object that is a separate entity from the original or a new object that is a clone of the original with all nested objects also cloned. Understanding the difference between shallow copy and deep copy is important for managing mutable objects and avoiding unintended side effects in your code.
Here’s an explanation of shallow copy and deep copy:
Shallow Copy:
- A shallow copy of an object is a new object that is a copy of the original object with a new reference, but it doesn’t create copies of nested objects within the original object. Instead, it copies references to those nested objects.
- Shallow copies are created using various techniques, such as the
copy.copy()
function or the[:]
slice notation for lists and other iterable objects. - Changes made to the top-level attributes or elements of the copied object do not affect the original object. However, changes made to the nested objects (if any) inside the copied object will affect both the copied object and the original object, as they share references to the same nested objects.
Here’s an example of creating a shallow copy:
import copy
original_list = [1, [2, 3], 4]
shallow_copy = copy.copy(original_list)
shallow_copy[1][0] = 99 # Modifying a nested object in the shallow copy
print(original_list) # Output: [1, [99, 3], 4]
Deep Copy:
- A deep copy of an object is a new object that is a complete clone of the original object, along with all nested objects, recursively. It creates entirely separate copies of all objects within the original object.
- Deep copies are created using the
copy.deepcopy()
function from thecopy
module. - Changes made to the deep copy or any of its nested objects do not affect the original object or its nested objects because they are completely independent.
Here’s an example of creating a deep copy:
import copy
original_list = [1, [2, 3], 4]
deep_copy = copy.deepcopy(original_list)
deep_copy[1][0] = 99 # Modifying a nested object in the deep copy
print(original_list) # Output: [1, [2, 3], 4]
In summary, when working with mutable objects in Python, consider whether you need a shallow copy or a deep copy, depending on your requirements. Shallow copies are faster and more memory-efficient but may lead to unexpected behavior when nested objects are shared. Deep copies provide complete isolation between objects but may be slower and consume more memory, especially for complex data structures. Use the appropriate copying technique based on the desired behavior and data structure complexity in your specific use case.