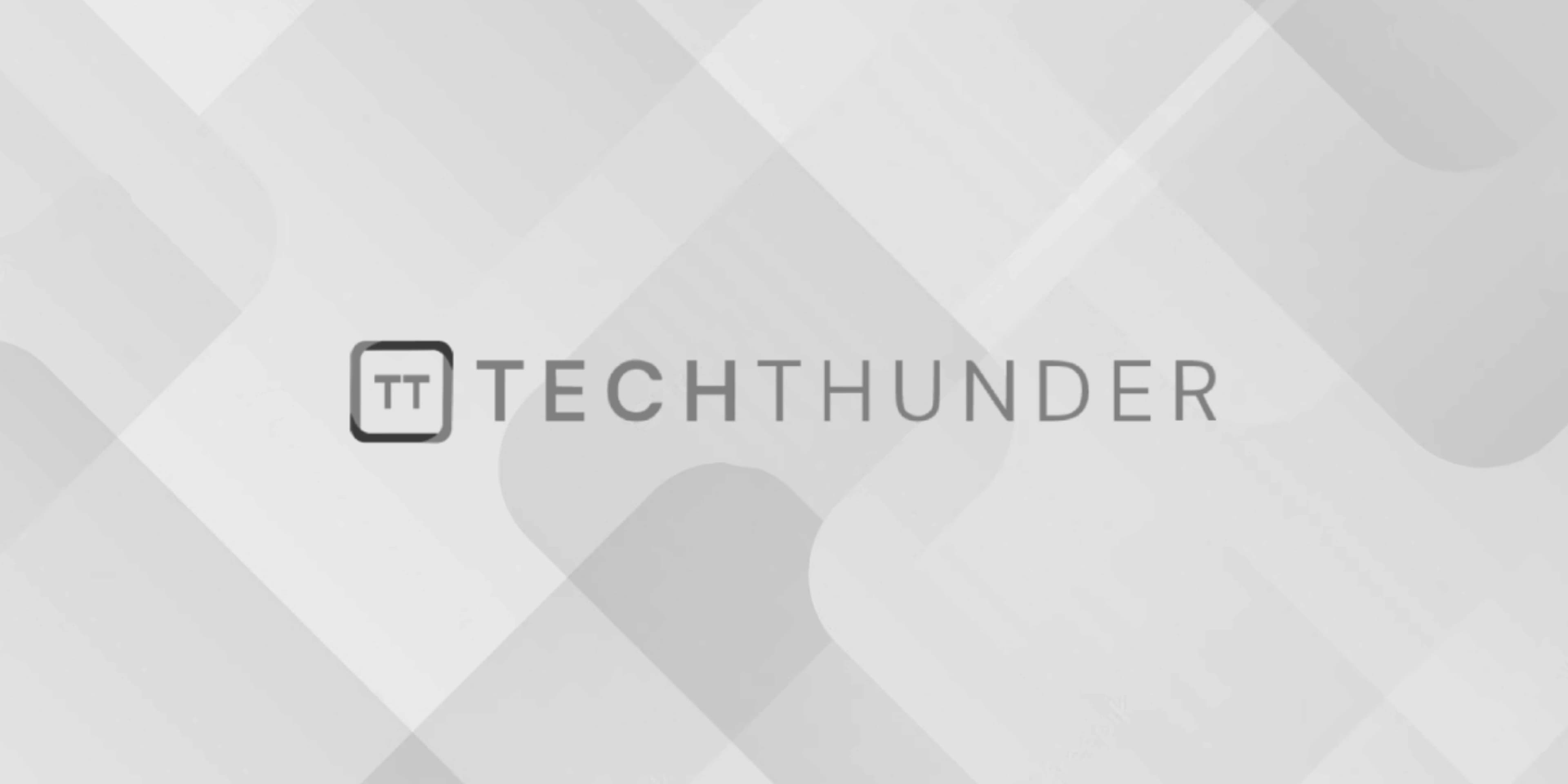
136 views
Flappy Bird Game using PyGame in Python
Creating a Flappy Bird game using Pygame in Python involves setting up a game window, handling user input, creating the game loop, and managing game objects like the bird, pipes, and background. Below is a simple example to get you started:
Python
import pygame
import sys
import random
# Initialize Pygame
pygame.init()
# Constants
WIDTH, HEIGHT = 800, 600
FPS = 60
GRAVITY = 0.5
BIRD_JUMP = 10
PIPE_SPEED = 5
PIPE_GAP = 200
PIPE_HEIGHT = 300
# Colors
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
# Create the game window
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Flappy Bird")
# Load images
bird_image = pygame.image.load("bird.png")
pipe_image = pygame.image.load("pipe.png")
background_image = pygame.image.load("background.jpg")
# Resize images
bird_image = pygame.transform.scale(bird_image, (50, 50))
pipe_image = pygame.transform.scale(pipe_image, (100, 300))
background_image = pygame.transform.scale(background_image, (WIDTH, HEIGHT))
# Bird class
class Bird(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = bird_image
self.rect = self.image.get_rect()
self.rect.center = (WIDTH // 4, HEIGHT // 2)
self.velocity = 0
def jump(self):
self.velocity = -BIRD_JUMP
def update(self):
self.velocity += GRAVITY
self.rect.y += self.velocity
# Pipe class
class Pipe(pygame.sprite.Sprite):
def __init__(self, x):
super().__init__()
self.image = pipe_image
self.rect = self.image.get_rect()
self.rect.topleft = (x, random.randint(HEIGHT // 4, HEIGHT // 2 + PIPE_GAP))
def update(self):
self.rect.x -= PIPE_SPEED
if self.rect.right < 0:
self.kill()
# Create sprite groups
all_sprites = pygame.sprite.Group()
pipes = pygame.sprite.Group()
# Create the bird
bird = Bird()
all_sprites.add(bird)
# Game loop
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bird.jump()
# Spawn pipes
if random.randint(1, 100) == 1:
pipe = Pipe(WIDTH)
all_sprites.add(pipe)
pipes.add(pipe)
# Update sprites
all_sprites.update()
# Check for collisions
hits = pygame.sprite.spritecollide(bird, pipes, False)
if hits:
pygame.quit()
sys.exit()
# Draw background
screen.blit(background_image, (0, 0))
# Draw sprites
all_sprites.draw(screen)
# Update display
pygame.display.flip()
# Cap the frame rate
clock.tick(FPS)
This example, you need to have images for the bird, pipe, and background. The bird image should be named “bird.png,” the pipe image should be named “pipe.png,” and the background image should be named “background.jpg.” Adjust the file names and paths accordingly.
Make sure you have Pygame installed before running the script:
Bash
pip install pygame
Copy and paste this code into a Python file, and run it to play a simple version of the Flappy Bird game using Pygame.